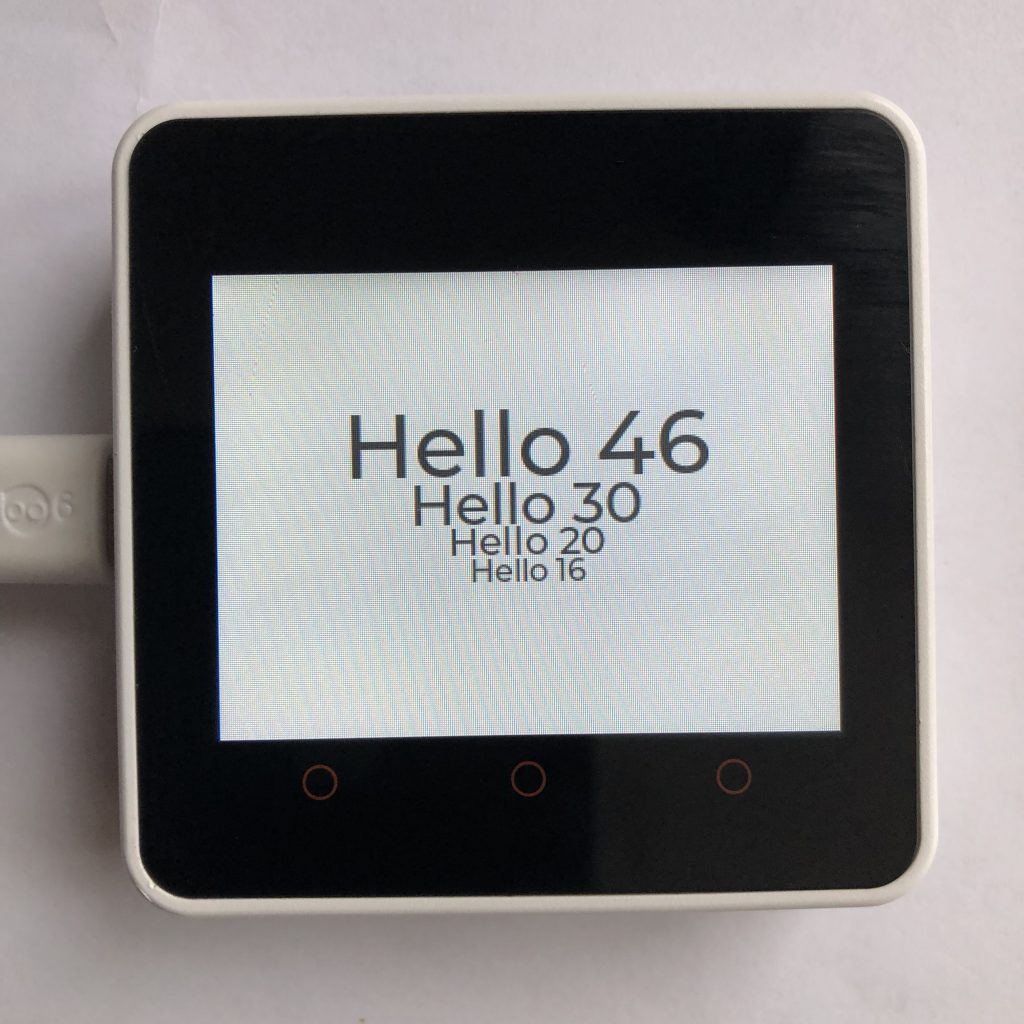
Being able to change fonts is extremely important. Unfortunately It takes a bit of work to get new fonts onto microcontrollers. Thankfully once they are there, LVGL makes it easy to use them.
Today I will show you how to add some extra fonts to your board and use those fonts with LVGL. There is also some example code at the bottom to test if your fonts work.
Compiling
To add new fonts, you need to recompile micropython LVGL. This tutorial is not intended to teach you how to compile micropython LVGL. If you are not familiar on how that is done, the best place to find information is in the readme files in the micropython LVGL source code.
Make sure to follow the instructions at the main readme and your boards readme, for example here is what I use Micropython Readme, Esp32 Readme, and Core2 Readme.
Adding Builtin Fonts
To add a font, we need to enable it in the lv_conf.h file. Of course the first step is to find the lv_conf.h file.
First find the lv_micropython folder. If you have compiled LVGL before, then this should already be downloaded onto your computer. Usually its put in your root directory.
Inside of the lv_micropython folder, find and enter the lib folder.
Then find and enter the lv_binding folder. Your path should look something like: Home/lv_micropython/lib/lv_binding
Inside of the lv_binding folder you will find the lv_conf.h file.
Editing lv_conf.h
The lv_conf.h file controls pretty much all of LVGL. Open it in your text editor and around line 346, you should see a list of fonts similar to the picture below.
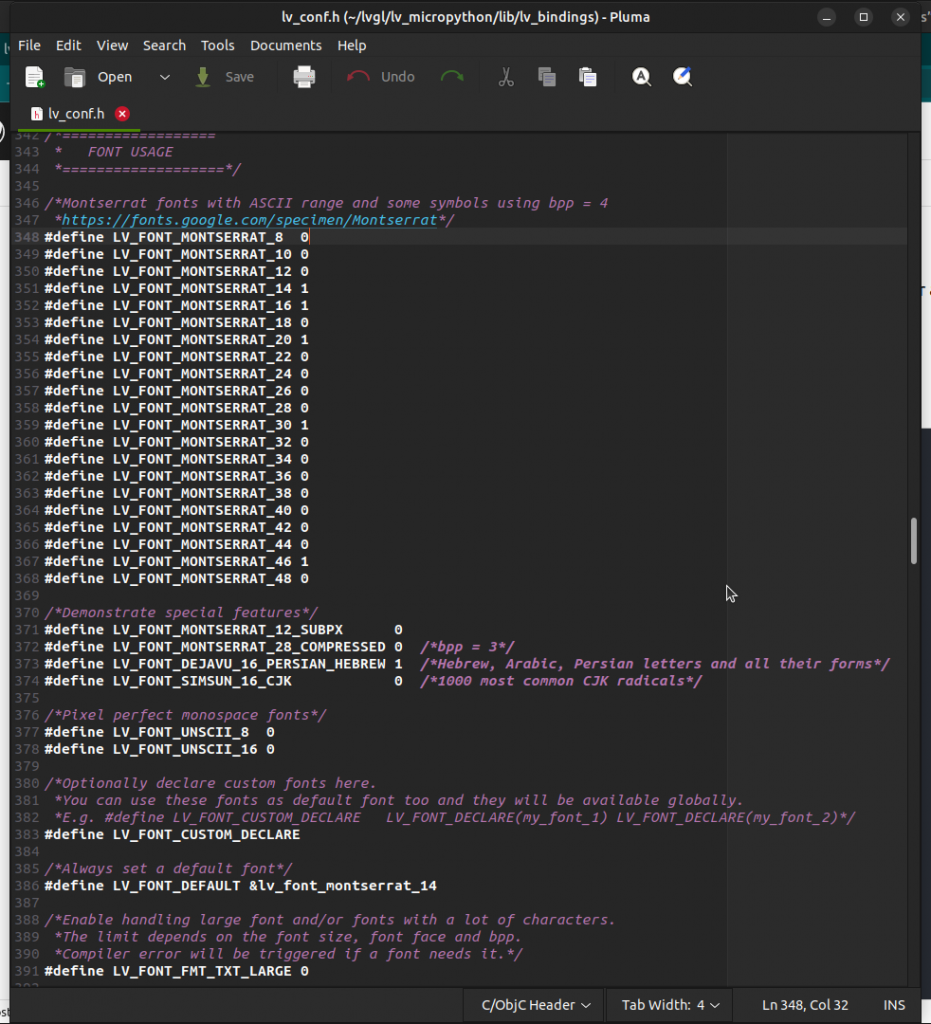
In each font name there is a number, that number is equal to how many pixels that font is high for example in LV_FONT_MONTSERRAT_30 each letter should be 30 pixels high.
Next to each font name there is ether a 1 or a 0. If the number is a one the font is added to LVGL if it is zero its not added. You can see from the picture above that I have the 14,16,20,30, and 46 size fonts enabled.
All you have to do now is add a one next to the fonts you want, save the file, and then recompile LVGL as normal, and the fonts you chose will be added to LVGL.
Fonts take a lot of memory, so if you add to many fonts your computer might not have enough memory to work. Its usually best if you only enable the fonts you know you will use.
Using Your Fonts with LVGL
Styles are what controls fonts. The most basic way you can use styles to change the font is like this.
YourObject.set_style_text_font(lvgl.YourFontName,0)
You will need to replace YourObject with what ever object you want to change the font of, and replace YourFontName with the name of your font, usually in lower case. Below is some code that shows this process in action.
The Screen
To get this or any code for that matter to work, you need to enable a screen for LVGL. If you don’t know how to do that then there is a little more information at How to get a LVGL Micropython screen to work.
import lvgl
big_lb = lvgl.label(lvgl.scr_act())
big_lb.set_style_text_font(lvgl.font_montserrat_46,0)
big_lb.align(lvgl.ALIGN.CENTER,0,-30)
big_lb.set_text('Hello 46')
large_lb = lvgl.label(lvgl.scr_act())
large_lb.set_style_text_font(lvgl.font_montserrat_30,0)
large_lb.align(lvgl.ALIGN.CENTER,0,0)
large_lb.set_text('Hello 30')
mid_lb = lvgl.label(lvgl.scr_act())
mid_lb.set_style_text_font(lvgl.font_montserrat_20,0)
mid_lb.align(lvgl.ALIGN.CENTER,0,20)
mid_lb.set_text('Hello 20')
default_lb = lvgl.label(lvgl.scr_act())
default_lb.set_style_text_font(lvgl.font_montserrat_16,0)
default_lb.align(lvgl.ALIGN.CENTER,0,35)
default_lb.set_text('Hello 16')
Other Options
You now can enable any of the default fonts LVGL has available but you might want a little more variaty for your projects. Thankfully you can add any font you want to LVGL. You just have to process them with LVGL Font Converter.
If you don’t want to go through that process there is a way around it. Down near the bottom of the lv_conf.h file there are some settings for enabling TTF fonts.
If enabled it is possible to load a normal font from the file system, meaning this method doesn’t even require you to recompile the the firmware when you add a new font.
In the code above we had to create a individual label for for each font we wanted to add. Because of how annoying this is, LVGL has the span. Spans are like labels but you can have more than one type of font in them.
If you are interested in spans you can learn more at LVGL Spans.