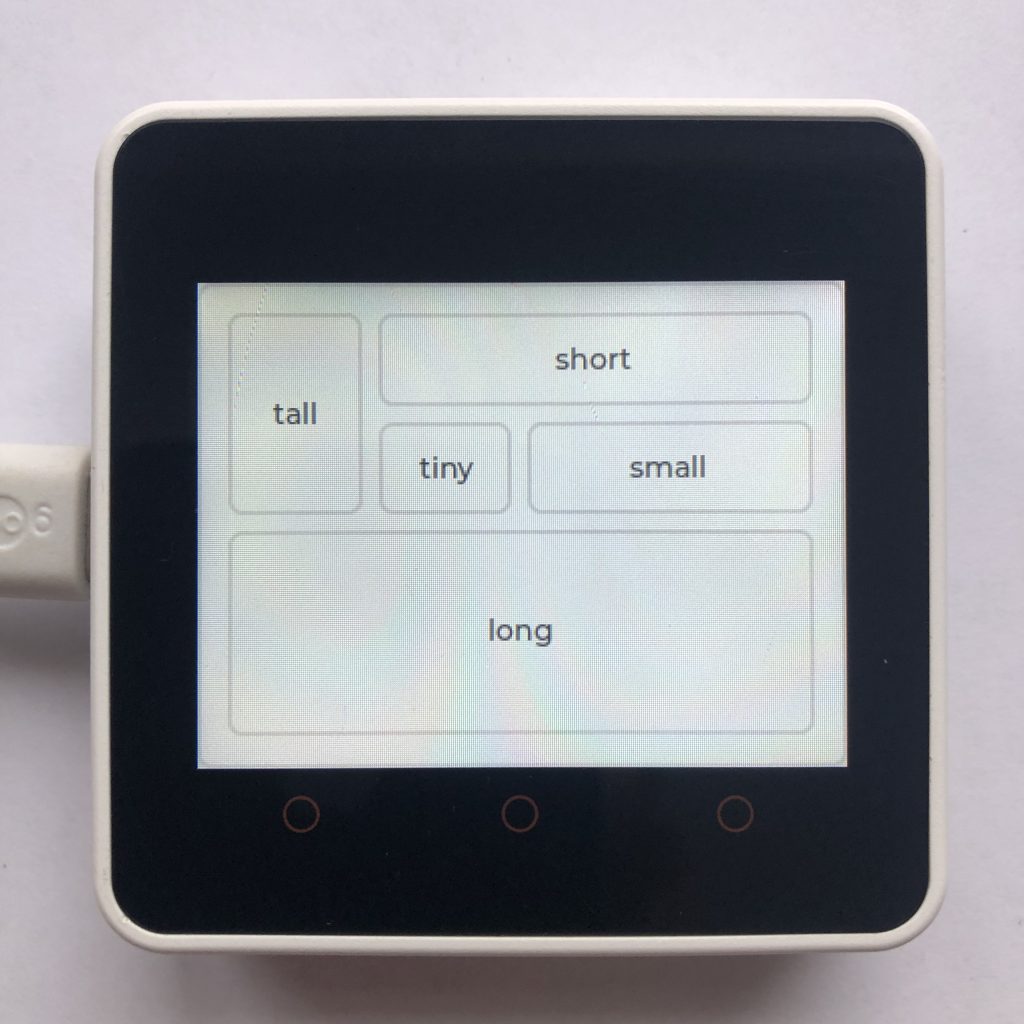
What layouts are there?
Lvgl currently supports two layouts: FLEX and GRID. The flex layout is simple, but not very controllable.
The grid layout is more complicated, but gives you more control. This page has examples for both of them.
How to use these examples
To get these examples to work you will need to add some screen code to the beginning of each example. If you want more information try How to get a LVGL Micropython screen to work.
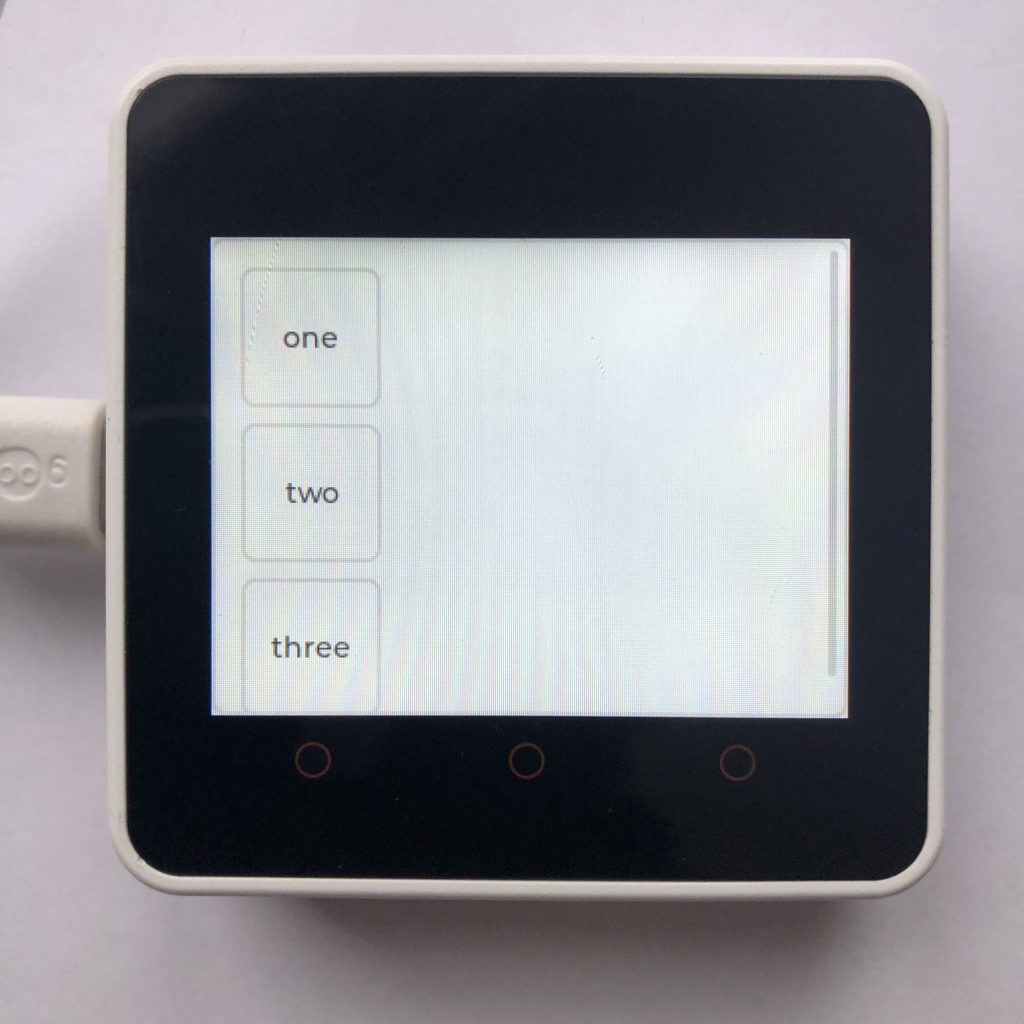
LVGL Flex Basic Example
This code creates a container with the flex layout enabled, and puts three objects in it.
import lvgl
cont = lvgl.obj( lvgl.scr_act() )
cont.set_size( 320, 240 )
cont.center()
cont.set_layout( lvgl.LAYOUT_FLEX.value )
cont.set_flex_flow( lvgl.FLEX_FLOW.COLUMN )
def area( text, width, height ):
area = lvgl.obj( cont )
area.set_size( width, height )
label = lvgl.label( area )
label.set_text( text )
label.center()
return area
area( 'one', 70, 70 )
area( 'two', 70, 70 )
area( 'three', 70, 70 )
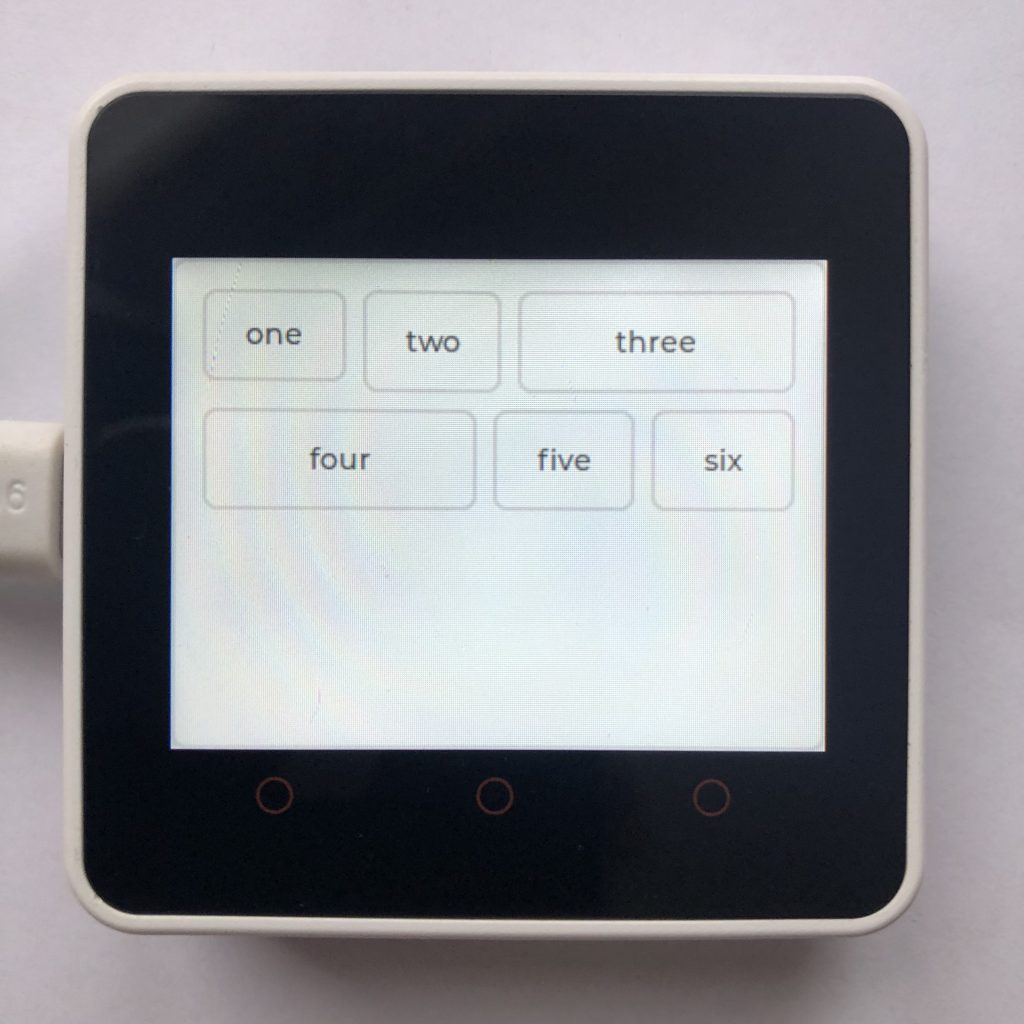
Flex Grow and New Line
Items in a flex layout can be set to fill any unused space in their row or column. This code shows how to set that grow factor, and how to force items onto new lines.
import lvgl
cont = lvgl.obj( lvgl.scr_act() )
cont.set_size( 320, 240 )
cont.center()
cont.set_layout( lvgl.LAYOUT_FLEX.value )
cont.set_flex_flow( lvgl.FLEX_FLOW.ROW_WRAP )
def area( text, width, height ):
area = lvgl.obj( cont )
area.set_size( width, height )
label = lvgl.label( area )
label.set_text( text )
label.center()
return area
def grow_area( text, height, grow ):
area = lvgl.obj( cont )
area.set_flex_grow( grow )
area.set_height( height )
label = lvgl.label( area )
label.set_text( text )
label.center()
return area
area( 'one', 70, 45 )
grow_area( 'two', 50, 1 )
grow_area( 'three', 50, 2 )
grow_area( 'four', 50, 1 ).add_flag( lvgl.obj.FLAG.LAYOUT_1 ) #this is how you start a new line
area( 'five', 70, 50 )
area( 'six', 70, 50 )
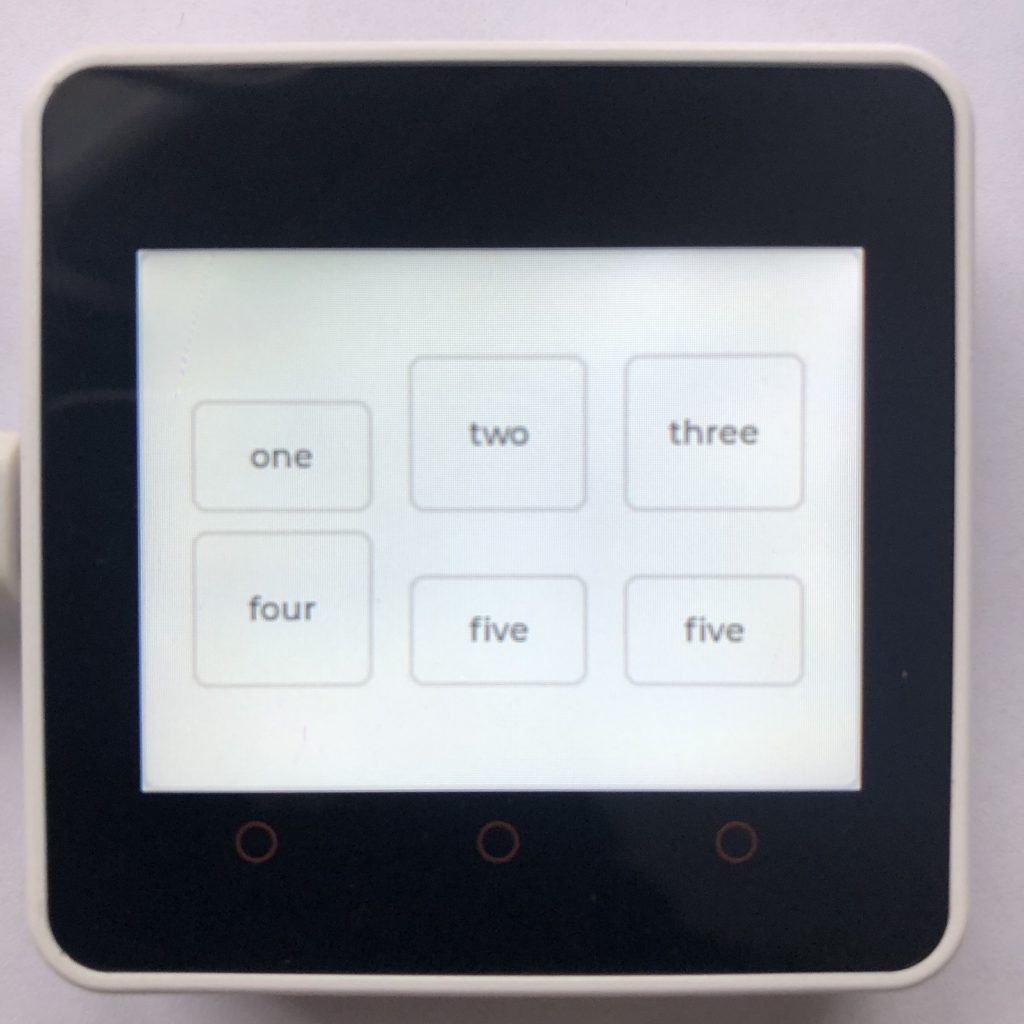
LVGL Flex Align
The flex layout allows you to align its tracks (the rows or columns), and to align the items in the tracks. You can also tell it how to align different sized objects.
import lvgl
cont = lvgl.obj( lvgl.scr_act() )
cont.set_size( 320, 240 )
cont.center( )
cont.set_layout( lvgl.LAYOUT_FLEX.value )
cont.set_flex_flow( lvgl.FLEX_FLOW.ROW_WRAP )
cont.set_flex_align( lvgl.FLEX_ALIGN.SPACE_EVENLY, lvgl.FLEX_ALIGN.END, lvgl.FLEX_ALIGN.CENTER )
def area( text, width, height ):
area = lvgl.obj( cont )
area.set_size( width, height )
label = lvgl.label( area )
label.set_text( text )
label.center()
return area
area( 'one', 80, 50 )
area( 'two', 80, 70 )
area( 'three', 80, 70 )
area( 'four', 80, 70 )
area( 'five', 80, 50 )
area( 'five', 80, 50 )
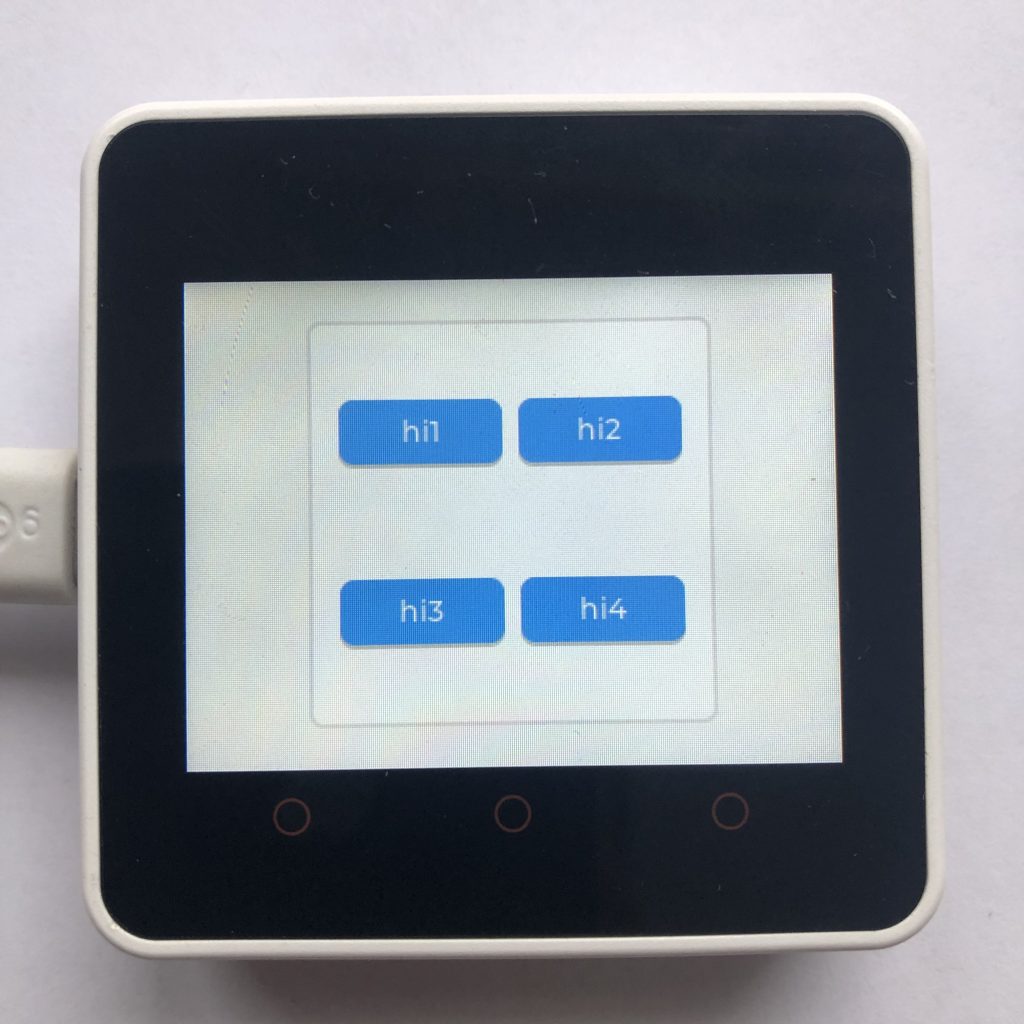
LVGL Grid Basic Example
This Grid layout, as you can see, takes a little more code to get working. But if you can get them to work, they look pretty good.
import lvgl
#you can replace the lvgl.grid_fr(x) with pixel width/height
row = [ lvgl.grid_fr(1), lvgl.grid_fr(1), lvgl.GRID_TEMPLATE.LAST ]
column = [ lvgl.grid_fr(1), lvgl.grid_fr(1), lvgl.GRID_TEMPLATE.LAST ]
cont = lvgl.obj( lvgl.scr_act() )
cont.set_size( 200, 200 )
cont.center()
cont.set_layout( lvgl.LAYOUT_GRID.value )
cont.set_style_grid_row_dsc_array( row, 0 )
cont.set_style_grid_column_dsc_array( column, 0 )
def button( text, row_x, column_y ):
btn = lvgl.btn( cont )
label = lvgl.label( btn )
label.set_text( text )
label.center()
btn.set_grid_cell( lvgl.GRID_ALIGN.STRETCH, row_x, 1, lvgl.GRID_ALIGN.CENTER, column_y, 1 )
return btn
button( 'hi1', 0, 0 )
button( 'hi2', 1, 0 )
button( 'hi3', 0, 1 )
button( 'hi4', 1, 1 )
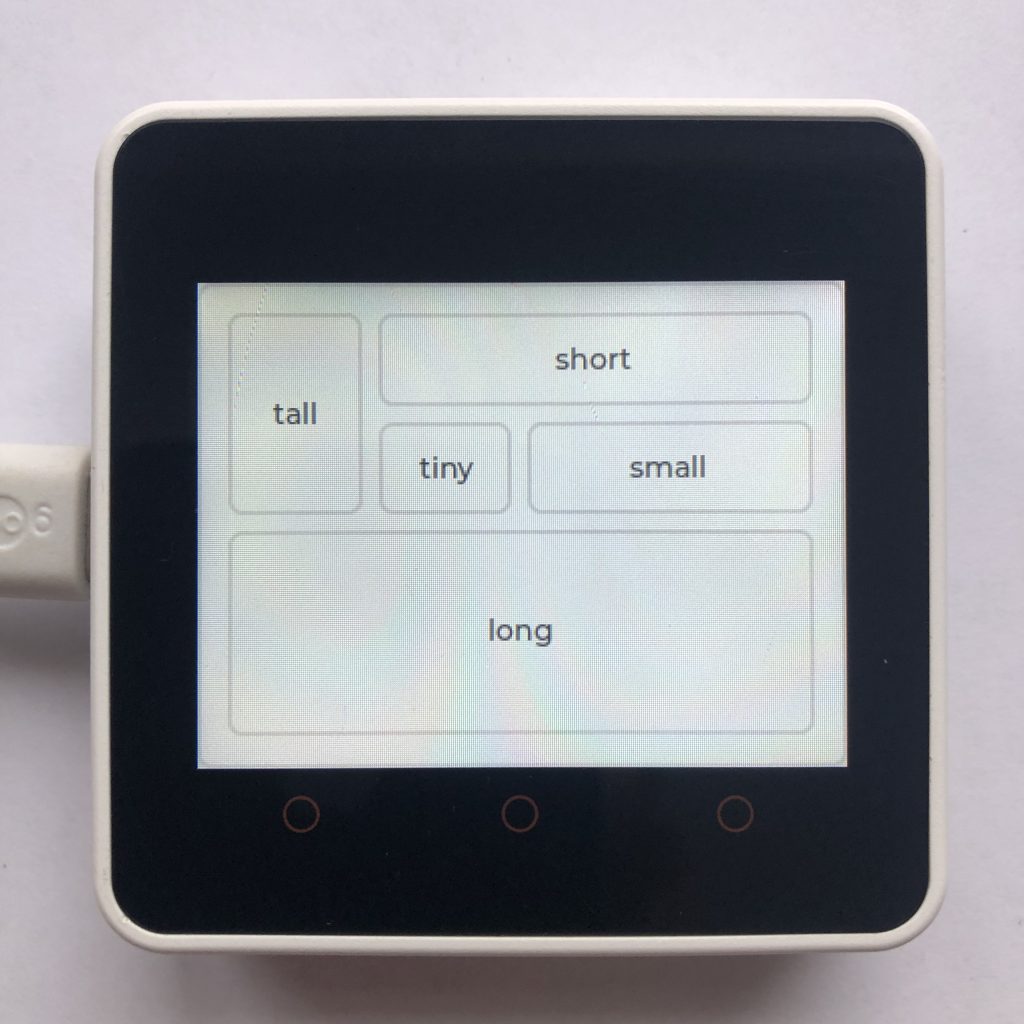
Spacing and Grids
The grid layout allows you to make items span multiple rows or columns.
import lvgl
row = [ lvgl.grid_fr(1), lvgl.grid_fr(1), lvgl.grid_fr(1), lvgl.grid_fr(1), lvgl.GRID_TEMPLATE.LAST ]
column = [ lvgl.grid_fr(1), lvgl.grid_fr(1), lvgl.grid_fr(1), lvgl.grid_fr(1), lvgl.GRID_TEMPLATE.LAST ]
cont = lvgl.obj( lvgl.scr_act() )
cont.set_size( 320, 240 )
cont.center()
cont.set_layout( lvgl.LAYOUT_GRID.value )
cont.set_style_grid_row_dsc_array( row, 0 )
cont.set_style_grid_column_dsc_array( column, 0 )
def area( text, row_x, column_y, width, height ):
area = lvgl.obj( cont )
label = lvgl.label( area )
label.set_text( text )
label.center()
area.set_grid_cell( lvgl.GRID_ALIGN.STRETCH, row_x, width, lvgl.GRID_ALIGN.STRETCH, column_y, height )
return area
area( 'tall', 0, 0, 1, 2 )
area( 'short', 1, 0, 3, 1 )
area( 'tiny', 1, 1, 1, 1 )
area( 'small', 2, 1, 2, 1 )
area( 'long', 0, 2, 4, 2 )
Whats next
Today you have learned how to use both the flex and grid layouts. If you want some more practice, try experimenting with the code. Maybe you could mix buttons and objects in your layouts and see what happens.
Whatever you do, knowing how to use layouts is a great skill to have.