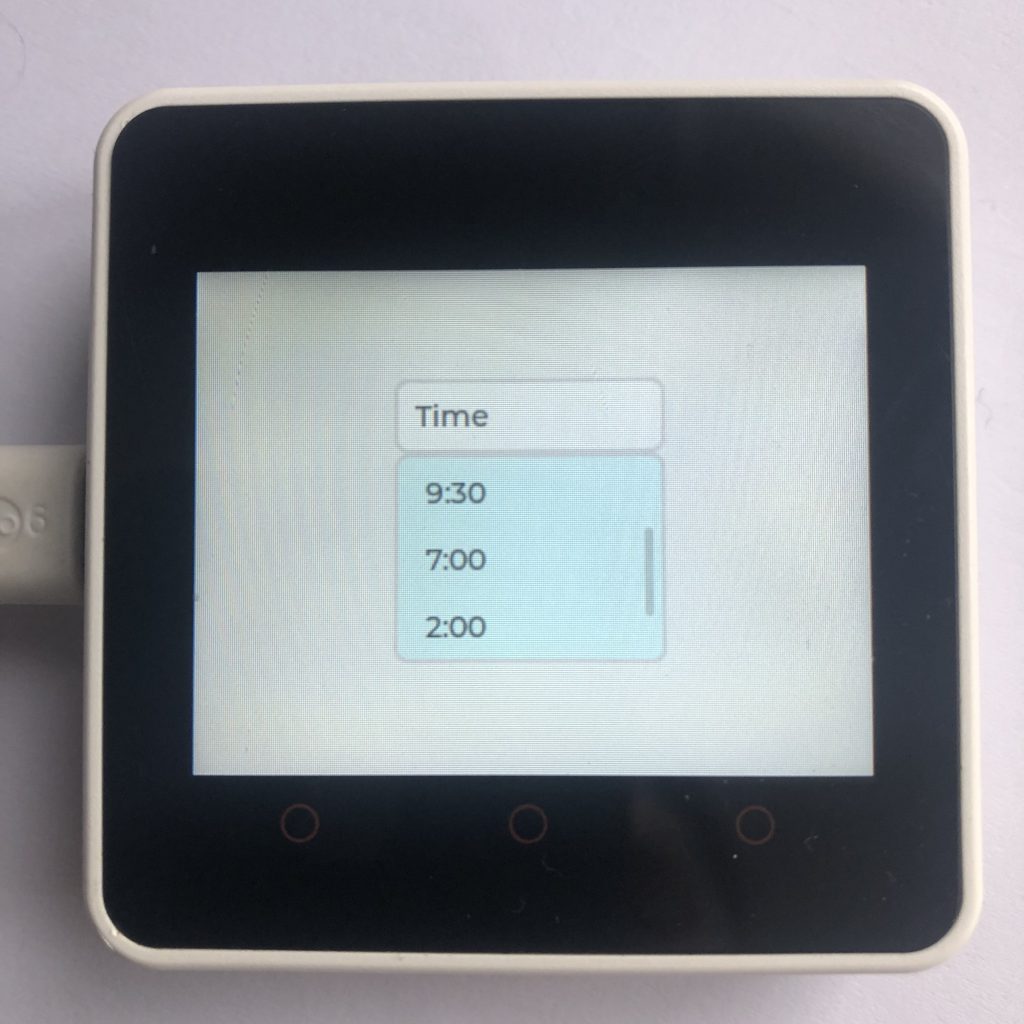
When creating a GUI, there is never enough room on the screen. You want to add this list here or that button there and there is no room left. Dropdown boxes are the age old trick to free up some space.
Dropdown boxes free space by shrinking lists, which usually take up a lot of your screen, into a small button. If you tap the button the list appears, then you can select the option you want and the list disappears again.
Today I will show you how to create, modify, and style dropdown boxes.
Setting Up a Micropython Screen
Obviously, if you are creating a program that draws things on a screen, you have to have access to a screen.
Because there are so many different screens out there, I can’t add support for all of them, so to make this example work, you will need to add your screen’s setup code to the beginning of the example.
There is a little more information on this process at How to get a LVGL Micropython Screen to Work if you aren’t familiar on how it’s done.
Here is the Example
import lvgl
drop = lvgl.dropdown(lvgl.scr_act())
drop.align(lvgl.ALIGN.CENTER,0,-50)
drop.set_dir(lvgl.DIR.BOTTOM)
drop.set_symbol('')
drop.set_selected_highlight(False)
drop.set_text('Time')
start = ['12:00','3:30','9:30','7:00','2:00','5:00']
opt = '\n'.join(start)
drop.set_options(opt)
def print_select(data):
dr = data.get_target()
ID = dr.get_selected()
name = start[ID]
print(name)
drop.add_event_cb(print_select,lvgl.EVENT.VALUE_CHANGED,None)
ls = drop.get_list()
ls.set_style_bg_color(lvgl.color_hex(0xDDFFFF),0)
ls.set_style_max_height(100,0)
Understanding This Code
Just looking at a block of code is not always very informational. To make how this micropython code works more clear, lets walk through the lines of code above and see what they do.
The Setup
First we need to import LVGL this is also a good spot to put your screen setup code.
import lvgl
The Dropdown
To begin, we create a new dropdown box.
drop = lvgl.dropdown(lvgl.scr_act())
Then we align it to 50 pixels above the center of the screen.
drop.align(lvgl.ALIGN.CENTER,0,-50)
Next we select which direction we want the list to pop out of.
drop.set_dir(lvgl.DIR.BOTTOM)
Dropdown boxes usually have a symbol next to their text. For this example, we don’t want that, so we set the symbol to ” (aka. blank text).
drop.set_symbol('')
Dropdown boxes can also highlight the list item that was last clicked. We will disable that too.
drop.set_selected_highlight(False)
After that we will set the default text of our dropdown box.
drop.set_text('Time')
Setting the Options
The whole point of the dropdown box is that it gives us a list of options and we select one. Obliviously before it can do that, it has to have a list of options, so it’s finally time to create our list of options.
To start we create a list of texts.
start = ['12:00','3:30','9:30','7:00','2:00','5:00']
Then we format the list correctly. The code below turns a list like [ ’12:00′,’3:30′,’9:30′] into the string ’12:00\n3:30\n9:30′.
opt = '\n'.join(start)
Lastly, we add the correctly formatted list of options to the dropdown box.
drop.set_options(opt)
Finding the Clicked Option
The next major thing we need to do is find the clicked option. We do that by using LVGL events.
Firstly, we need to create a new function.
def print_select(data):
Inside it we run some code that finds the object (aka. our dropdown box) that called this function.
dr = data.get_target()
Then we use that object to find the currently selected option.
ID = dr.get_selected()
After that we use the list options that we originally created to figure out the text of the selected option.
name = start[ID]
Finally we print that text to the terminal.
print(name)
Hooking Up That Function
We just created our event handler function but now we need to connect it to the dropdown box for it to work.
drop.add_event_cb(print_select,lvgl.EVENT.VALUE_CHANGED,None)
Styling the List
The last thing we want to do is style the list that appears when the dropdown is clicked.
First, we collect the list object.
ls = drop.get_list()
Then we will change the background.
ls.set_style_bg_color(lvgl.color_hex(0xDDFFFF),0)
And finally, we set the maximum size of our list.
ls.set_style_max_height(100,0)
What Should Happen
Once you run this program you should see a button that says Time. If you click it, a list will appear with different times on it and if you click one of those times, the list will disappear and the time you clicked will appear in your REPL window.
Dropdowns are useful when you don’t have lots of screen space or you are just trying to make your GUI interesting.
If you have a little more space on your screen, then LVGL rollers are a great alternative to dropdowns. Rollers make really cool GUIs but dropdowns still win when it comes to simplicity and ease of use.