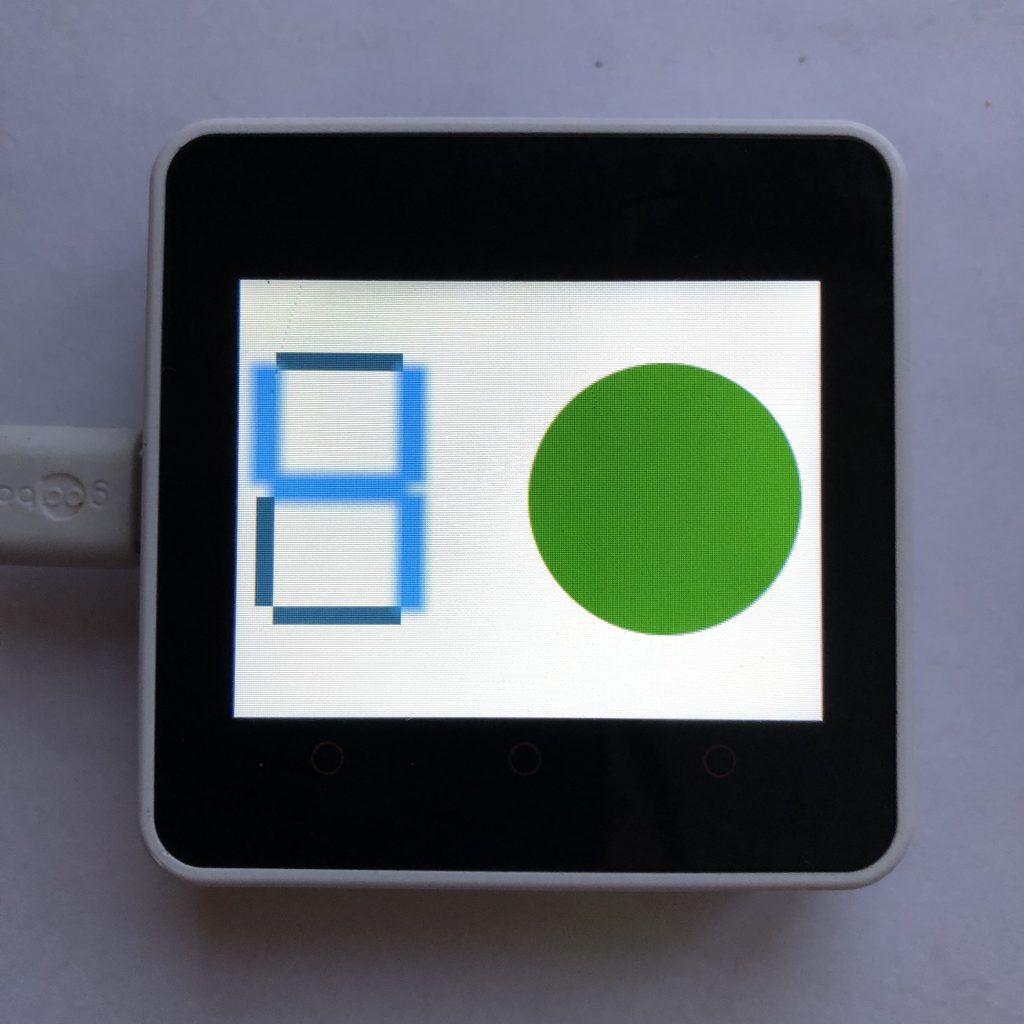
LVGL has several widgets intended to polish off your projects. LEDs are one of them. They are very simple to use because they do all of the color math and styling for you.
Today, I will show you how to create a LED and also how to use LEDs to make a 7 segment display. We will do that by walking through how the code down below works.
Setting Up a Micropython Screen
Obviously, if you are creating a program that draws things on a screen, you have to have access to a screen.
Because there are so many different screens out there, I can’t add support for all of them, so to make this example work, you will need to add your screen’s setup code to the beginning of the example.
There is a little more information on this process at How to get a LVGL Micropython Screen to Work if you aren’t familiar on how it’s done.
Here is that code
import lvgl
from time import sleep
led1 = lvgl.led(lvgl.scr_act())
led1.set_brightness(100)
led1.set_color(lvgl.color_hex(0x00ff00))
led1.set_pos(160,45)
led1.set_size(150,150)
def add_led(x,y,w,h):
led = lvgl.led(lvgl.scr_act())
led.set_style_radius(0,0)
led.set_pos(x,y)
led.set_size(w,h)
led.off()
return led
t = add_led(20,40,70,10)
m = add_led(20,110,70,10)
b = add_led(20,180,70,10)
tl = add_led(10,50,10,60)
tr = add_led(90,50,10,60)
bl = add_led(10,120,10,60)
br = add_led(90,120,10,60)
def display(top,middle,bottom,top_left,top_right,bottom_left,bottom_right):
if top:
t.on()
else:
t.off()
if middle:
m.on()
else:
m.off()
if bottom:
b.on()
else:
b.off()
if top_left:
tl.on()
else:
tl.off()
if top_right:
tr.on()
else:
tr.off()
if bottom_left:
bl.on()
else:
bl.off()
if bottom_right:
br.on()
else:
br.off()
def update(num):
if num == 0:
display(1,0,1,1,1,1,1)
if num == 1:
display(0,0,0,0,1,0,1)
if num == 2:
display(1,1,1,0,1,1,0)
if num == 3:
display(1,1,1,0,1,0,1)
if num == 4:
display(0,1,0,1,1,0,1)
if num == 5:
display(1,1,1,1,0,0,1)
if num == 6:
display(1,1,1,1,0,1,1)
if num == 7:
display(1,0,0,0,1,0,1)
if num == 8:
display(1,1,1,1,1,1,1)
if num == 9:
display(1,1,0,1,1,0,1)
while True:
for x in range(10):
update(x)
led1.set_brightness(x*20)
sleep(1)
Understanding This Code
Just looking at a block of code is not always very informational. To make how this micropython code works more clear, lets walk through the lines of code above and see what they do.
The Setup
Before we can use LVGL we need to import it. This is also a good spot to add your screen setup code.
import lvgl
Some of this code requires special timing, so we import the sleep function from the time module.
from time import sleep
Creating a Simple LED
Will start by creating a LED.
led1 = lvgl.led(lvgl.scr_act())
Next we will set that LED’s brightness to 100. The brightness range is between 0 and 255.
led1.set_brightness(100)
Then we will set the color of the LED to green.
led1.set_color(lvgl.color_hex(0x00ff00))
After that we set its position.
led1.set_pos(160,45)
Lastly we set its size to 150 pixels wide by 150 pixels tall.
led1.set_size(150,150)
Simplifying the Process
We are going to have to create a lot of LEDs, so lets create a function that will make that process easier.
To start we define the function.
def add_led(x,y,w,h):
After that we create a new LED.
led = lvgl.led(lvgl.scr_act())
Then we set the radius to zero. This makes the LEDs square instead of Circular.
led.set_style_radius(0,0)
Next we set the position of the LED and its size.
led.set_pos(x,y)
led.set_size(w,h)
After that we turn it off which is the same as setting the brightness to 0.
led.off()
Finally we return the LED object we created, so the program can use it.
return led
Creating Lots of LEDs
For the 7 segment display, we need 7 LEDs so lets create them.
We will use that function we made earlier to create our LEDS. It takes four numbers; the first two numbers are the x and y coordinates of the led and the second two numbers are the width and height of the led.
We start by creating the top,middle, and bottom LEDs.
t = add_led(20,40,70,10)
m = add_led(20,110,70,10)
b = add_led(20,180,70,10)
Then we create the top_left and top_right LEDs.
tl = add_led(10,50,10,60)
tr = add_led(90,50,10,60)
Lastly we create the bottom left and bottom right LEDs.
bl = add_led(10,120,10,60)
br = add_led(90,120,10,60)
The Control Function
The next thing we are going to do is create a function to control all of those LEDs.
We begin by defining it.
def display(top,middle,bottom,top_left,top_right,bottom_left,bottom_right):
After that we check if the top LED should be on.
if top:
t.on()
else:
t.off()
I won’t bore you with the process, but we do the exact same thing for the rest of the LEDs.
if middle:
m.on()
else:
m.off()
if bottom:
b.on()
else:
b.off()
if top_left:
tl.on()
else:
tl.off()
if top_right:
tr.on()
else:
tr.off()
if bottom_left:
bl.on()
else:
bl.off()
if bottom_right:
br.on()
else:
br.off()
Numbers to LEDs
We want the leds to display numbers. So we are going to create one last function. We will give this function a number like 4 and it figures out which LEDs should be turned on to show the number four.
As usual we start by defining the function.
def update(num):
Then we check if the number given to this function is the number zero.
if num == 0:
If it was we turn on the correct LEDs to display zero.
display(1,0,1,1,1,1,1)
We repeat this process for the numbers 1-9.
if num == 1:
display(0,0,0,0,1,0,1)
if num == 2:
display(1,1,1,0,1,1,0)
if num == 3:
display(1,1,1,0,1,0,1)
if num == 4:
display(0,1,0,1,1,0,1)
if num == 5:
display(1,1,1,1,0,0,1)
if num == 6:
display(1,1,1,1,0,1,1)
if num == 7:
display(1,0,0,0,1,0,1)
if num == 8:
display(1,1,1,1,1,1,1)
if num == 9:
display(1,1,0,1,1,0,1)
Generating The Numbers
The last thing we want to do is make the LEDs display different numbers.
First we create a infinite loop.
while True:
Next we use a for loop to generate our numbers.
for x in range(10):
Then we update the LEDs to equal x ,which will be a number from 0-9, and we also change the brightness of the original LED.
update(x)
led1.set_brightness(x*20)
Lastly, we tell the computer to wait one second before it does anything else.
sleep(1)
What Should Happen
Once you run the above code, you should see a number next to a green circle. If you let the program keep running the number get bigger and bigger and the circle get brighter. Eventually the number reaches 9 and the hole thing starts back from 0 and the circle goes dark.
LEDs are great because they can be made into almost anything. You can create complex displays like we did today, or you can create a simple dot that turns on and off.
A lot of the control of LEDS comes from using styles, if you want to learn more about how they work LVGL Style Examples might interest you.