What Are LVGL Events?
Events are how lvgl controls action. If you click a button, it will call an event to tell you a button was pressed. This page has five examples on events and how they work.
Getting the Screen to Work
This is some simple code to get a ili9341 display to work with micropython lvgl. You will want to add it or your own screen code to the beginning of all the examples on this page.
Instead of putting it at the beginning you could just put the code in the micropython boot file. It seems to work best there.
import lvgl
#this is the code that works for my screen:
#from ili9XXX import ili9341
#screen = ili9341(mosi=23, miso=38, clk=18, dc=15, cs=5, invert=True rot=0x10, width=320, height=240 )
# this is some generic code that might work better for you
from ili9XXX import ili9341
screen = ili9341(mosi=23, miso=38, clk=18, dc=15, cs=5,width=320, height=240)
#You will want some touch screen code
#here is what I use
from ft6x36 import ft6x36
touch = ft6x36()
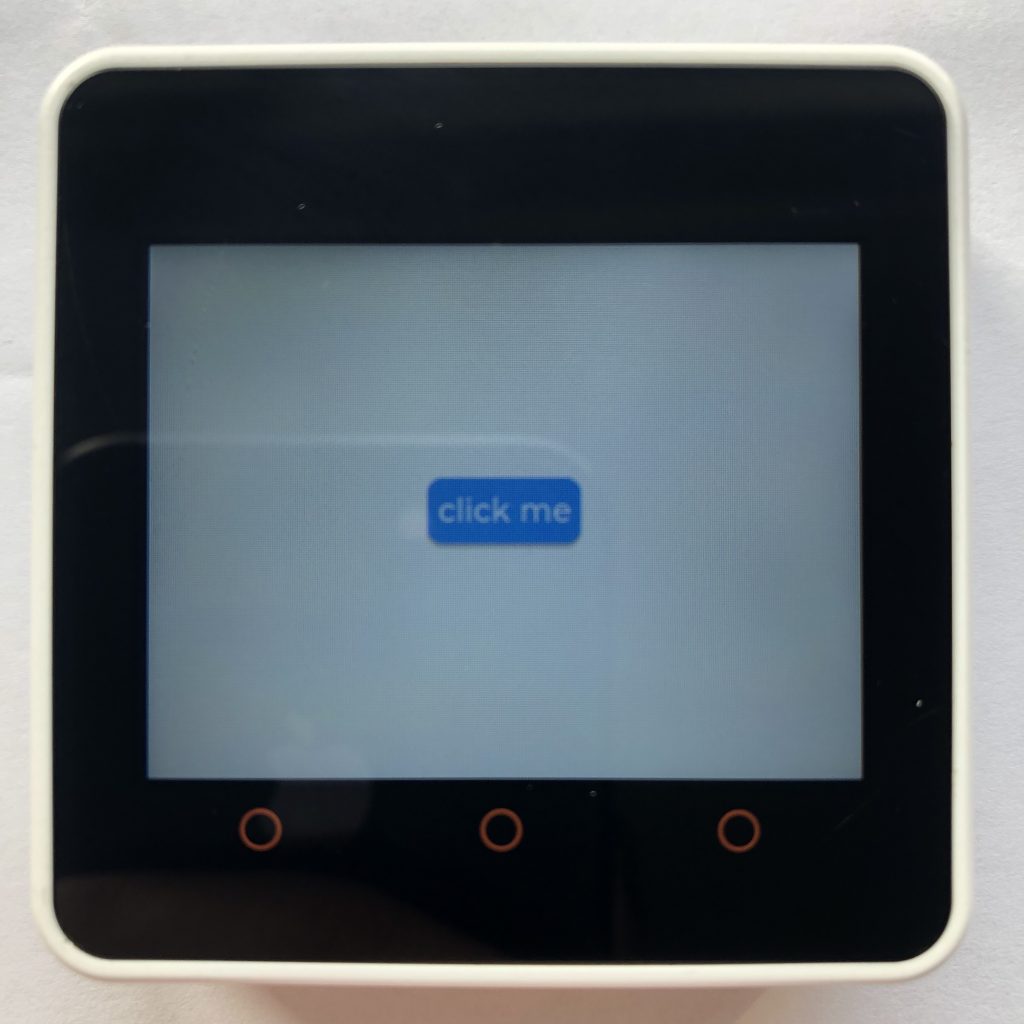
Buttons and Events
This is about as simple as it gets. If you hit the button, it prints “button clicked” on your computer.
import lvgl
button = lvgl.btn( lvgl.scr_act() )
button.set_size( 70, 30 )
button.center()
label = lvgl.label( button )
label.set_text( 'click me' )
label.center()
def handler(data):
event_type = data.get_code()
if event_type == lvgl.EVENT.CLICKED:
print( 'button clicked' )
button.add_event_cb( handler, lvgl.EVENT.ALL, None )
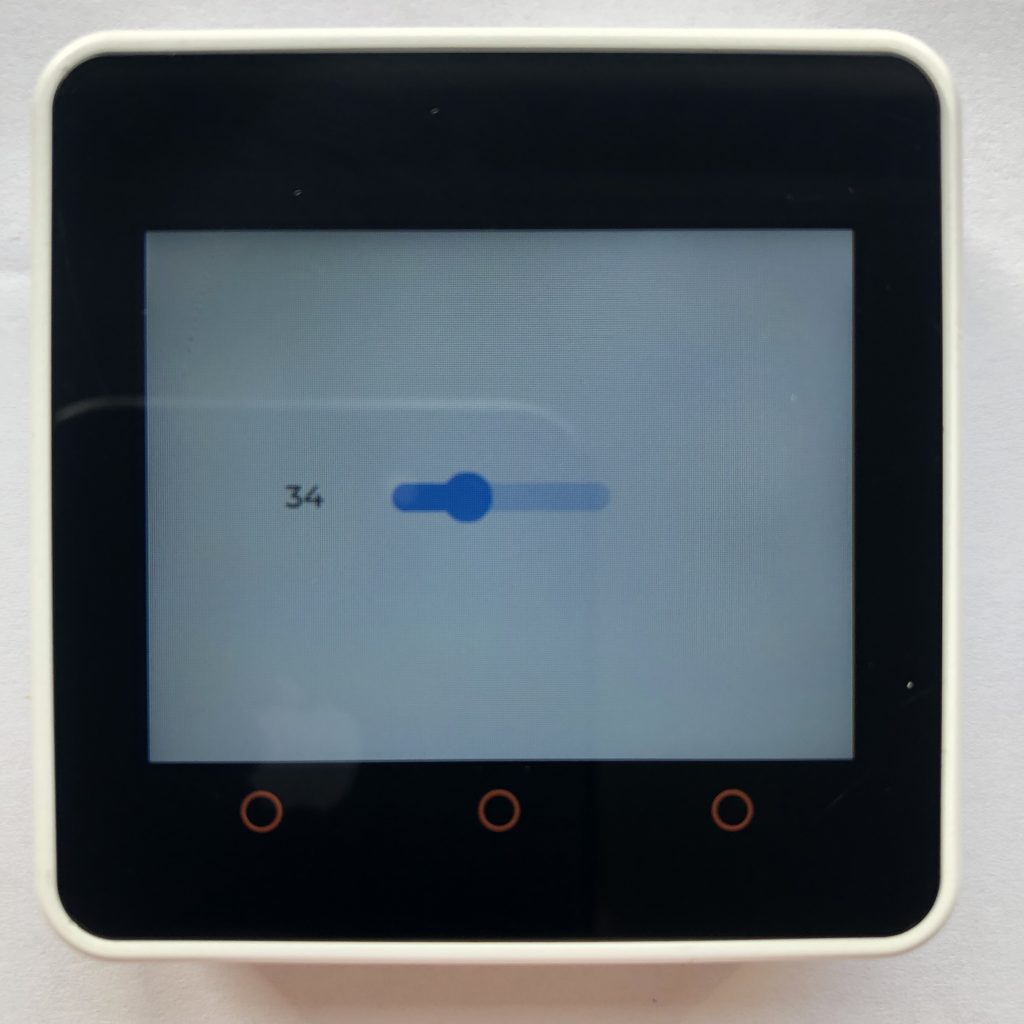
Sliders and Events
This example shows you how to use events to get the position of a slider and display it on your screen.
import lvgl
label = lvgl.label( lvgl.scr_act() )
label.set_text( '0' )
label.center()
label.set_x( -90 )
def handler( data ):
the_slider = data.get_target()
the_value = the_slider.get_value()
label.set_text( str ( the_value ) )
print(the_value)
slider = lvgl.slider( lvgl.scr_act() )
slider.center()
slider.set_width( 100 )
slider.add_event_cb( handler, lvgl.EVENT.VALUE_CHANGED, None )
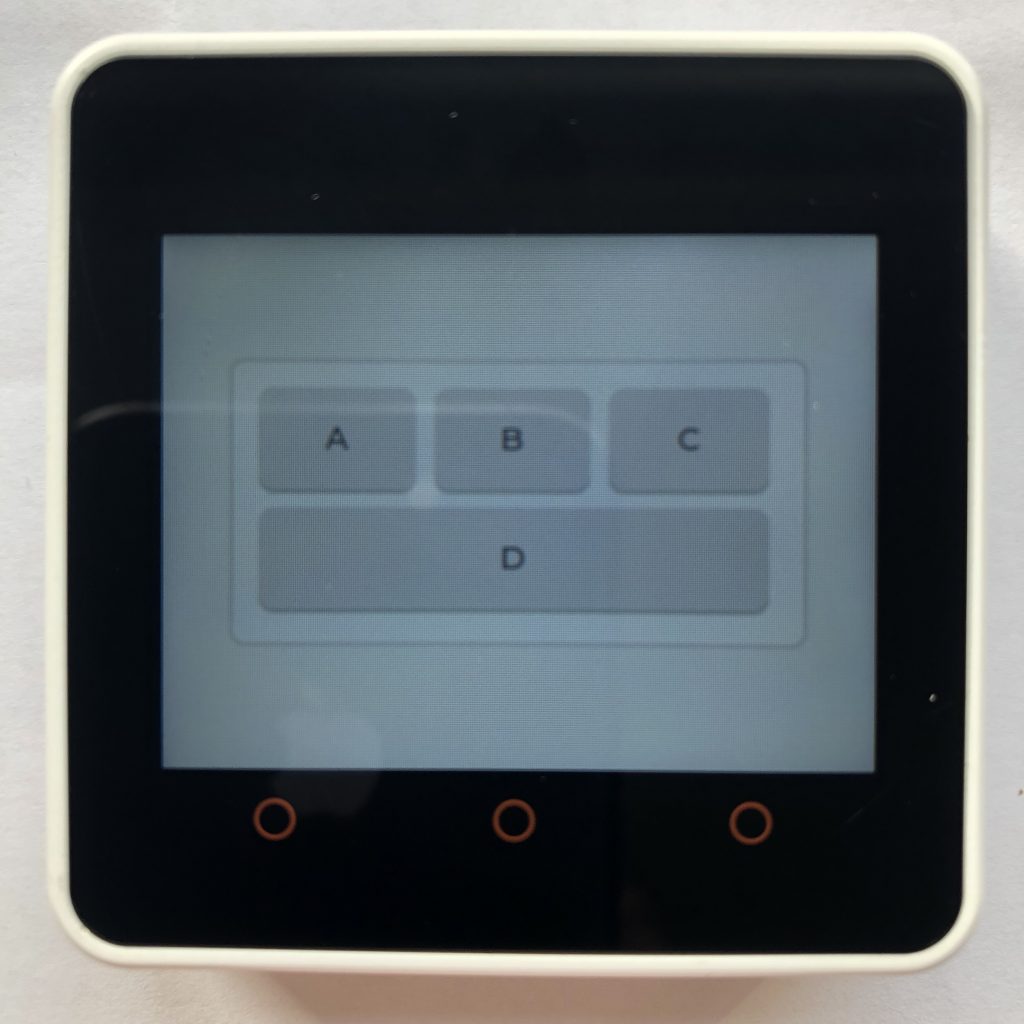
Button Matrixs and Micropython Events
Button matrixs allow you to create a lot of buttons quickly, but they are a little more complicated to work with. Here is the rough idea:
import lvgl
buttonMap = ['A','B','C','\n',
'D']
def handler(data):
target = data.get_target()
btn = target.get_selected_btn()
label = target.get_btn_text(btn)
print(label,'pressed')
matrix = lvgl.btnmatrix(lvgl.scr_act())
matrix.set_map(buttonMap)
matrix.set_btn_width(5,3)
matrix.center()
matrix.add_event_cb(handler,lvgl.EVENT.VALUE_CHANGED,None)
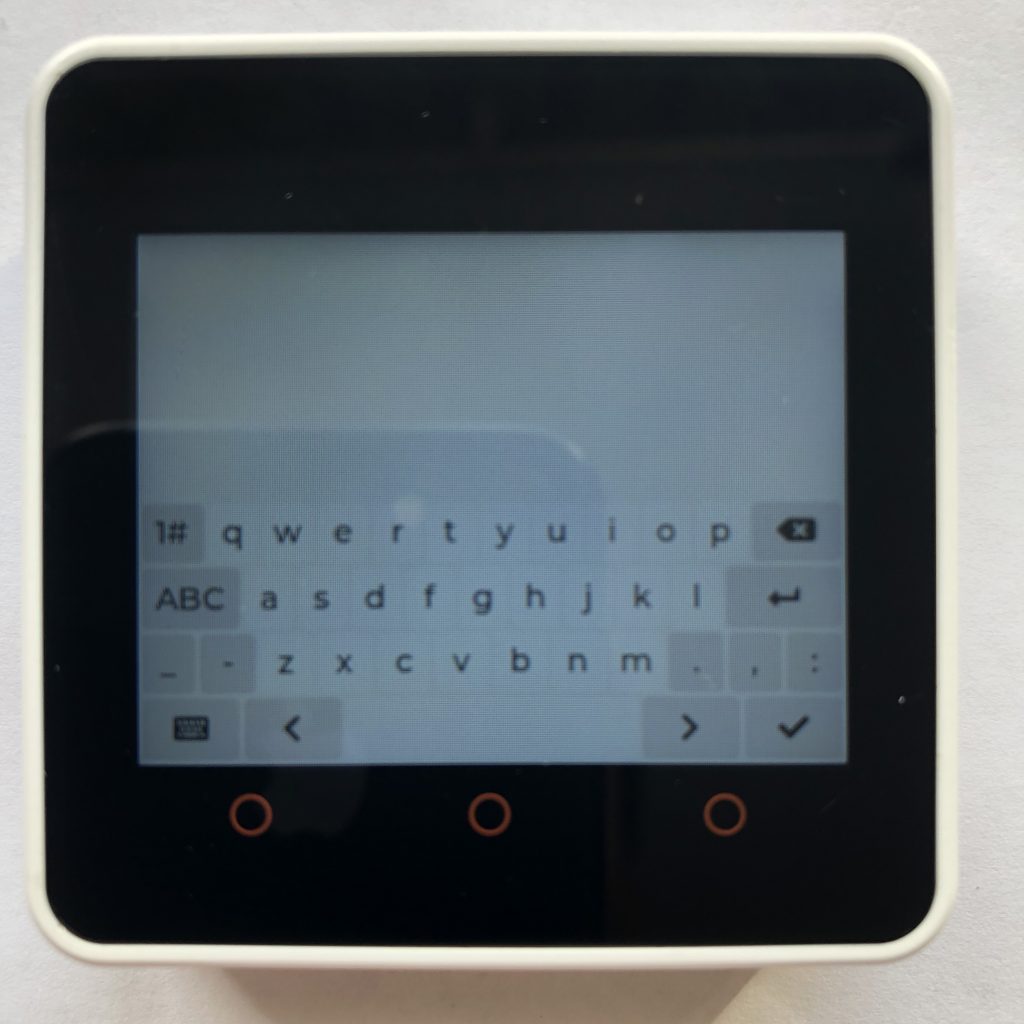
Keyboards and Events
Keyboards are a special type of button matrix. They are automatically formatted and setup with the alphabet, symbols, and numbers. You don’t have to create all those buttons.
import lvgl
def handler(data):
target = data.get_target()
btn = target.get_selected_btn()
label = target.get_btn_text(btn)
print(btn,label,'pressed')
kyb = lvgl.keyboard(lvgl.scr_act())
kyb.add_event_cb(handler,lvgl.EVENT.VALUE_CHANGED,None)
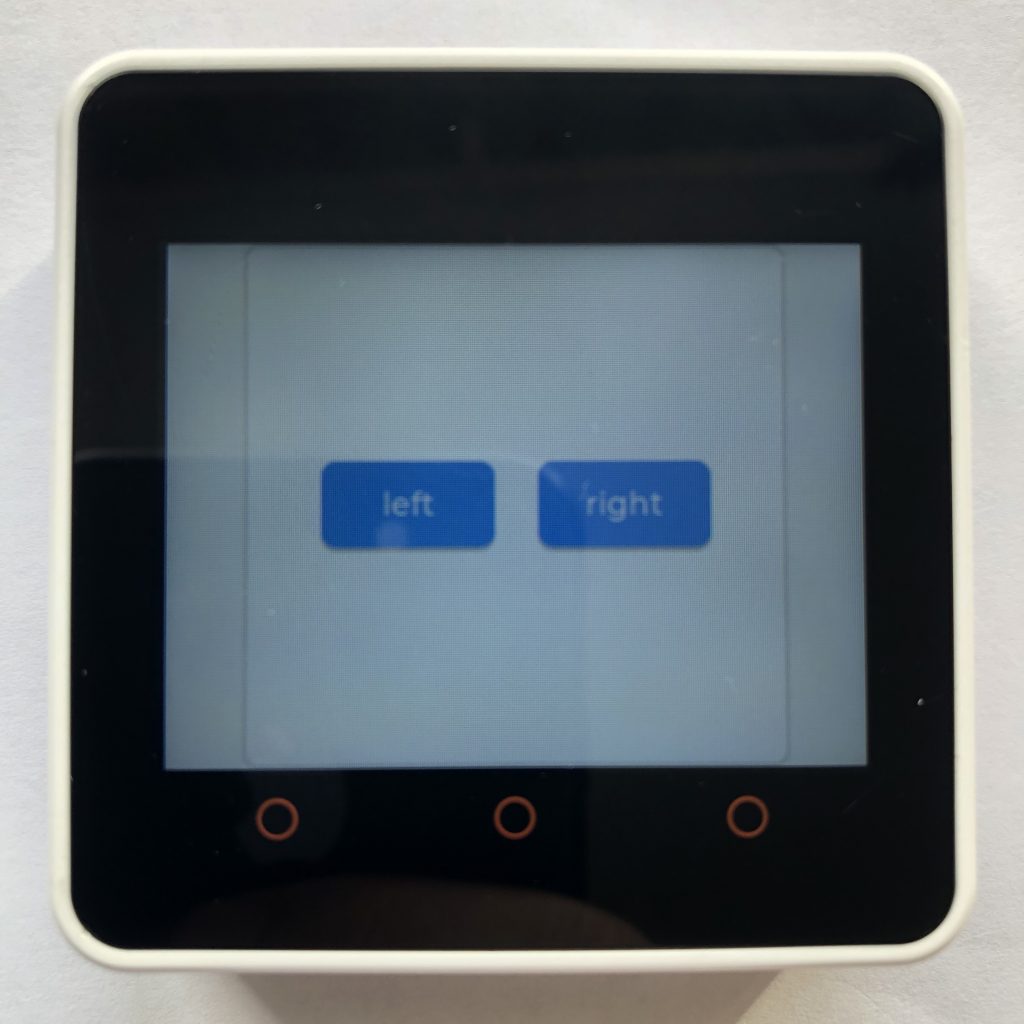
Event Bubbling
Event bubbling allows a parent object to collect the events of its children. If setup completely, you could run your entire program with only one handler function.
import lvgl
#event bubbling allows a parent object to collect all the events of its children
def handler( data ):
obj = data.get_target()
if type( obj ) == type( lvgl.btn() ):
print( 'button pressed' )
obj = lvgl.obj( lvgl.scr_act() )
obj.set_size( 250, 240 )
obj.center()
obj.add_event_cb( handler, lvgl.EVENT.CLICKED, None )
leftButton = lvgl.btn( ob j)
#each button has to enable event bubbling
leftButton.add_flag( lvgl.obj.FLAG.EVENT_BUBBLE )
leftButton.set_size( 80, 40 )
leftButton.center()
leftButton.set_pos( -50, 0 )
leftLabel = lvgl.label( leftButton )
leftLabel.center()
leftLabel.set_text( 'left' )
rightButton = lvgl.btn( obj )
rightButton.add_flag( lvgl.obj.FLAG.EVENT_BUBBLE )
rightButton.set_size( 80, 40 )
rightButton.center()
rightButton.set_pos( 50, 0 )
rightLabel = lvgl.label( rightButton )
rightLabel.center()
rightLabel.set_text( 'right' )
What You Learned
This page has show you how to work with buttons, sliders, and matrixs. Using these should give you some nice inputs for your projects.
If you like lvgl events you might also like lvgl meters.