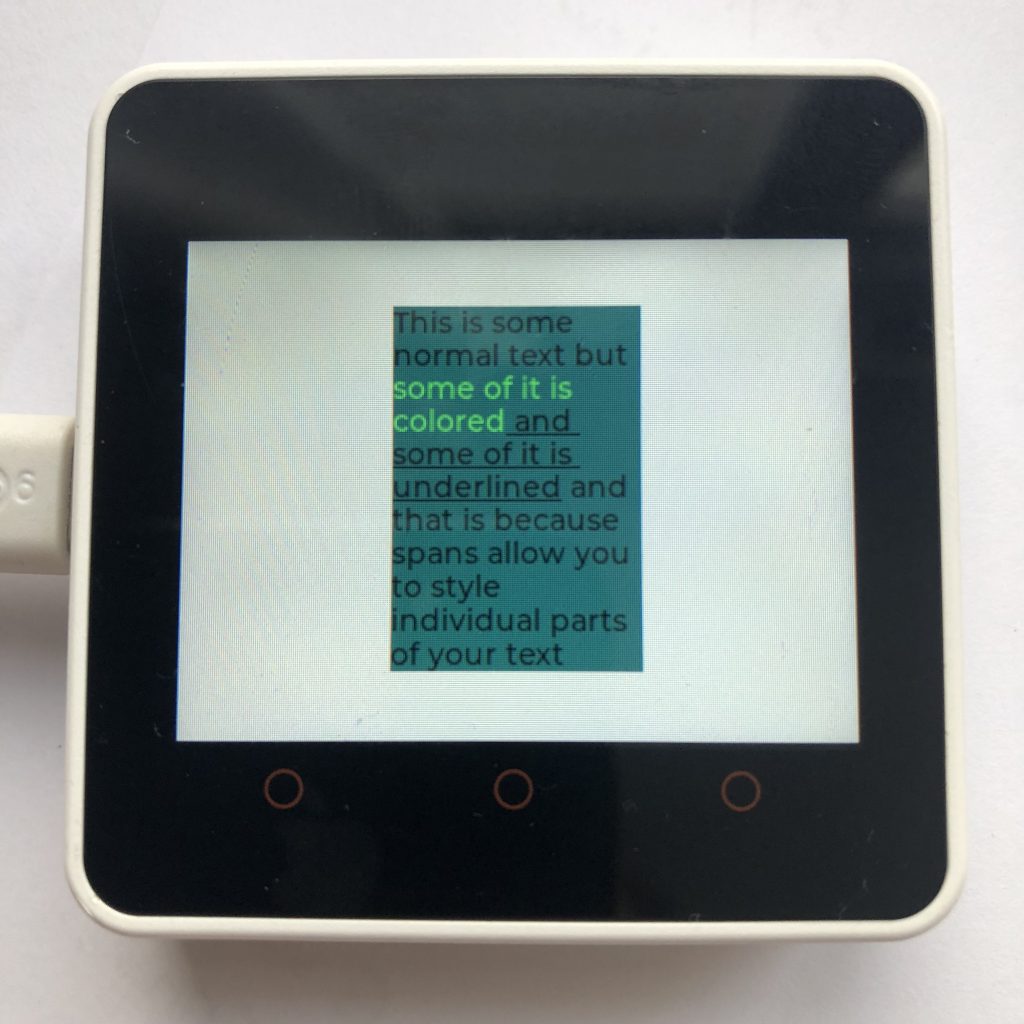
The LVGL library has a lot of powerful features for styling text. You can set the color, underline the text, set the opacity, etc., but if you want to style a small part of some text instead of the entire thing, that gets a little more complicated. The best way to pull that off is with the span.
What Is A Span?
The span is an LVGL widget that is similar to the label. Think of them like a container that holds a bunch of individual labels inside. Spans take care of all the details like word wrap and line positioning, and let you take care of the other details like styling the text.
How Do You Use Spans?
The rough idea is that you create a Spangroup(aka. Span), and inside of it you create spans. Spans are little snippets of text, plus any styles you want to add to that text. To make the process a little clearer I have included an example below. Down there is also an explanation of how the example works.
Here is that code:
import lvgl
group = lvgl.spangroup(lvgl.scr_act())
group.set_size(120,100)
group.set_mode(lvgl.SPAN_MODE.BREAK)
group.set_style_bg_color(lvgl.color_hex(0x005050),0)
group.set_style_bg_opa(255,0)
group.set_style_text_color(lvgl.color_hex(0x000000),0)
group.center()
span = group.new_span()
span.set_text('This is some normal text but')
other_span = group.new_span()
other_span.set_text(' some of it is colored')
other_span.style.set_text_color(lvgl.color_hex(0x00FF50))
other_other_span = group.new_span()
other_other_span.set_text(' and some of it is underlined')
other_other_span.style.set_text_decor(lvgl.TEXT_DECOR.UNDERLINE)
other_other_other_span = group.new_span()
other_other_other_span.set_text(' and that is because spans allow you to style individual parts of your text')
group.refr_mode()
Understanding This LVGL Code
Ok, now that you have seen all the code, let’s step through each section, so you can understand exactly what each part does. This will make it much easier to actually use the span in your projects…
The Setup
First we have to import LVGL so we can use it.
import lvgl
Setting Up the Spangroup
To start, we need to create an empty spangroup.
group = lvgl.spangroup(lvgl.scr_act())
Then we will set its size to 120 pixels wide and 100 pixels high.
group.set_size(120,100)
If you put more text into the span than it can hold, it will normally cut off that extra text, but Spans have modes to control what they do. Below, I set the mode to BREAK, which makes the span increase its height if it has to much text.
If you don’t care what it does, then you can remove this line of code entirely and the span will continue to work fine.
group.set_mode(lvgl.SPAN_MODE.BREAK)
After that we will change the background color to a greenish blue.
group.set_style_bg_color(lvgl.color_hex(0x005050),0)
The background of spans is normally invisible, so to see the new color we added, we need to make the background visible by setting its opacity to 255.
group.set_style_bg_opa(255,0)
To make the text more readable we are going to change its color to black. This code will change the default color but later we can override it to highlight portions of the text that we want.
group.set_style_text_color(lvgl.color_hex(0x000000),0)
Lastly we will center the span because that makes it look a little better.
group.center()
Creating the First Span
It is finally time to actually create a span which is not to be confused with the spangroup.
span = group.new_span()
After that we set its text.
span.set_text('This is some normal text but')
The Other Span
This span is colored greenish. We start just like we did last time by creating a new span.
other_span = group.new_span()
Then we set its text.
other_span.set_text(' some of it is colored')
Here is where it gets interesting. We are going to add a style to the span to change the color. Take a good look at the code below, because for some reason, the way you add styles to spans is done differently than normal styles.
I haven’t seen any other object that uses styles this way. It seems to be unique to spans.
other_span.style.set_text_color(lvgl.color_hex(0x00FF50))
The Underlined Span
In this span, we are going to underline the text, but first we have to create it.
other_other_span = group.new_span()
After that the text needs to be set.
other_other_span.set_text(' and some of it is underlined')
Add then we add the style that underlines the text.
other_other_span.style.set_text_decor(lvgl.TEXT_DECOR.UNDERLINE)
The Last Span
This last span is again set to the default settings and works like all the previous ones. First we create it.
other_other_other_span = group.new_span()
Then we set its text.
other_other_other_span.set_text(' and that is because spans allow you to style individual parts of your text')
Making Every Thing Work Right
This last line of code is very important. Whenever we change something in the spangroup, like creating a span, changing some text, or adding a style, we need to refresh the main spangroup otherwise our updates might not be displayed.
The code below is what calls that refresh. Thankfully, it’s pretty simple.
group.refr_mode()
What Next?
Now that you know how the above code works, try running it. Your screen should look like the picture at the top of this page. Hopefully, in spite of my poor choice of colors, it is clear what spans can do and how useful they are.
One of the best features about spans is that they are similar to labels, so if you have created a project that uses labels, it is not too complicated to update those labels with spans.
If you’re looking for useful ways to display text on your screens then Micropython LVGL Textarea Examples might be able to interest you.