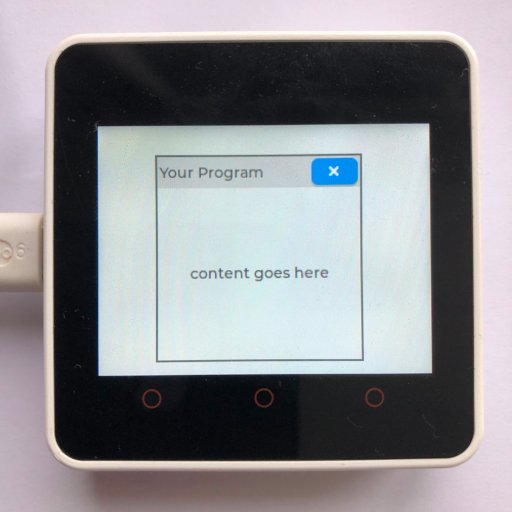
Its easy to make a ridged GUI. Everything has a place that it always stays in, and while this type of program is easy to build. Its not the funnest to use, so its best to allow the user to be able to customize the interface a bit.
Windows are one of the simplest ways to pull this off. They allow you to group a bunch of objects together, and the user can move that group around the screen. Today we will look at how to create a LVGL window and make it dragable.
Setting Up a Micropython Screen
Because there are so many different screens out there, I can’t add support for all of them to the example, so to make the example work, you will need to add your screen’s setup code to the beginning of it.
There is a little more information on this process at How to get a LVGL Micropython Screen to Work if you aren’t familiar on how it’s done.
Here is the example
import lvgl
window = lvgl.win(lvgl.scr_act(),30)
window.set_size(200,200)
window.set_style_border_side(lvgl.BORDER_SIDE.FULL,0)
window.set_style_border_width(2,0)
window.set_style_border_color(lvgl.color_hex(0x454545),0)
window.add_title('Your Program')
close = window.add_btn(lvgl.SYMBOL.CLOSE,45)
def close_window(data):
window.delete()
close.add_event_cb(close_window,lvgl.EVENT.CLICKED,None)
cont = window.get_content()
text = lvgl.label(cont)
text.set_text('content goes here')
text.center()
def follow(data):
input_dev = lvgl.indev_get_act()
move = lvgl.point_t()
input_dev.get_vect(move)
window.set_pos(window.get_x()+move.x,move.y+window.get_y())
header = window.get_header()
header.add_event_cb(follow,lvgl.EVENT.PRESSING,None)
How Does This Code Work?
Since you’ve seen the program above, why don’t we walk through the code and see how it works. This should make it clearer how you can modify this code for your own uses…
The Setup
First we need to import the LVGL module. This is also a good spot to put your screen setup code.
import lvgl
The Window
We start by creating a empty window. The 30 tells the computer to make the header section 30 pixels tall.
window = lvgl.win(lvgl.scr_act(),30)
Then we will set the windows size to 200*200 pixels.
window.set_size(200,200)
Our window look a lot like the background, and it can sometimes be hard to tell where it is on the screen, so we will create a border around our window to make it’s position clear.
To begin we need to have our window enable borders on all sides.
window.set_style_border_side(lvgl.BORDER_SIDE.FULL,0)
Then we will set the border width to 2 pixels.
window.set_style_border_width(2,0)
Then we will set the border color to a dark grey.
window.set_style_border_color(lvgl.color_hex(0x454545),0)
The Header Content
Windows have a header section. Often some text or a button is put there. To show how its done we will add both.
First we will add a tittle.
window.add_title('Your Program')
Then we will add a button. It will be 45 pixels long and have the close symbol in the center of it.
close = window.add_btn(lvgl.SYMBOL.CLOSE,45)
Next we are going to create a function that will delete our window.
def close_window(data):
window.delete()
Finally we connect that function to the close button, so when the button is clicked the the window is deleted from the screen.
close.add_event_cb(close_window,lvgl.EVENT.CLICKED,None)
The Content
We have created the window, but now we need something to put in it. The first step in that process is to retrieve the content area of the window.
cont = window.get_content()
Then, we create a label inside of the content area.
text = lvgl.label(cont)
Next, we will set that label’s text.
text.set_text('content goes here')
And lastly, we center it to the window’s content area.
text.center()
Making the Window Dragable
You can’t just click a window and it move to were you want it by default, but that is what we want it to be able to do today.
To start we need to create a new function.
def follow(data):
In side that function we retrieve the current input device in my case that is a touch screen.
input_dev = lvgl.indev_get_act()
After that we create a empty point object.
move = lvgl.point_t()
Next we set that point equalt to the movement of the input deviece.
input_dev.get_vect(move)
Lastly we move the window in the direct of the input device.
window.set_pos(window.get_x()+move.x,move.y+window.get_y())
We want to make the window move if you click the header, but not move if you click the body of the window, so we retrieve the window’s header.
header = window.get_header()
Then we connect that function we made to the header.
header.add_event_cb(follow,lvgl.EVENT.PRESSING,None)
The Result
Once you run the program, the window should appear. If you click the header, the dark bar at the top, and drag it, the window will follow your finger. If you click the close button the window will be deleted and disappear.
Windows are a great way to organize things on your screen. It is best if you have a larger screen and a cursor, but with a little creativity they can work great for small touch screens as well.
A good replaysment for windows on small screens is tileviews. If you want to learn more about what they are and how they work check out LVGL Tileviews.