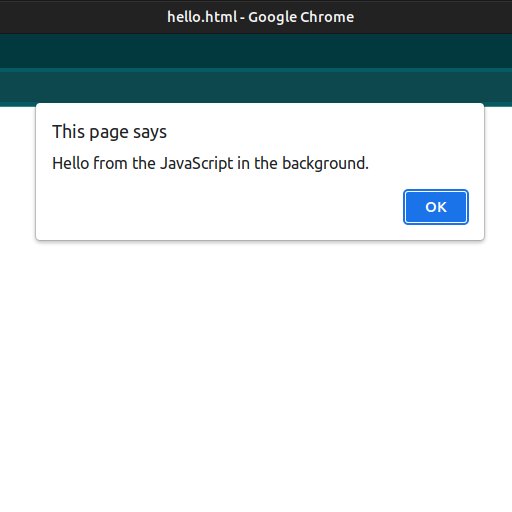
To create a web page for your ESP32, you need to know HTML, CSS, and JavaScript, and while I can’t help you much with HTML and CSS, JavaScript is a different story.
JavaScript is very similar to C++ (the Arduino IDE language) and if you already know C++ there is a faster way to learn it.
Instead of learning the entire language from scratch, you can learn the major differences between C++ and JavaScript. Which should take much less time then reading an entire beginners guide to JavaScript.
Where To Put Your Code
It is pretty well know that JavaScript is part of how web pages are made, but a little less know is how you actual put it in the page. There is two main ways and I hope you understand a little HTML other wise this will probably be complete gibberish.
The first place is in-between the HTML script tags, which looks something like this.
<script>
// Your code goes here
</script>
Or you can create a file, lets say you called it mycode.js, and then include it with the script tags.
<script src="mycode.js"></script>
If you use the file method make sure to put your js file in the same folder as your HTML code.
Void Loop and Void Setup
You should be familiar with the idea that Arduino boards have a setup and loop function and they are where you start your code from. They usually look something like this.
void setup(){
//some setup code
}
void loop(){
//some loop code
}
JavaScript does not have ether of these functions, instead you write code where ever you want. Think of it like the entire program is inside the setup function.
Here is how you would write the code above in JavaScript.
//some setup code
while(true){
//some loop code
}
Variables
Arduino has a lot of different ways you can declare a variable. You have int, String, char, byte, bool, etc. JavaScript is different in that it only has four ways you create variables.
Var
The var keyword replaces all of the Arduino variable types. Lets run through a few examples.
This is how you create a number variable in C++.
int number = 123;
And this is how its done with JS (JavaScript).
var number = 123;
Here is a letter variable in C++.
char letter = 'L';
And here is how its done with JS.
var letter = 'L';
You should see the pattern, you just replace any keyword you would normally use like int with the var keyword.
Const
The Const keyword is used to create a variable that can be read but never changed.
Here is how you use it.
const number = 123;
Here is the C++ equivalent.
const int number = 123;
Let
The let keyword is used to make your programs slightly more memory efficient.
If you used the var keyword to create a variable inside of an if statement, you can still access the variable after the if statement has finished like in the code below.
if(true){
var myvar = 10;
}
alert(myvar); // totally allowed
But many times you don’t need that variable any more, and it is just waisting your memory.
The let keyword tells the computer to automatically delete the variable once the if statement is completed.
if(true){
let myvar = 10;
}
alert(myvar); // myvar no longer exists so it is undefined
In fact the let command works for any code inside of brackets {}, so it is also useful with loops.
Nothing
This is probably the weirdest of the bunch, but you are also allowed to create variables with out a keyword at all.
myvar = 10;
Functions
Functions are pretty much identical except the parts that use variables. It is easiest, if I show an example.
Here is a C++ function.
int add(int a,int b){
return a + b;
}
And here is the same function in JS.
function add(a,b){
return a + b;
}
The key things to notice is that all functions start with the word function and that the arguments for a function are simply a list of names and never have words like var or let with them.
Comparisons
In C++ comparisons are done with the ==, !=, >=, etc. In JavaScript you need to add an extra = to the end of the == and != commands. As usual here is an example.
C++ code.
int value = 200;
if(value >= 1000){
Serial.println("High");
}else if(value == 0){
Serial.println("Zero");
}else{
Serial.println("In-between");
}
Add here is the same thing in JavaScript.
var value = 200;
if(value >= 1000){
alert("High");
}else if(value === 0){
alert("Zero");
}else{
alert("In-between");
}
Arrays
JavaScript and C++ arrays are actually very different behind the scenes. To start here is a C++ array.
int numbers[10] = {0,10,20,30,40,50,60,70,80,90};
Serial.println(numbers[3]);
And here is the same array but in JS.
var numbers = [0,10,20,30,40,50,60,70,80,90];
alert(numbers[3]);
While those two arrays may look similar, there are actually two main differences, JavaScript arrays can change in size which is why, when you create a new array, you don’t have to specify the size.
JavaScript arrays can also contain multiple types of data at the same time, look at this array.
var mixedArray = [10,"some text",true,"and some more"];
Strings
Arduino boards have two types of strings used with them C style strings, and the String library. JavaScript strings are closest to the String library.
Here is a simple example of them in action.
var string = "hello world";
string = string + "!" + 1;
alert(string);
Input and Output
When you are writing code, it is always nice, if you have some simple way to send debug information out and receive commands in.
You can get basic input and output by using the alert and prompt commands.
alert("some message");
var answer = prompt("a question");
The alert command creates a popup box with the message you give it and The prompt command will create a popup box that asks a question and below that there is a little text area that you can type your answer in. Your answer will then be returned by the prompt command.
Math
Thankfully math is pretty much the same in both languages. You can use the +, -, *, / or the +=, -=, etc. The only thing you will need to keep in mind is that JavaScript will automatically turn strings into numbers, if the strings are in a math equation.
var result = "100" - 20;
The result of the above code will be 80. This rule is true for all operations except addition.
var result = "100" + 20;
Instead a equaling 120, the code above actually results in “10020”. With addition, the number will be turned to a string, and then the two strings will be added together.
Loops
JavaScript loops are the same as C++.
for(var i = 0; i < 100;i++){
//do something
}
while(true){
//do something
}
Obliviously I can’t explain the entire JavaScript language in a single post, but by now you should have a basic understanding of how it works.