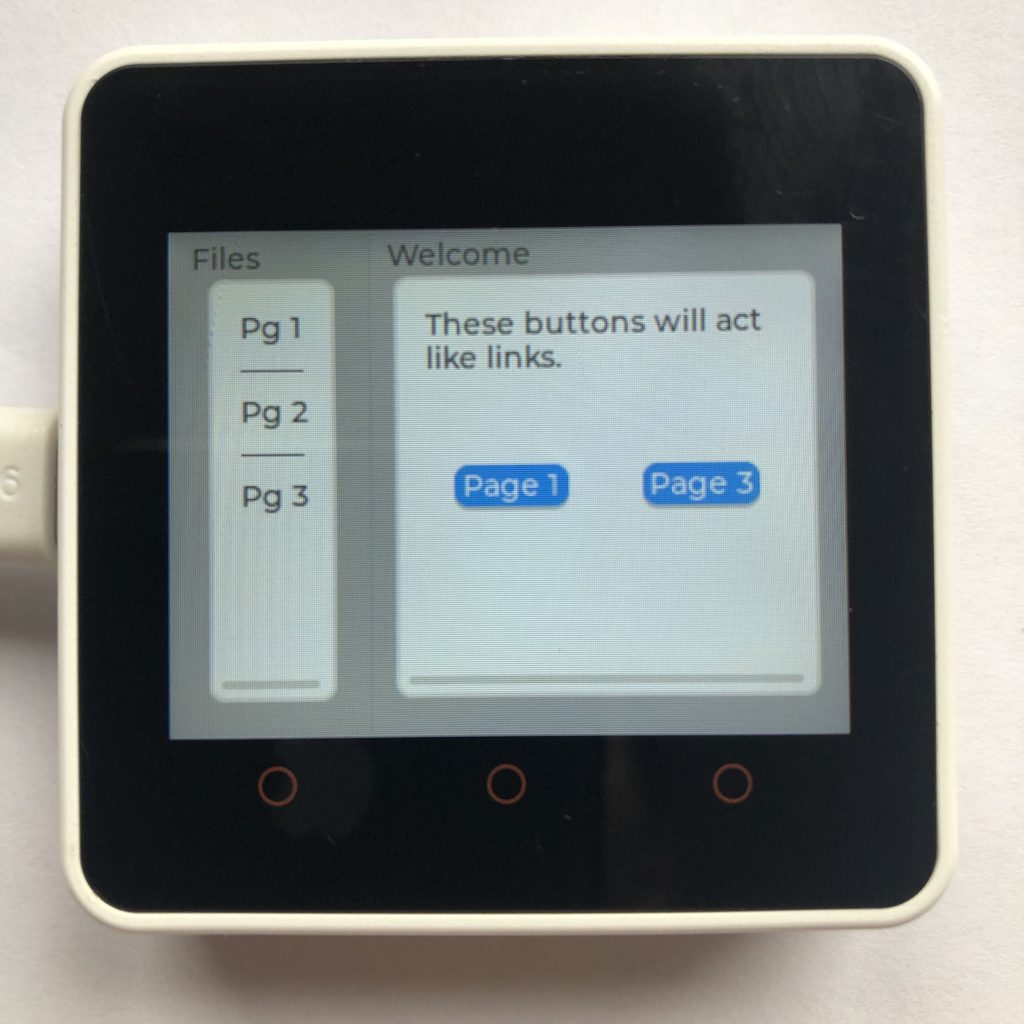
What This Tutorial Will Teach You…
If you want to create a system that has pages that you can move around in, like a browser or file system, then menus are what you want.
This post is a tutorial on how to create menus and edit their pages.
Getting a Screen to Work In Micropython
This is some simple code to get a ili9341 display to work with micropython lvgl. You will want to add it or your own screen code to the beginning of all the examples on this page.
Instead of putting it at the beginning of your code you could just put this code in the micropython boot file. It seems to work best there.
import lvgl
#this is the code that works for my screen:
#from ili9XXX import ili9341
#screen = ili9341(mosi=23, miso=38, clk=18, dc=15, cs=5, invert=True rot=0x10, width=320, height=240 )
# this is some generic code that might work better for you
from ili9XXX import ili9341
screen = ili9341(mosi=23, miso=38, clk=18, dc=15, cs=5,width=320, height=240)
#You will want some touch screen code
#here is what I use
from ft6x36 import ft6x36
touch = ft6x36()
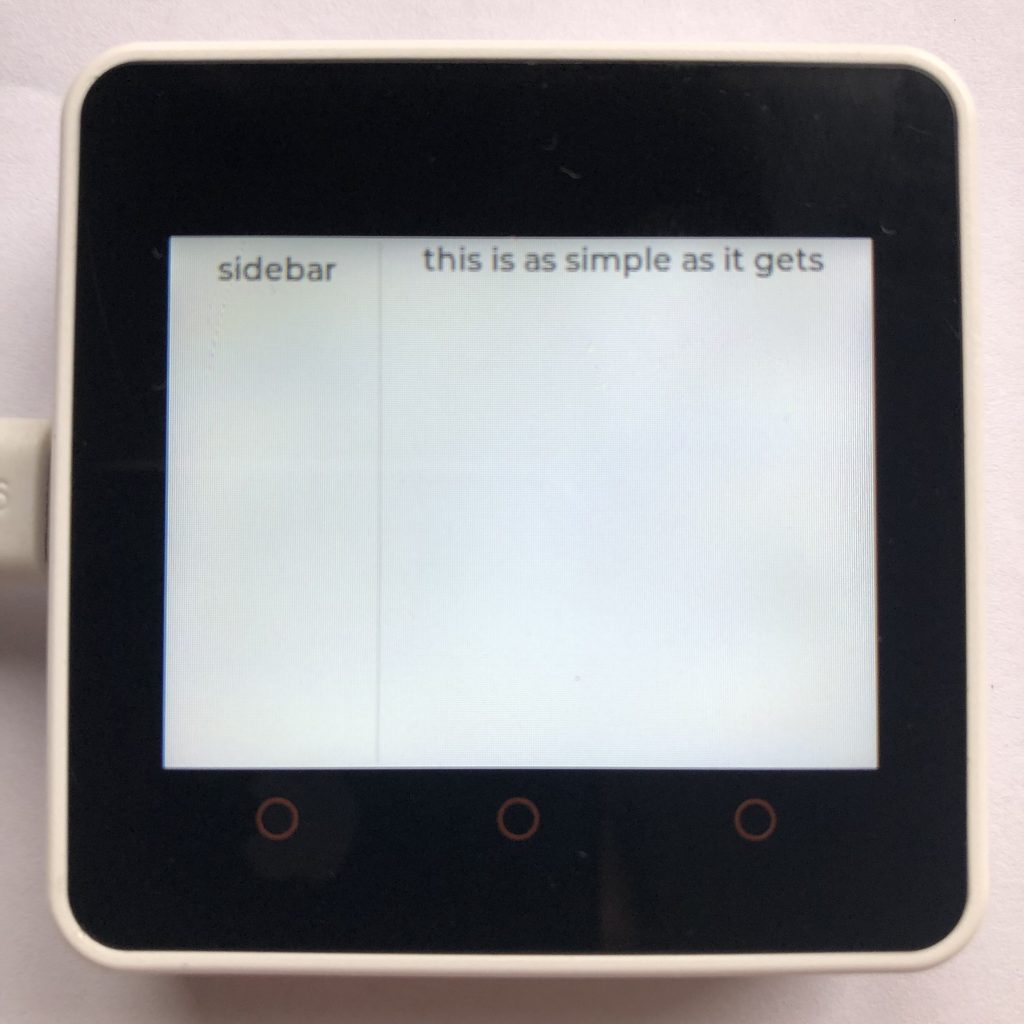
Simple Menu
This code creates a menu with a page and a sidebar. It shows the basics of creating menus.
import lvgl
Menu = lvgl.menu( lvgl.scr_act() )
Menu.set_size( 320, 240 )
#create the main page
Home_Page = lvgl.menu_page( Menu, None )
label = lvgl.label( Home_Page )
label.set_text( 'this is as simple as it gets' )
#create a sidebar
Side_Page = lvgl.menu_page( Menu, None )
label2 = lvgl.label( Side_Page )
label2.set_text( 'sidebar' )
#make them visable
Menu.set_page( Home_Page )
Menu.set_sidebar_page( Side_Page )
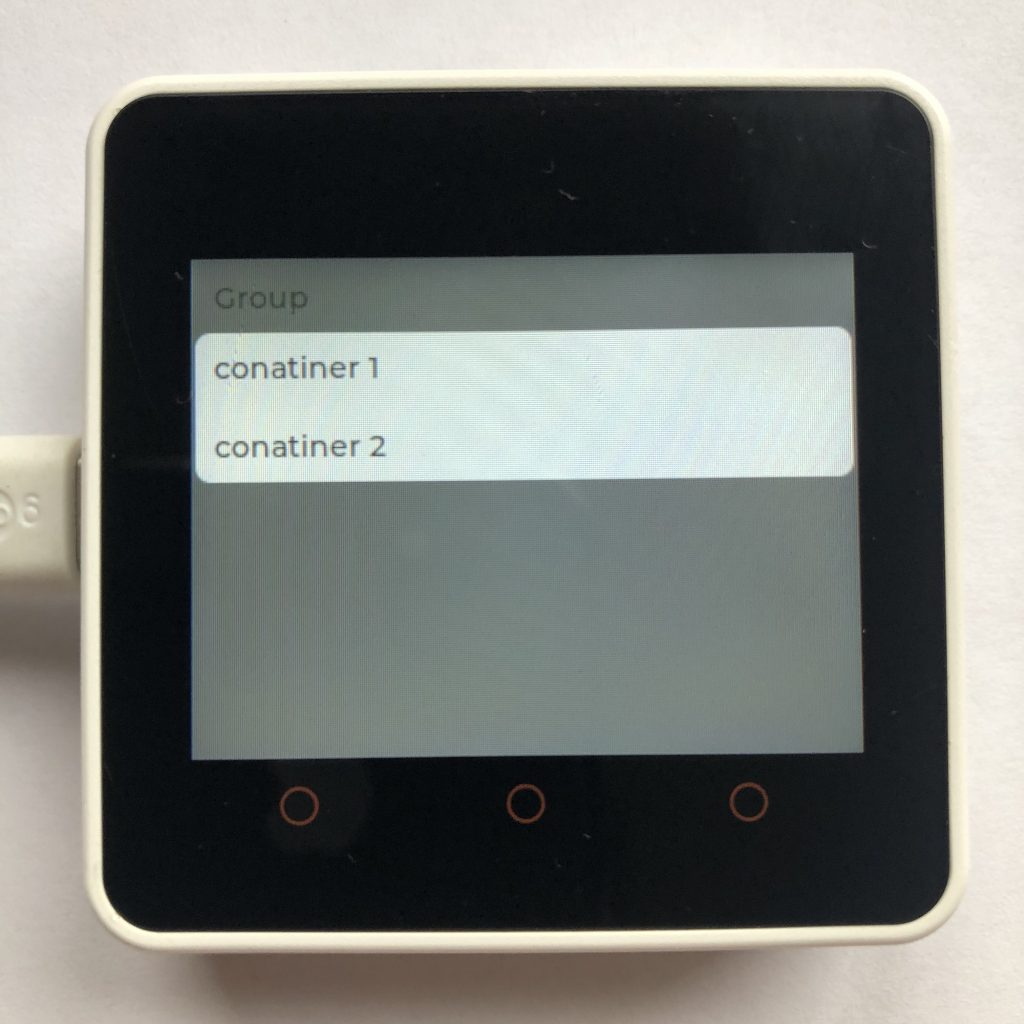
Menu Sections, Containers, and Separators
Menus have some functions for formatting parts of the screen. They are Sections, Containers, and Separators.
They seem to use the Flexbox layout which is a powerful tool, but can be tricky to use.
import lvgl
Menu = lvgl.menu( lvgl.scr_act() )
Menu.set_size( 320, 240 )
Menu.set_style_bg_color( lvgl.color_hex( 0x505050 ), 0 )
#create the page
Home_Page = lvgl.menu_page( Menu, None )
#create some normal text
cont = lvgl.menu_cont( Home_Page )
title = lvgl.label( cont )
title.set_text( 'Group' )
#create a section
section = lvgl.menu_section( Home_Page )
#create some text in the section
container1 = lvgl.menu_cont( section )
label1 = lvgl.label( container1 )
label1.set_text( 'conatiner 1' )
lvgl.menu_separator( section )
container2 = lvgl.menu_cont( section )
label2 = lvgl.label( container2 )
label2.set_text( 'conatiner 2' )
Menu.set_page( Home_Page )
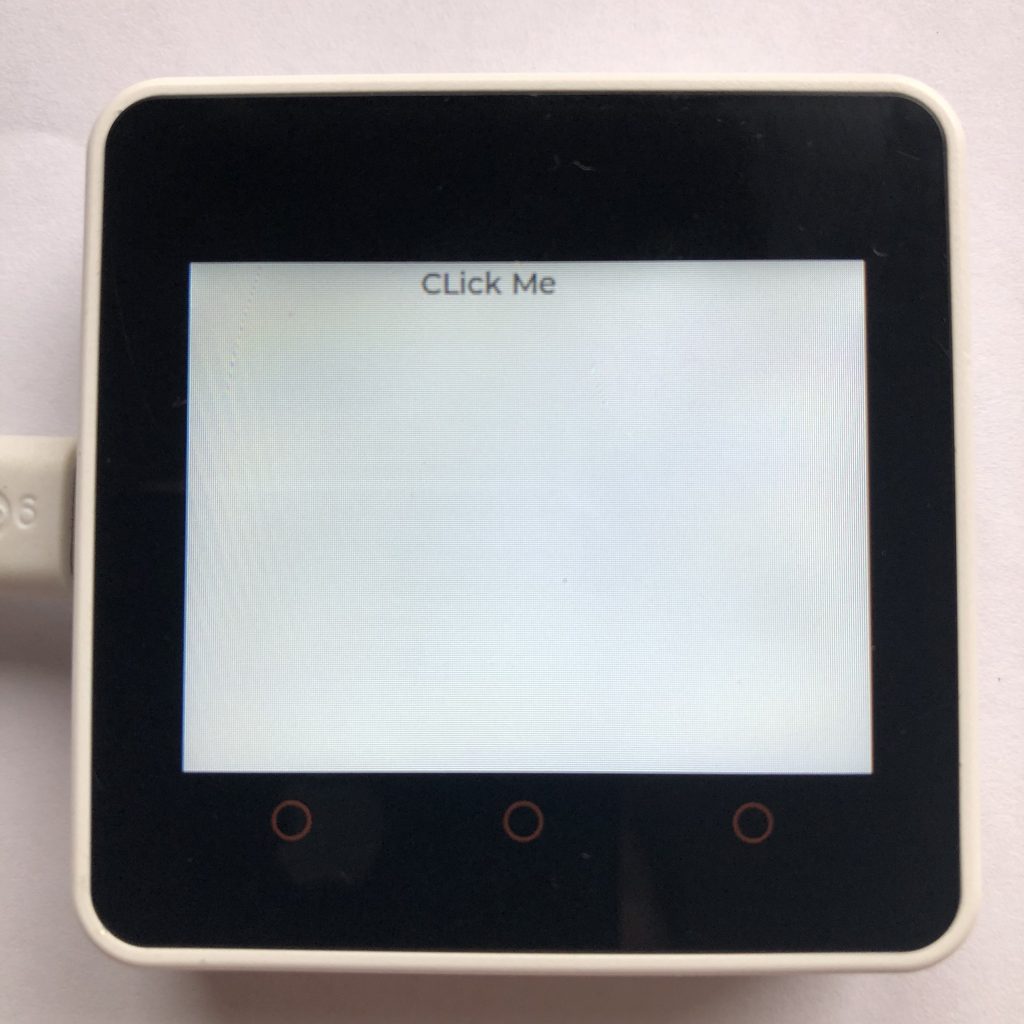
Changing Pages
The whole point of a menu is to show multiple pages. Here is the best way to change which page you show.
import lvgl
Menu = lvgl.menu( lvgl.scr_act() )
Menu.set_size( 320, 240 )
#create the main page
Main_Page = lvgl.menu_page( Menu, None )
label = lvgl.label( Main_Page )
label.set_text( 'CLick Me' )
label.set_size( 100, 50 )
#create another page
Other_Page = lvgl.menu_page( Menu, None )
label2 = lvgl.label( Other_Page )
label2.set_text( 'You found page two' )
#if text clicked load Other_Page
Menu.set_load_page_event( label, Other_Page )
#make Main_Page visable
Menu.set_page( Main_Page )
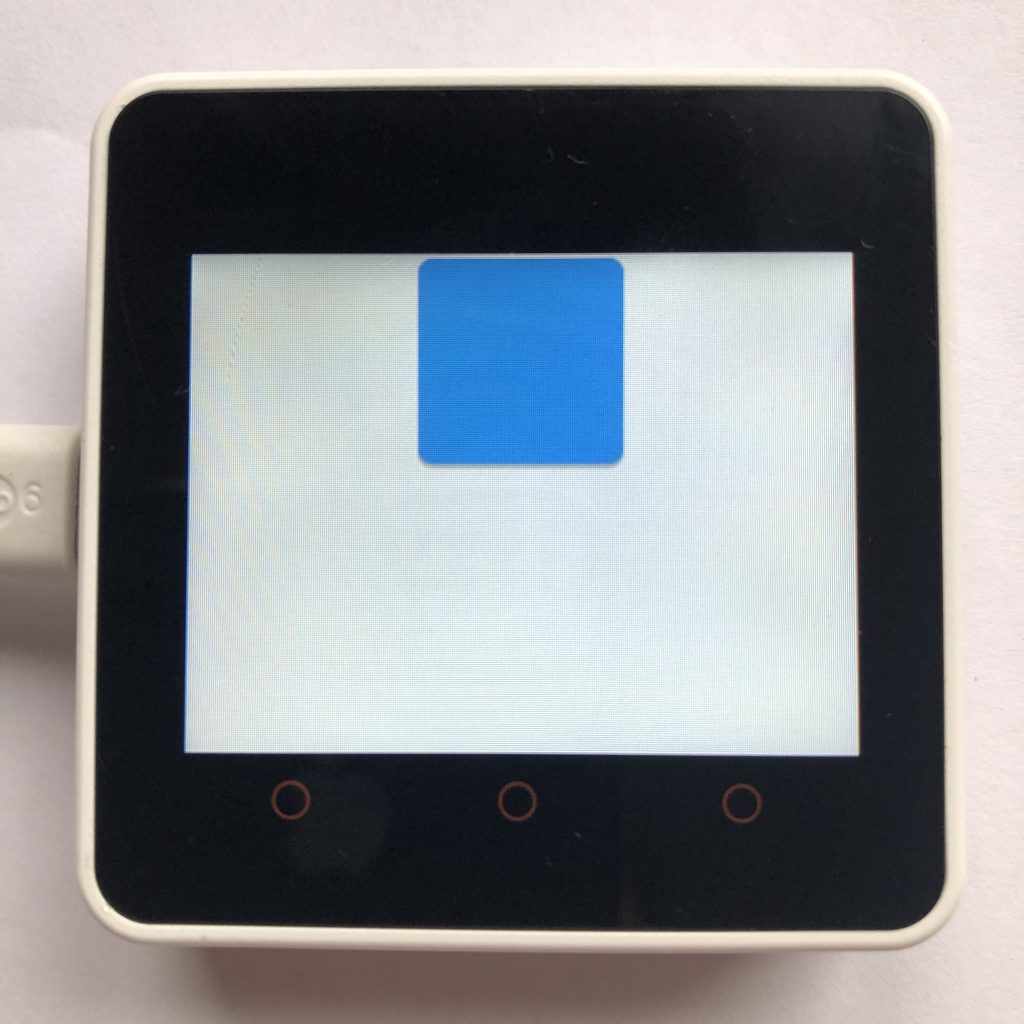
Loading Pages
Another way you can change the current page is to set it manually with the .set_page() function. This gets a little more complicated but gives you more choices.
import lvgl
Menu = lvgl.menu( lvgl.scr_act() )
Menu.set_size( 320, 240 )
#create destination page
Des_Page = lvgl.menu_page( Menu, None )
label2 = lvgl.label( Des_Page )
label2.set_text( 'you found page two' )
#create the main page
Main_Page = lvgl.menu_page( Menu, None )
btn = lvgl.btn( Main_Page )
btn.set_size( 100, 100 )
def handler( data ):
Menu.set_page( Des_Page )
btn.add_event_cb( handler, lvgl.EVENT.PRESSED, None )
#make Home_Page visable
Menu.set_page( Main_Page )
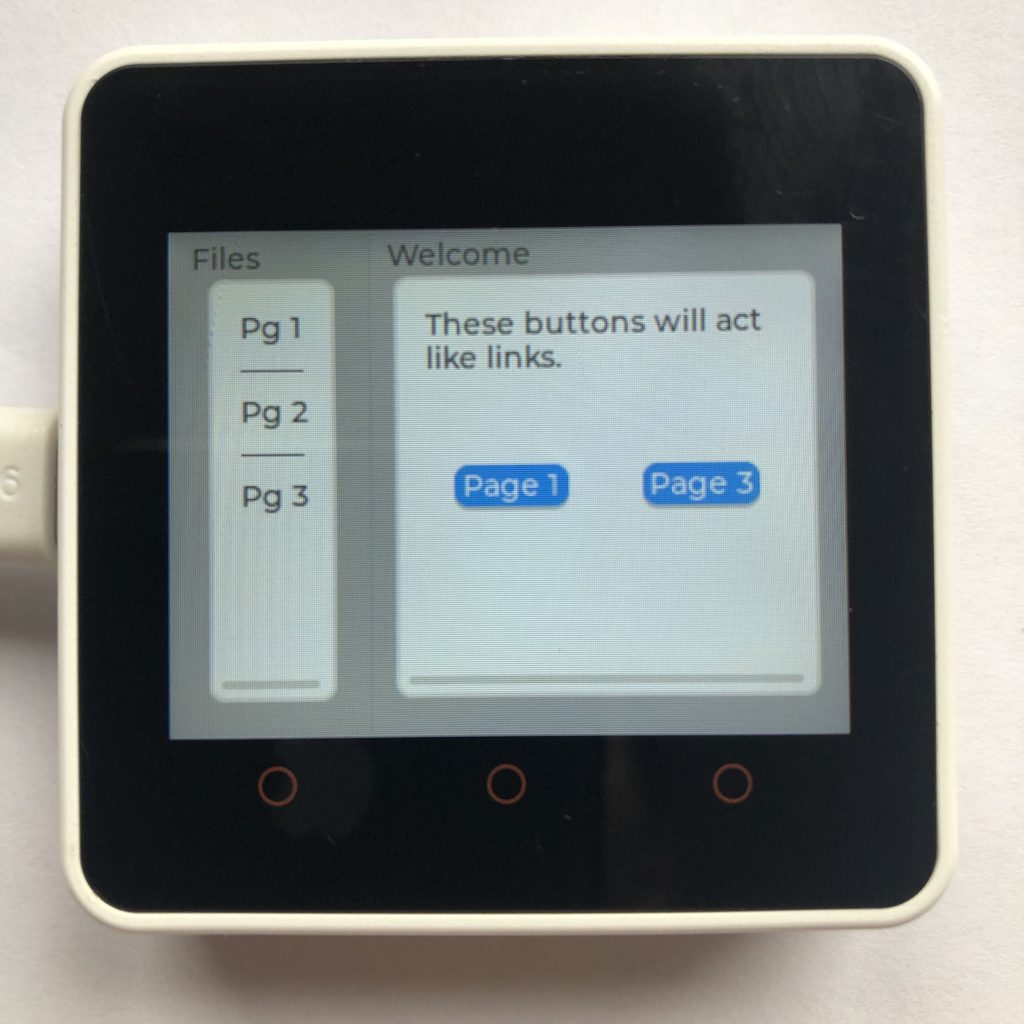
Simple Menu System
This is a simple menu system to give you an idea of what menus can do, but if you build one, I would suggest putting more into the design than I did.
import lvgl
Menu = lvgl.menu( lvgl.scr_act() )
Menu.set_size( 320, 240 )
Menu.set_style_bg_color( lvgl.color_hex( 0xA0A0A0 ), 0 )
#Main page
Main_pg = lvgl.menu_page( Menu, 'Welcome' )
#Main page content
section = lvgl.obj( Main_pg )
section.set_size( 200, 200 )
intro = lvgl.label( section )
intro.set_width( 172 )
intro.set_long_mode( lvgl.label.LONG.WRAP )
intro.set_flex_grow( 1 )
intro.set_text( 'These buttons will act like links.' )
link1 = lvgl.btn( section )
link1.set_size( 55, 20 )
link1.align( lvgl.ALIGN.CENTER, -45, 0 )
link1_label = lvgl.label( link1 )
link1_label.set_text( 'Page 1' )
link1_label.center()
link2 = lvgl.btn( section )
link2.set_size( 55, 20 )
link2.align( lvgl.ALIGN.CENTER, 45, 0)
link2_label = lvgl.label( link2 )
link2_label.set_text( 'Page 3' )
link2_label.center()
#Main page side bar
Main_Sidebar = lvgl.menu_page( Menu, 'Files' )
Sidebar_section = lvgl.obj( Main_Sidebar )
Sidebar_section.set_size( 60, 200 )
Page_link1 = lvgl.label( Sidebar_section )
Page_link1.set_text( 'Pg 1' )
seperator = lvgl.line( Sidebar_section )
pt = [{'x':0,'y': 28},{'x': 30, 'y': 28}]
seperator.set_points( pt, 2 )
Page_link2 = lvgl.label( Sidebar_section )
Page_link2.set_text( 'Pg 2' )
Page_link2.set_y( 40 )
seperator = lvgl.line(Sidebar_section)
pt = [{'x':0,'y': 68},{'x': 30, 'y': 68}]
seperator.set_points( pt,2 )
Page_link3 = lvgl.label( Sidebar_section )
Page_link3.set_text( 'Pg 3' )
Page_link3.set_y( 80 )
#End of main page
#Page 1
Page1 = lvgl.menu_page( Menu, None )
Page1_label = lvgl.label( Page1 )
Page1_label.set_text( 'This is page 1' )
#page2
Page2 = lvgl.menu_page( Menu, None )
Page2_label = lvgl.label( Page2 )
Page2_label.set_text( 'This is page 2' )
#page3
Page3 = lvgl.menu_page( Menu, None )
Page3_label = lvgl.label( Page3 )
Page3_label.set_text( 'This is page 3' )
#setup all the links
Menu.set_load_page_event( link1, Page1 )
Menu.set_load_page_event( link2, Page3 )
Menu.set_load_page_event( Page_link1, Page1 )
Menu.set_load_page_event( Page_link2, Page2 )
Menu.set_load_page_event( Page_link3, Page3 )
#start Main Page
Menu.set_page( Main_pg )
Menu.set_sidebar_page( Main_Sidebar )
In Conclusion
You should now have a idea of how to create menus in lvgl and some of the things you can do with them.
If you liked menus then micropython lvgl animation examples might interest you.