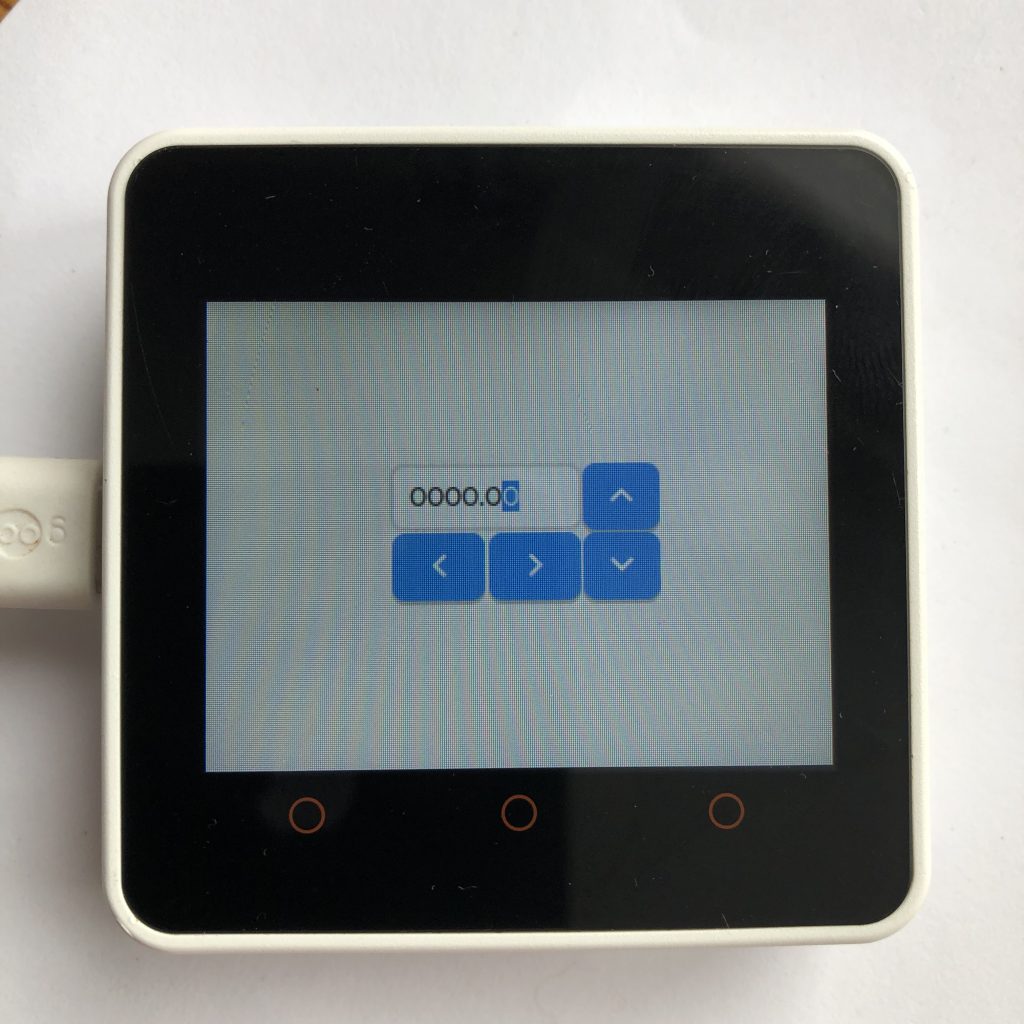
Many GUIs need a way to input a number. Normally this is done with a Keypad and a Textarea but that takes a lot of screen space. Spinboxes can do the same job but they are much smaller.
Lets look at how you can create a spinbox and use some buttons to control it.
Setting Up a Micropython Screen
Because there are so many different screens out there, I can’t add support for all of them to the example, so to make the example work, you will need to add your screen’s setup code to the beginning of it.
There is a little more information on this process at How to get a LVGL Micropython Screen to Work if you aren’t familiar on how it’s done.
Here is the code
import lvgl
spin = lvgl.spinbox(lvgl.scr_act())
spin.align(lvgl.ALIGN.CENTER,-17,-19)
spin.set_size(100,35)
spin.set_range(0,100000)
spin.set_digit_format(6,4)
up = lvgl.btn(lvgl.scr_act())
up.set_size(40,35)
up.align(lvgl.ALIGN.CENTER,53,-19)
down = lvgl.btn(lvgl.scr_act())
down.set_size(40,35)
down.align(lvgl.ALIGN.CENTER,53,17)
left = lvgl.btn(lvgl.scr_act())
left.set_size(48,35)
left.align(lvgl.ALIGN.CENTER,-42,17)
right = lvgl.btn(lvgl.scr_act())
right.set_size(48,35)
right.align(lvgl.ALIGN.CENTER,8,17)
def add_lb(text,obj):
lb = lvgl.label(obj)
lb.set_text(text)
lb.center()
add_lb(lvgl.SYMBOL.UP,up)
add_lb(lvgl.SYMBOL.DOWN,down)
add_lb(lvgl.SYMBOL.LEFT,left)
add_lb(lvgl.SYMBOL.RIGHT,right)
def up_handler(data):
spin.increment()
up.add_event_cb(up_handler,lvgl.EVENT.CLICKED,None)
def down_handler(data):
spin.decrement()
down.add_event_cb(down_handler,lvgl.EVENT.CLICKED,None)
position = 0
def left_handler(data):
global position
if position == 0:
position = 1
position = min(position*10,100000)
spin.set_step(position)
left.add_event_cb(left_handler,lvgl.EVENT.CLICKED,None)
def right_handler(data):
global position
position = int(max(position/10,0))
spin.set_step(position)
right.add_event_cb(right_handler,lvgl.EVENT.CLICKED,None)
How Does This Code Work?
Since you’ve seen the program above, why don’t we walk through the code and see how it works. This should make it clearer how you can use spinners in your projects.
The Setup
First we import the LVGL module. This is also a good spot to put your screen setup code.
import lvgl
Creating the Spinner
To begin, we create a new spinner.
spin = lvgl.spinbox(lvgl.scr_act())
Then, we will position it on the screen.
spin.align(lvgl.ALIGN.CENTER,-17,-19)
Next, we set is size to 100*35 pixels.
spin.set_size(100,35)
After that, we will set the range of the spinbox to 0-100,000. The range limits the number inside the spinbox so for us that number can never be bigger that 100,000 or smaller than 0.
spin.set_range(0,100000)
Finally we will format the spinbox. When you format a spinbox you give it two numbers: the first tells LVGL how many digits the spinbox should have, and the second number tells LVGL where to put the decimal point.
spin.set_digit_format(6,4)
The Buttons
To use the spin box we need a few buttons. We will start by creating the up button.
up = lvgl.btn(lvgl.scr_act())
Then we will set its size.
up.set_size(40,35)
Lastly we set its position on the screen.
up.align(lvgl.ALIGN.CENTER,53,-19)
We create the rest of the buttons the same way.
down = lvgl.btn(lvgl.scr_act())
down.set_size(40,35)
down.align(lvgl.ALIGN.CENTER,53,17)
left = lvgl.btn(lvgl.scr_act())
left.set_size(48,35)
left.align(lvgl.ALIGN.CENTER,-42,17)
right = lvgl.btn(lvgl.scr_act())
right.set_size(48,35)
right.align(lvgl.ALIGN.CENTER,8,17)
The Labels
We now have four buttons but they all look the same so we need to add a label to each of them. To make that process easier we will create a function.
def add_lb(text,obj):
This function will create a label inside a button that we choose, set its text, and center it.
lb = lvgl.label(obj)
lb.set_text(text)
lb.center()
After that we use the above function to add the UP symbol to the up button.
add_lb(lvgl.SYMBOL.UP,up)
We add the rest of the symbols like we did the first.
add_lb(lvgl.SYMBOL.DOWN,down)
add_lb(lvgl.SYMBOL.LEFT,left)
add_lb(lvgl.SYMBOL.RIGHT,right)
Connecting the Buttons
We last thing we have to do is connect the buttons we have made to the spinbox. We use handler functions to do that.
As usual we will start with the up button by create a new function.
def up_handler(data):
In side that function we simply tell the spin box to increment its value.
spin.increment()
And finally we attach that handler to the up button so whenever the button is clicked the function is called.
up.add_event_cb(up_handler,lvgl.EVENT.CLICKED,None)
The down button is the same as the up bottom except we decrement the value instead of increment.
def down_handler(data):
spin.decrement()
down.add_event_cb(down_handler,lvgl.EVENT.CLICKED,None)
The left and right buttons require a little more work we will start with the left.
To begin we create a new variable.
position = 0
Then we create the left handler function.
def left_handler(data):
Inside that function we import the position variable we created.
global position
Then we check if position equals zero if it is we set position equal to one.
if position == 0:
position = 1
Then we multiply position by 10 and constrain it so its not bigger than 100,000.
position = min(position*10,100000)
After that we set the spinbox’es cursor to the correct position.
spin.set_step(position)
To finish the left button off we add that function to the button.
left.add_event_cb(left_handler,lvgl.EVENT.CLICKED,None)
The right buttons function is very similar to the one we just made except its a little simpler.
def right_handler(data):
global position
position = int(max(position/10,0))
spin.set_step(position)
Last of all we add that function to the right button.
right.add_event_cb(right_handler,lvgl.EVENT.CLICKED,None)
What Should Happen?
Once you run the program, the spinbox and the four buttons should appear on your screen. If you click the up or down buttons the number in the spinbox that the cursor is on will get bigger or smaller. When you press the left or right button the cursor in the spinbox will move left or right.
Spinboxes are extremely customizeable. You can fit them into pretty much any little gap on your screen. The only side affect of using spinboxes is that they are a little slow to put numbers in.
In general a full on keypad will be nicer to use but if you don’t have the room spinboxes are a excellent replacement.
If you are looking for other ways to shrink down your GUI then you might like to look into LVGL dropdown boxes.