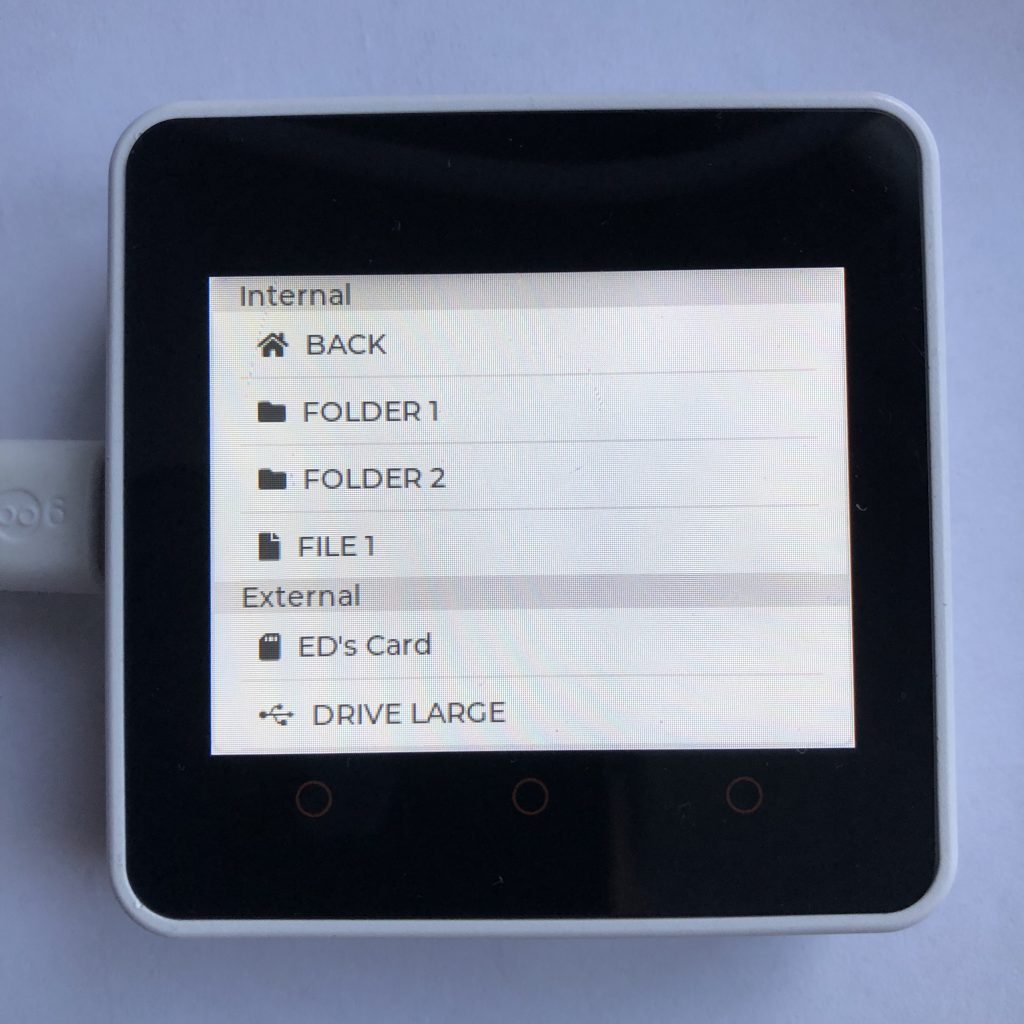
As you probably already know, LVGL is a graphics library. What I’d like to show you today is how to create a list of items, and assign those items to a print command that you can see in the terminal window. This can be really helpful if you are trying to create your own filesystem.
Also, with just a little code modification, you could use this for working directly with any button that is clicked by the user.
When you are finished with this code tutorial today, you’ll be able to tap any of the items in this list, and you’ll see the name of the list item that you clicked in the terminal window. You can then develop additional code that will allow you to interact with this code to create additional actions.
To get this example to work you will need to add your screen code to the beginning of the example. If you want more information try How to get a LVGL Micropython screen to work.
Let’s get started with the code below…
import lvgl
def handler( data ):
theBTN = data.get_target()
print( ls.get_btn_text( theBTN ))
ls = lvgl.list( lvgl.scr_act() )
ls.set_size( 320, 240 )
ls.center()
ls.add_text( 'Internal' )
BACK = ls.add_btn( lvgl.SYMBOL.HOME, 'BACK' )
BACK.add_event_cb( handler, lvgl.EVENT.CLICKED, None )
FOLDER_1 = ls.add_btn( lvgl.SYMBOL.DIRECTORY, 'FOLDER 1' )
FOLDER_1.add_event_cb( handler, lvgl.EVENT.CLICKED, None )
FOLDER_2 = ls.add_btn( lvgl.SYMBOL.DIRECTORY, 'FOLDER 2' )
FOLDER_2.add_event_cb( handler, lvgl.EVENT.CLICKED, None )
FILE = ls.add_btn( lvgl.SYMBOL.FILE, 'FILE 1' )
FILE.add_event_cb( handler, lvgl.EVENT.CLICKED, None )
ls.add_text( 'External' )
CARD = ls.add_btn( lvgl.SYMBOL.SD_CARD, 'ED\'s Card' )
CARD.add_event_cb( handler, lvgl.EVENT.CLICKED, None )
DRIVE = ls.add_btn( lvgl.SYMBOL.USB, 'DRIVE LARGE' )
DRIVE.add_event_cb( handler, lvgl.EVENT.CLICKED, None )
Understanding This LVGL Code
Ok, now that you have seen all the code, let’s step through each section, so you can understand exactly what each part does. This will make it much easier to actually use the code in your future projects…
The Import Command
This basically imports the lvgl library, so we can use it to put things on our display.
import lvgl
The Terminal Printing Code
First, we will declare the handler function. Next, we will get the target button and store it in a variable. Finally, we will use the print command to print the name of the button to the terminal window.
def handler( data ):
theBTN = data.get_target()
print( ls.get_btn_text( theBTN ))
Creating The List And Declaring The Size
Here, we are telling LVGL that we want to use the list feature. We will also declare the size of the list and use and some formatting options so that our list displays correctly on the screen.
ls = lvgl.list( lvgl.scr_act() )
ls.set_size( 320, 240 )
ls.center()
Adding The First Label To The FileSystem View
We’ll use the add_text command to print a label to our screen with the word, “Internal”.
ls.add_text( 'Internal' )
Adding The Back/Home Button
Here, we are going to give our button the name “Back”, and then we will add the button to the display, and use the LVGL symbol “HOME” so that it shows on the screen.
You can see in the example screenshot that this symbol is a little house icon. In a true file system, clicking this button would take the user back to the main page or home page of the application.
BACK = ls.add_btn( lvgl.SYMBOL.HOME, 'BACK' )
BACK.add_event_cb( handler, lvgl.EVENT.CLICKED, None )
Adding The Folder Icons To The FileSystem List
In this code, we will create two more filesystem items to add to our list. We will give the items the names “Folder 1” and “Folder 2”, which will be shown on the display.
Notice that this time, we have chosen to use the LVGL symbol for a Directory, which is this folder icon you see in the example above.
FOLDER_1 = ls.add_btn( lvgl.SYMBOL.DIRECTORY, 'FOLDER 1' ) FOLDER_1.add_event_cb( handler, lvgl.EVENT.CLICKED, None )
FOLDER_2 = ls.add_btn( lvgl.SYMBOL.DIRECTORY, 'FOLDER 2' ) FOLDER_2.add_event_cb( handler, lvgl.EVENT.CLICKED, None )
Adding Files To The FileSystem List
This code is very similar to the Folder code above, but in this case, we are going to display a file icon and call the item, ‘FILE 1’. All the other code is the same as in the previous example.
FILE = ls.add_btn( lvgl.SYMBOL.FILE, 'FILE 1' )
FILE.add_event_cb( handler, lvgl.EVENT.CLICKED, None )
Adding Additional Labels To The FileSystem List
This code below is identical to the “Internal” label that you added earlier. You can see how you can use the combination of labels and icons to create a visual filesystem that has structure to it.
ls.add_text( 'External' )
Adding External Memory/SD Card Icons To The FileSystem List
This code will declare a “Card” button value, and we will use the built in LVGL symbol for an SD card. In a filesystem, you could use this to allow users to access the filesystem of an inserted SD card into their device.
CARD = ls.add_btn( lvgl.SYMBOL.SD_CARD, 'ED\'s Card' )
CARD.add_event_cb( handler, lvgl.EVENT.CLICKED, None )
Adding External Drive Icons To The FileSystem List
This code is very similar to the code above, but uses the USB icon from the LVGL library. You can imagine how you might use this icon and structure in a real filesystem list that you would interact with on your device.
DRIVE = ls.add_btn( lvgl.SYMBOL.USB, 'DRIVE LARGE' )
DRIVE.add_event_cb( handler, lvgl.EVENT.CLICKED, None )
What Happens Next?
Ok, now that you understand how this code works and can use the different types of symbols and the LVGL list feature, you can see how this can be used to build your own filesystem.
Try out the code above, and notice that when you click on any of the menu items, you will see the name of the button that was clicked on your terminal screen.
Now, you can imagine how this can be used to interact with the touch screen. You could develop your code further so that when a button is pushed, and the terminal reads the data, it then begins to process this further and execute additional code.
Using this simple code is a great way to get started with building a working filesystem so that you can interact with stored information on your device.
If you haven’t learned how to use the Flex and Grid layout features, these micropython lvgl layout examples would be a good place to go next to learn these features…