What are LVGL animations?
In code, if you want to change the size of some text or move a button across the screen, it will usually happen instantly. The button will teleport across the screen or the the label will suddenly be large.
LVGL animations allow you to make things look real. You can make a button slide across the screen or a label slowly grow bigger.
Getting a Screen to Work for These Examples
This is some simple code to get a ili9341 display to work with micropython lvgl. You will want to add it or your own screen code to the beginning of all the examples on this page.
Instead of putting it at the beginning of your code you could just put this code in the micropython boot file. It seems to work best there.
import lvgl
#this is the code that works for my screen:
#from ili9XXX import ili9341
#screen = ili9341(mosi=23, miso=38, clk=18, dc=15, cs=5, invert=True rot=0x10, width=320, height=240 )
#this is some generic code that might work better for you
from ili9XXX import ili9341
screen = ili9341(mosi=23, miso=38, clk=18, dc=15, cs=5,width=320, height=240)
#You will probably want some touch screen code
#here is what I use
from ft6x36 import ft6x36
touch = ft6x36()
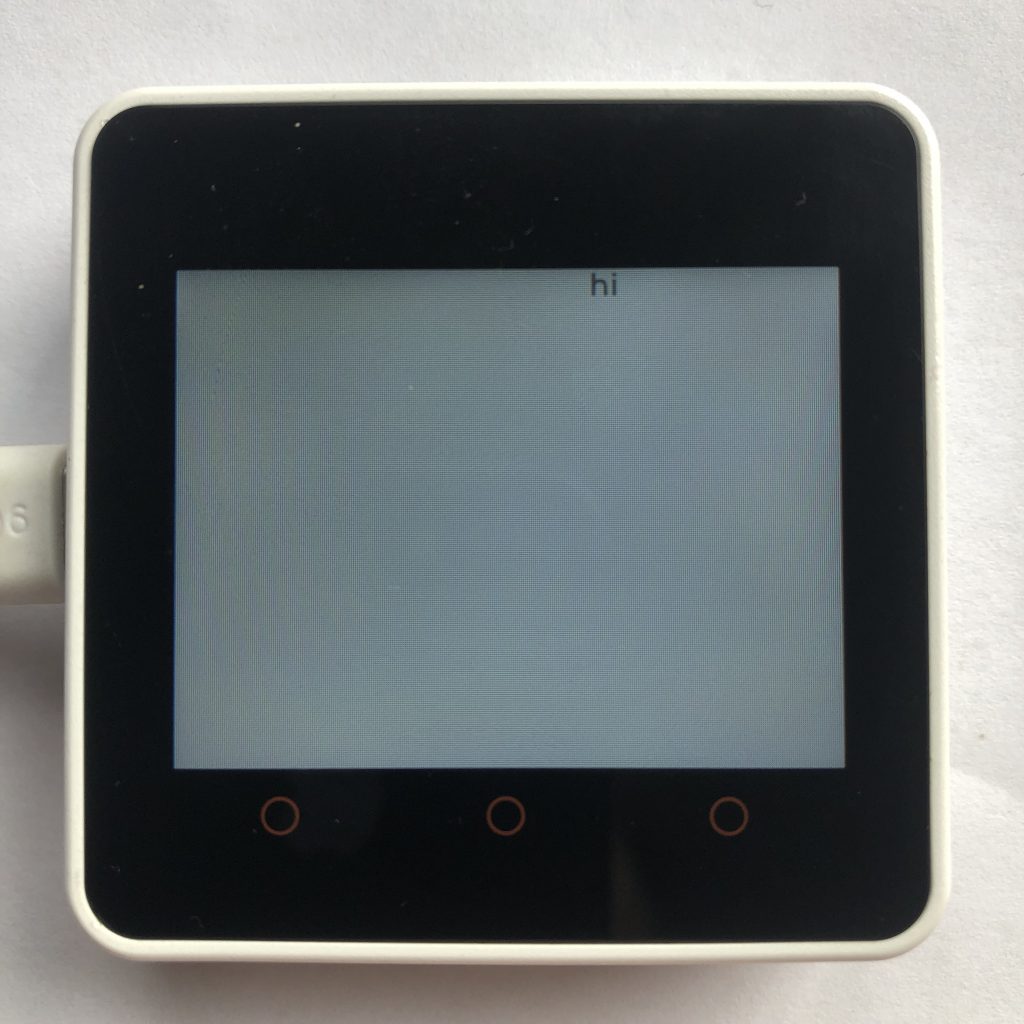
Creating an LVGL animation
This animation will move a label from 0x to 200x in 4 seconds (4000 milliseconds). This is the foundation of animations.
import lvgl
label = lvgl.label( lvgl.scr_act() )
label.set_text( 'hi' )
animation = lvgl.anim_t()
animation.init()
animation.set_var( label )
animation.set_time( 4000 )
animation.set_values( 0, 200 )
animation.set_custom_exec_cb( lambda not_used, value : label.set_x( value ))
animation.start()
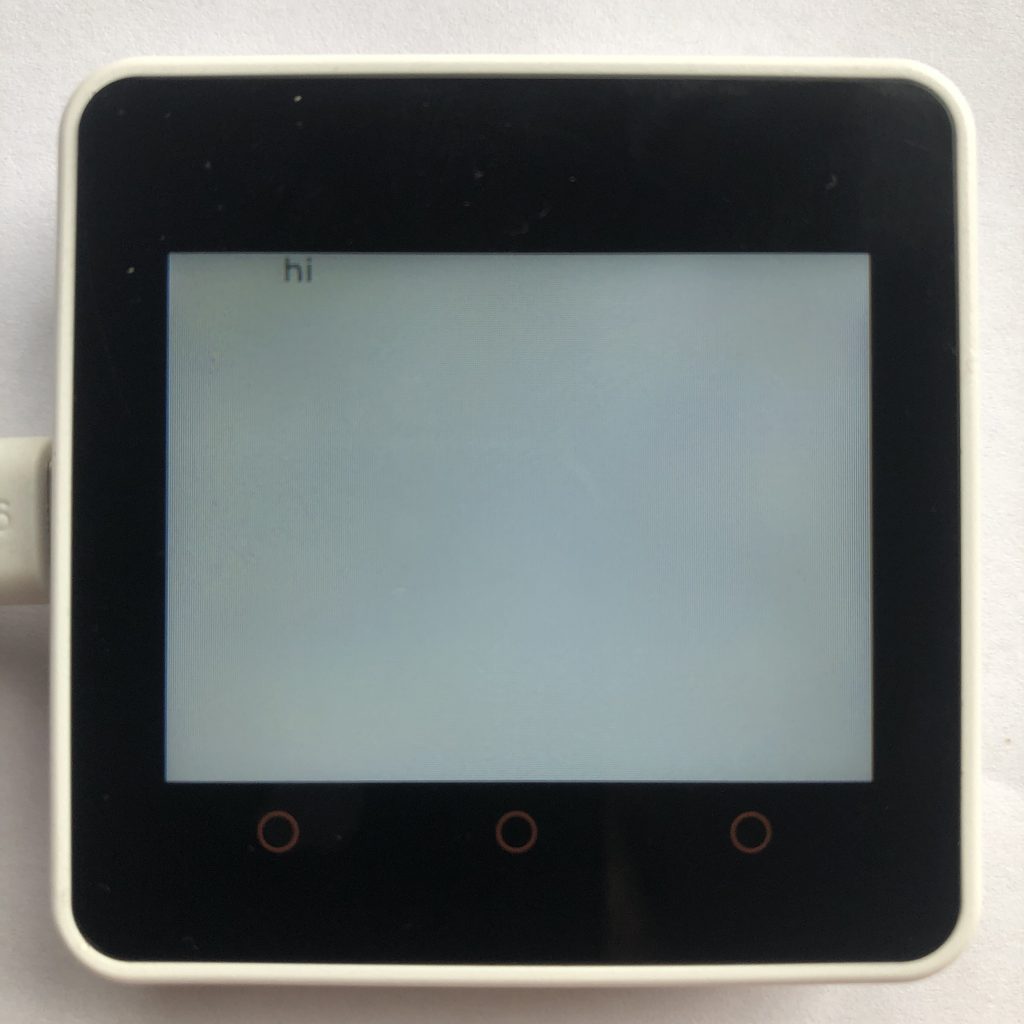
Animation controls
Animations have a lot of settings you can modify. These are the most important ones.
import lvgl
label = lvgl.label( lvgl.scr_act() )
label.set_text( 'hi' )
animation = lvgl.anim_t()
animation.init()
animation.set_var( label )
animation.set_values( 0, 100 )
animation.set_time( 1000 )
animation.set_custom_exec_cb( lambda not_used, value : label.set_x( value ))
#wait half a second before starting animation
animation.set_delay( 500 )
#play animation backward for 1 second after first play
animation.set_playback_time( 1000 )
#repeat animation infinitely
animation.set_repeat_count( lvgl.ANIM_REPEAT.INFINITE )
animation.set_repeat_delay( 500 )
animation.start()
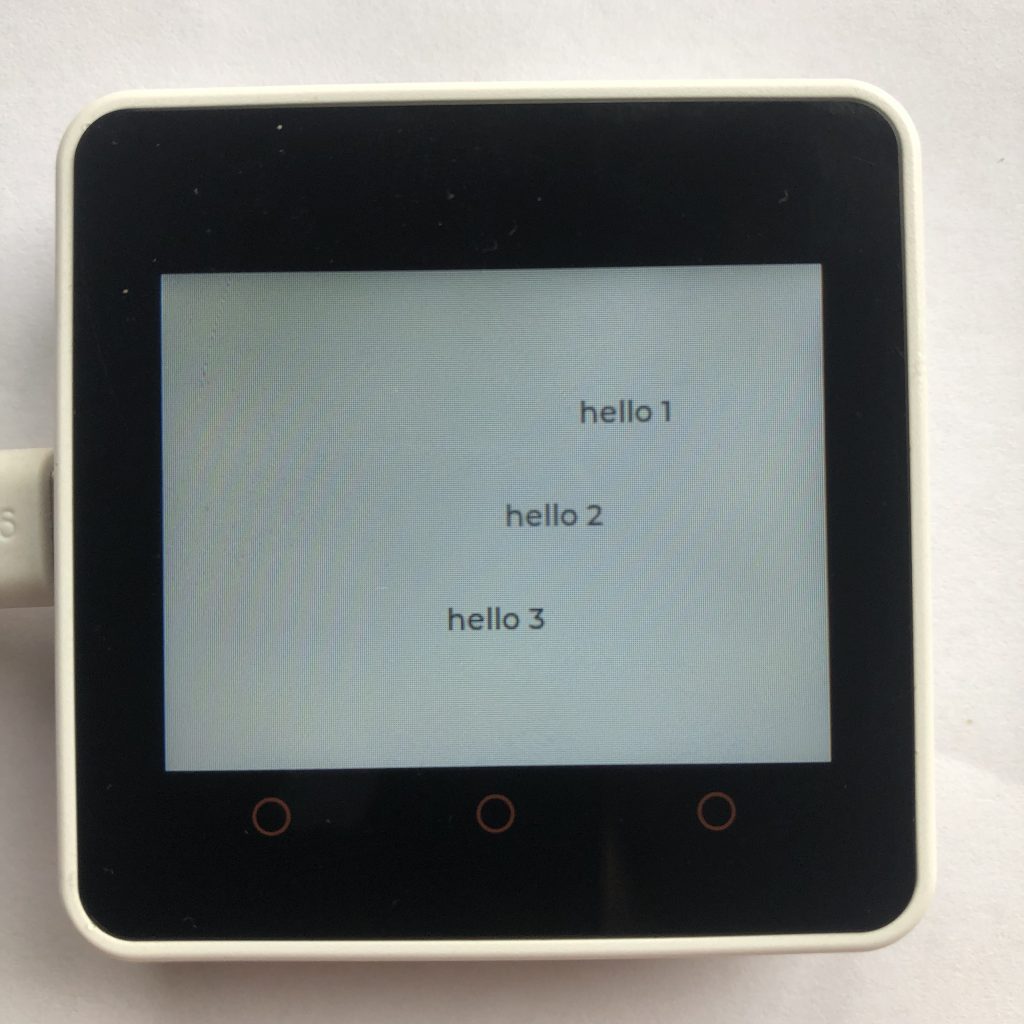
Using speed instead of time
If you wanted to, LVGL allows you to set the speed of an animations instead of the time of an animation. Sometimes this will simplify your code.
import lvgl
#create the slow short label
label1 = lvgl.label( lvgl.scr_act() )
label1.set_text( 'hello 1' )
label1.align( lvgl.ALIGN.CENTER, 0, -50 )
anim1 = lvgl.anim_t()
anim1.init()
anim1.set_var( label1 )
anim1.set_time( lvgl.anim_speed_to_time( 20, 0, 100 ))
anim1.set_values( 0, 100 )
anim1.set_repeat_count( lvgl.ANIM_REPEAT.INFINITE )
anim1.set_repeat_delay( 2000 )
anim1.set_custom_exec_cb( lambda not_used, value : label1.set_x( value ))
#create the fast long label
label2 = lvgl.label( lvgl.scr_act() )
label2.set_text('hello 2')
label2.align(lvgl.ALIGN.CENTER,-100,0)
anim2 = lvgl.anim_t()
anim2.init()
anim2.set_var( label2 )
anim2.set_time( lvgl.anim_speed_to_time( 40, -100, 100 ))
anim2.set_values( -100, 100 )
anim2.set_custom_exec_cb( lambda not_used, value : label2.set_x( value ))
anim2.set_repeat_count( lvgl.ANIM_REPEAT.INFINITE )
anim2.set_repeat_delay( 2000 )
#Create the fast short label
label3 = lvgl.label( lvgl.scr_act() )
label3.set_text( 'hello 3' )
label3.align( lvgl.ALIGN.CENTER, -100, 50 )
anim3 = lvgl.anim_t()
anim3.init()
anim3.set_var( label3 )
anim3.set_time( lvgl.anim_speed_to_time( 40, -100, 0 ))
anim3.set_values( -100, 0)
anim3.set_custom_exec_cb( lambda not_used, value : label3.set_x( value ))
anim3.set_repeat_count( lvgl.ANIM_REPEAT.INFINITE )
anim3.set_repeat_delay( lvgl.anim_speed_to_time( 40, -100, 0) + 2000 )
anim1.start()
anim2.start()
anim3.start()
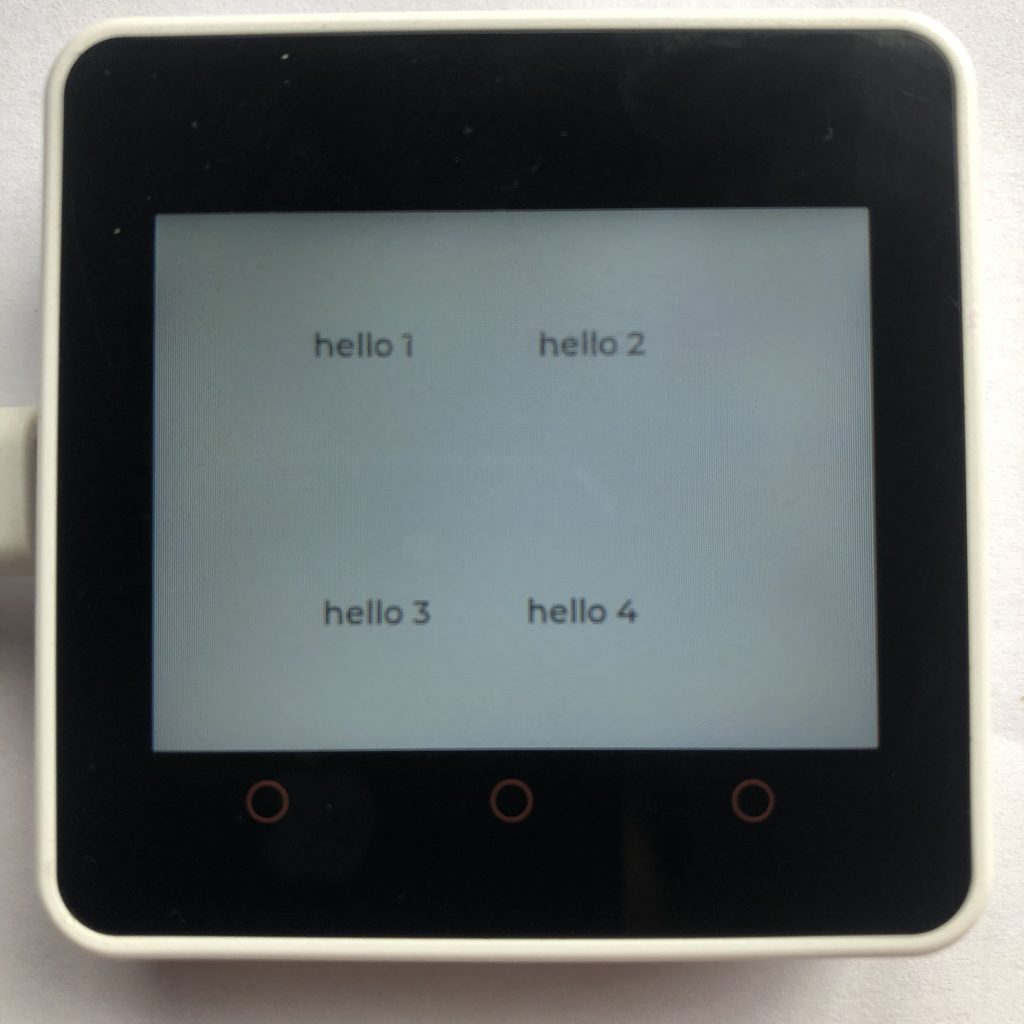
LVGL animation paths
Paths will make you animations look nicer. They modify your animation’s speed, like starting slow then speeding up.
This code shows three unique paths you can use.
import lvgl
#normal animation
label1 = lvgl.label( lvgl.scr_act() )
label1.set_text( 'hello 1' )
label1.align( lvgl.ALIGN.CENTER, -70, -60 )
anim1 = lvgl.anim_t()
anim1.init()
anim1.set_var( label1 )
anim1.set_time( 1000 )
anim1.set_values( -70, 20 )
anim1.set_repeat_count( lvgl.ANIM_REPEAT.INFINITE )
anim1.set_repeat_delay( 2000 )
anim1.set_custom_exec_cb( lambda not_used, value : label1.set_x( value ))
#this animation bounces the label when it ends
label2 = lvgl.label( lvgl.scr_act() )
label2.set_text( 'hello 2' )
label2.align( lvgl.ALIGN.CENTER, 30, -60 )
anim2 = lvgl.anim_t()
anim2.init()
anim2.set_var( label2 )
anim2.set_time( 1000 )
anim2.set_values( 30, 120 )
anim2.set_custom_exec_cb( lambda not_used, value : label2.set_x( value ))
anim2.set_repeat_count( lvgl.ANIM_REPEAT.INFINITE )
anim2.set_repeat_delay( 2000 )
anim2.set_path_cb( lvgl.anim_t.path_bounce )
#this animation goes past the end point then comes back
label3 = lvgl.label( lvgl.scr_act() )
label3.set_text( 'hello 3' )
label3.align( lvgl.ALIGN.CENTER, -70, 60 )
anim3 = lvgl.anim_t()
anim3.init()
anim3.set_var( label3 )
anim3.set_time( 1000 )
anim3.set_values( -70, 20 )
anim3.set_custom_exec_cb( lambda not_used, value : label3.set_x( value ))
anim3.set_repeat_count( lvgl.ANIM_REPEAT.INFINITE )
anim3.set_repeat_delay( 2000 )
anim3.set_path_cb( lvgl.anim_t.path_overshoot )
#this animation slowly starts and then slowly ends
label4 = lvgl.label( lvgl.scr_act() )
label4.set_text( 'hello 4' )
label4.align( lvgl.ALIGN.CENTER, 30, 60 )
anim4 = lvgl.anim_t()
anim4.init()
anim4.set_var( label4 )
anim4.set_time( 1000 )
anim4.set_values( 30, 120 )
anim4.set_custom_exec_cb( lambda not_used, value : label4.set_x( value ))
anim4.set_repeat_count( lvgl.ANIM_REPEAT.INFINITE )
anim4.set_repeat_delay( 2000 )
anim4.set_path_cb( lvgl.anim_t.path_ease_in_out )
anim1.start()
anim2.start()
anim3.start()
anim4.start()
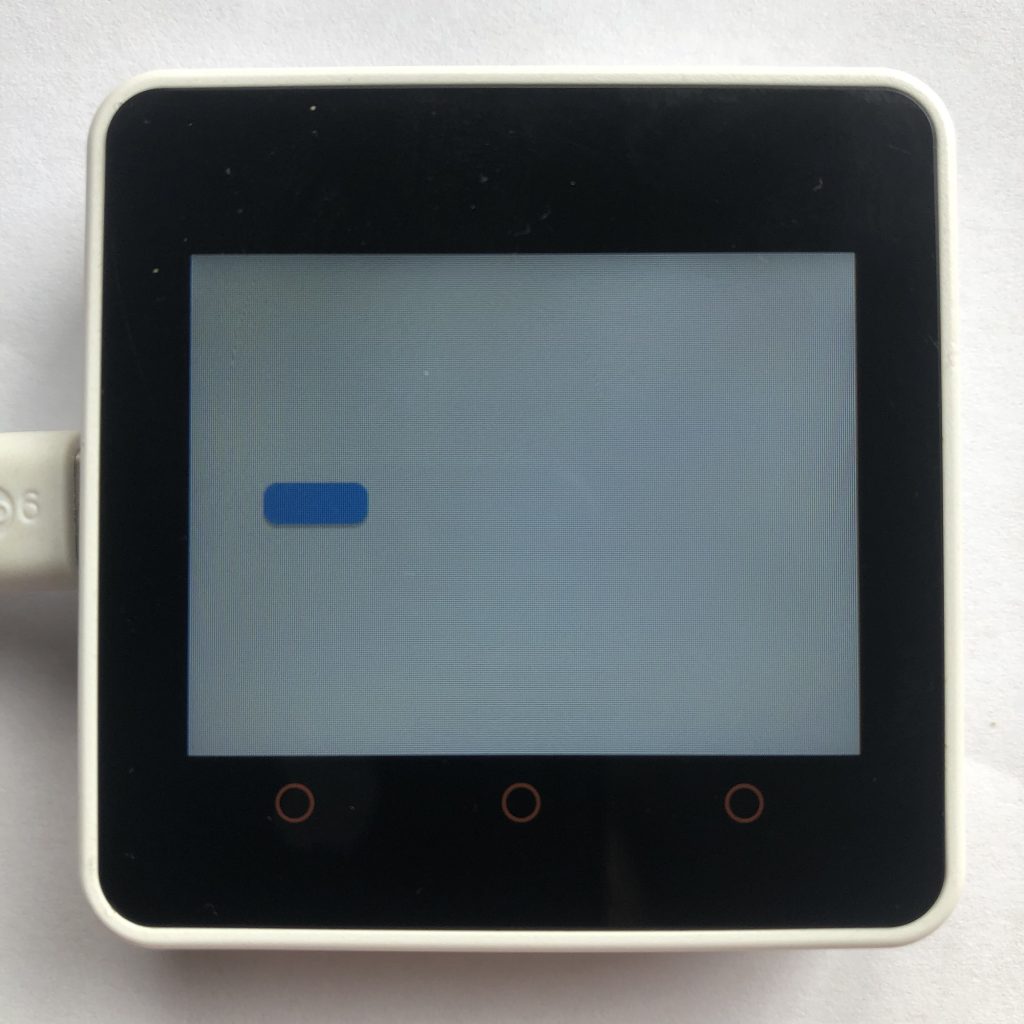
LVGL animation timelines
Timelines allow you to connect multiple animations together so controlling them is easier.
import lvgl
button = lvgl.btn( lvgl.scr_act() )
button.set_size( 50, 20 )
button.center()
anim1 = lvgl.anim_t()
anim1.init()
anim1.set_var( button )
anim1.set_time( 1000 )
anim1.set_values( -100, 100 )
anim1.set_custom_exec_cb( lambda not_used, value : button.set_x( value ))
anim2 = lvgl.anim_t()
anim2.init()
anim2.set_var( button )
anim2.set_time( 150 )
anim2.set_values( 100, 30 )
anim2.set_custom_exec_cb( lambda not_used, value : button.set_x( value ))
anim3 = lvgl.anim_t()
anim3.init()
anim3.set_var( button )
anim3.set_time( 2000 )
anim3.set_values( 30, -100 )
anim3.set_custom_exec_cb( lambda not_used, value : button.set_x( value ))
time = lvgl.anim_timeline_create()
lvgl.anim_timeline_add( time, 0, anim1 )
lvgl.anim_timeline_add( time, 1000, anim2 )
lvgl.anim_timeline_add( time, 1150, anim3 )
lvgl.anim_timeline_start( time )
Summery of Micropython LVGL animations
Animations are how you make your projects look nicer. They aren’t required for your project to work, but once you have got your project working they can take it to the next level.
If you liked animations you might also like micropython lvgl keyboard examples.