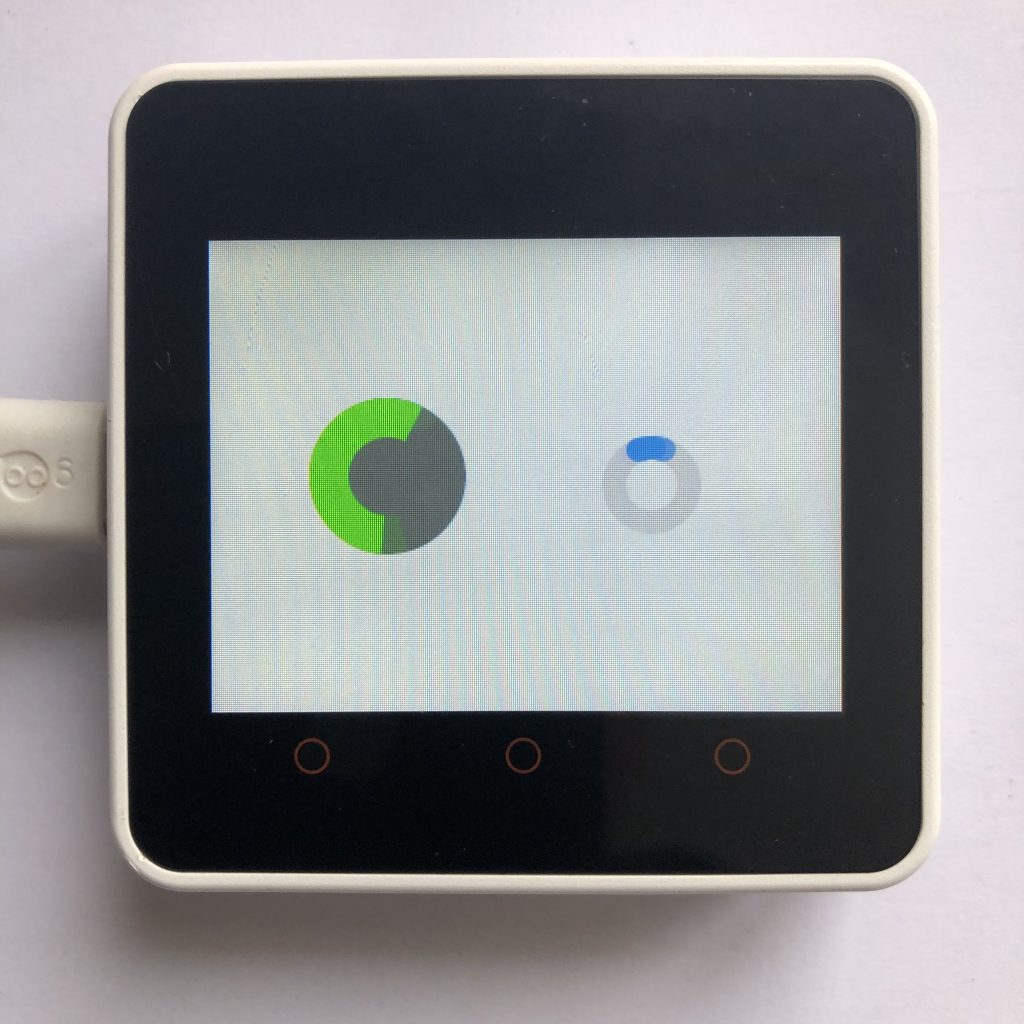
Every now and then you will create a program that takes a while to complete. When that happens, it is best if you give the user some indicator of what is happening like a progress bar. One of the most common, indicators is the spinner.
Today, we will look at how you make and edit spinners. Below is some example code and below that is an explanation of how the code works
Setting Up a Micropython Screen
Obviously, if you are creating a program that draws things on a screen, you have to have access to a screen.
Because there are so many different screens out there, I can’t add support for all of them, so to make this example work, you will need to add your screen’s setup code to the beginning of the example.
There is a little more information on this process at How to get a LVGL Micropython Screen to Work if you aren’t familiar on how it’s done.
Here is the code
import lvgl
spin = lvgl.spinner(lvgl.scr_act(),900,60)
spin.set_pos(200,100)
spin.set_size(50,50)
background = lvgl.style_t()
background.init()
background.set_arc_color(lvgl.color_hex(0x555555))
background.set_arc_width(40)
indicator = lvgl.style_t()
indicator.init()
indicator.set_arc_color(lvgl.color_hex(0x00FF00))
indicator.set_arc_rounded(0)
indicator.set_arc_width(20)
wait = lvgl.spinner(lvgl.scr_act(),1000,200)
wait.set_size(80,80)
wait.align(lvgl.ALIGN.CENTER,-70,0)
wait.add_style(background,0)
wait.add_style(indicator,lvgl.PART.INDICATOR)
Understanding This LVGL Code
Ok, now that you have seen all the code, let’s step through each section, so you can understand exactly what each part does. This will make it much easier to actually use spinners in your projects…
The Setup
First we need to import the LVGL module. This is also a good spot to put you screen setup code.
import lvgl
A Basic Spinner
Because spinners are usually used when you microcontroller is doing some hard task its best to make them as simple as possible so they don’t slow down your microcontroller.
We will start by creating a new spinner. “lvgl.scr_act()” tells the computer to put the spinner on the main screen.
The first numbers is how many milliseconds it takes for the spinner to make one rotation, and the last number controls how big the blue part of the spinner is.
spin = lvgl.spinner(lvgl.scr_act(),900,60)
Then we will set the position of the spinner.
spin.set_pos(200,100)
And finally we set the size of the spinner.
spin.set_size(50,50)
This is all it takes to make a simple spinner.
Creating Some Styles
We are going to create one more spinner, and this one will have some styles added to it.
first we create the style for the background.
background = lvgl.style_t()
Then, we initialize our new style.
background.init()
After that, we are going to set the background color to a dark grey.
background.set_arc_color(lvgl.color_hex(0x555555))
Then, we will set the width of the arc.
background.set_arc_width(40)
Next, we make the style for the indicator.
indicator = lvgl.style_t()
Then, we initialize it.
indicator.init()
After that, we set the it’s color to a bright green.
indicator.set_arc_color(lvgl.color_hex(0x00FF00))
We are also going to make the edges of the indicator flat, normally they would be rounded.
indicator.set_arc_rounded(0)
And lastly, we will set the indicators width to 20 pixels.
indicator.set_arc_width(20)
The Last Spinner
We will start by creating a new spinner.
wait = lvgl.spinner(lvgl.scr_act(),1000,200)
Then we will set it’s size to 80*80 pixels.
wait.set_size(80,80)
We will align it to the center and then have it move 70 pixels to the left.
wait.align(lvgl.ALIGN.CENTER,-70,0)
The last thing we need to do is add the styles we created. First we add the background style like normal.
wait.add_style(background,0)
Then we add the indicator style to the indicator part.
wait.add_style(indicator,lvgl.PART.INDICATOR)
What Should Happen?
Once you run the program, the two spinners we created should spin. The big spinner should rotate slightly slower the the small one. For most projects, I would use the smaller spinner because it is simpler.
Once you task is done you should call .delete() on your spinner for example you would delete the to spinners we just created like this:
spin.delete()
wait.delete()
If you want a indicator that gives a little more information to the user, I would suggest looking into LVGL Bars.