What Examples Are Given…
This page has six examples on how to use micropython lvgl. The first one is code to get some screens to work. The rest are examples on label color and lvgl inheritance.
Getting the Screen to Work
This is some simple code to get a ili9341 display to work with micropython lvgl. You will want to add it or your own screen code to the beginning of all the other examples on this page.
Instead of putting it at the beginning you could just put the code in the micropython boot file. It seems to work best there.
import lvgl
#this is the code that works for my screen:
#from ili9XXX import ili9341
#screen = ili9341(mosi=23, miso=38, clk=18, dc=15, cs=5, invert=True rot=0x10, width=320, height=240 )
# this is some generic code that might work better for you
from ili9XXX import ili9341
screen = ili9341(mosi=23, miso=38, clk=18, dc=15, cs=5,width=320, height=240)
#You will probably want some touch screen code
#here is what I use
from ft6x36 import ft6x36
touch = ft6x36()
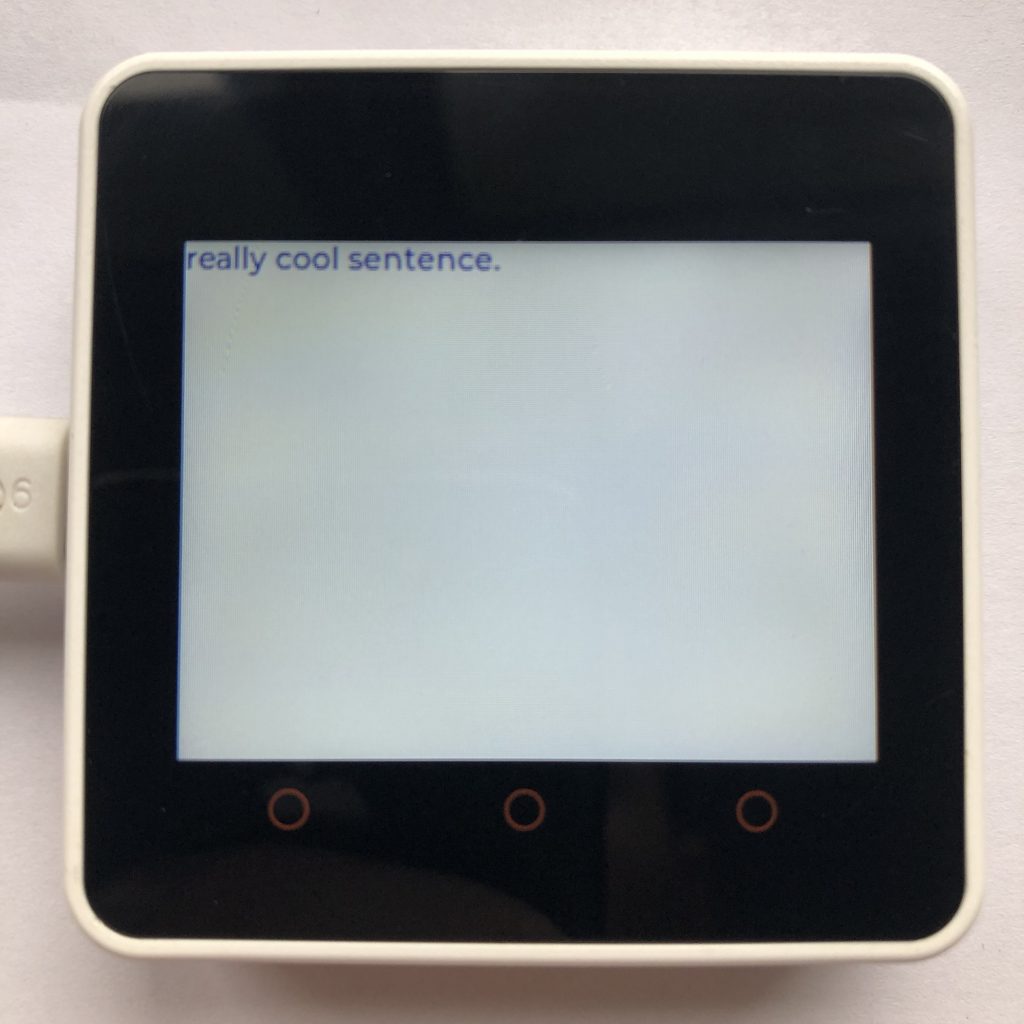
Changing Text Color
This code uses styles to change a label’s text color. If you want a better explanation here is a page on LVGL labels
import lvgl
label = lvgl.label(lvgl.scr_act())
label.set_text("really cool sentence.")
blueStyle = lvgl.style_t()
blueStyle.init()
blueStyle.set_text_color(lvgl.color_hex(0x00009c))
label.add_style(blueStyle,0)
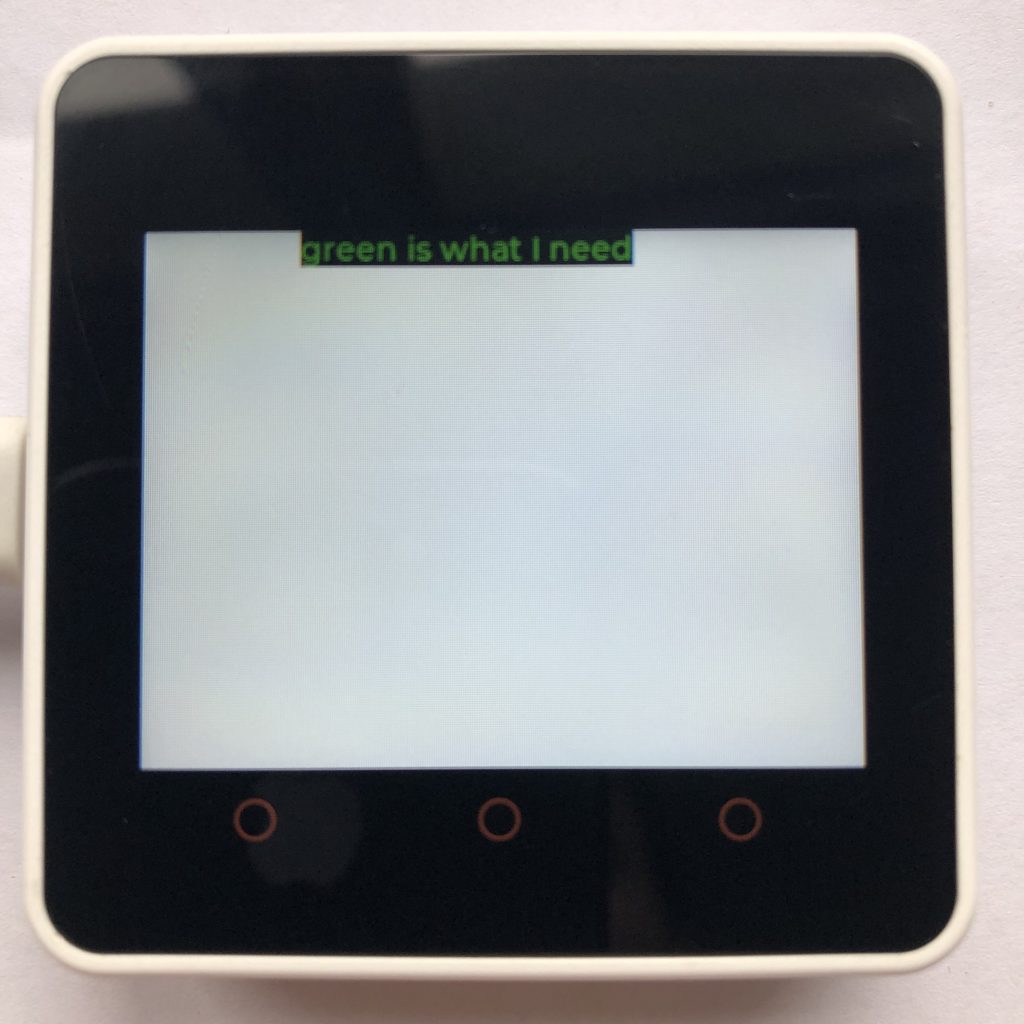
Highlighting Text
An example on changing the background color in lvgl.
import lvgl
highlight = lvgl.style_t()
highlight.init()
highlight.set_bg_opa(255)
#opacity (opa) is how visable the background is, 255 is completely visable and 0 is transparent
highlight.set_bg_color(lvgl.color_hex(0x000000))
highlight.set_text_color(lvgl.color_hex(0x009A00))
importantText = lvgl.label(lvgl.scr_act())
importantText.add_style(highlight, 0)
importantText.set_text("green is what I need")
importantText.set_pos(70,0)
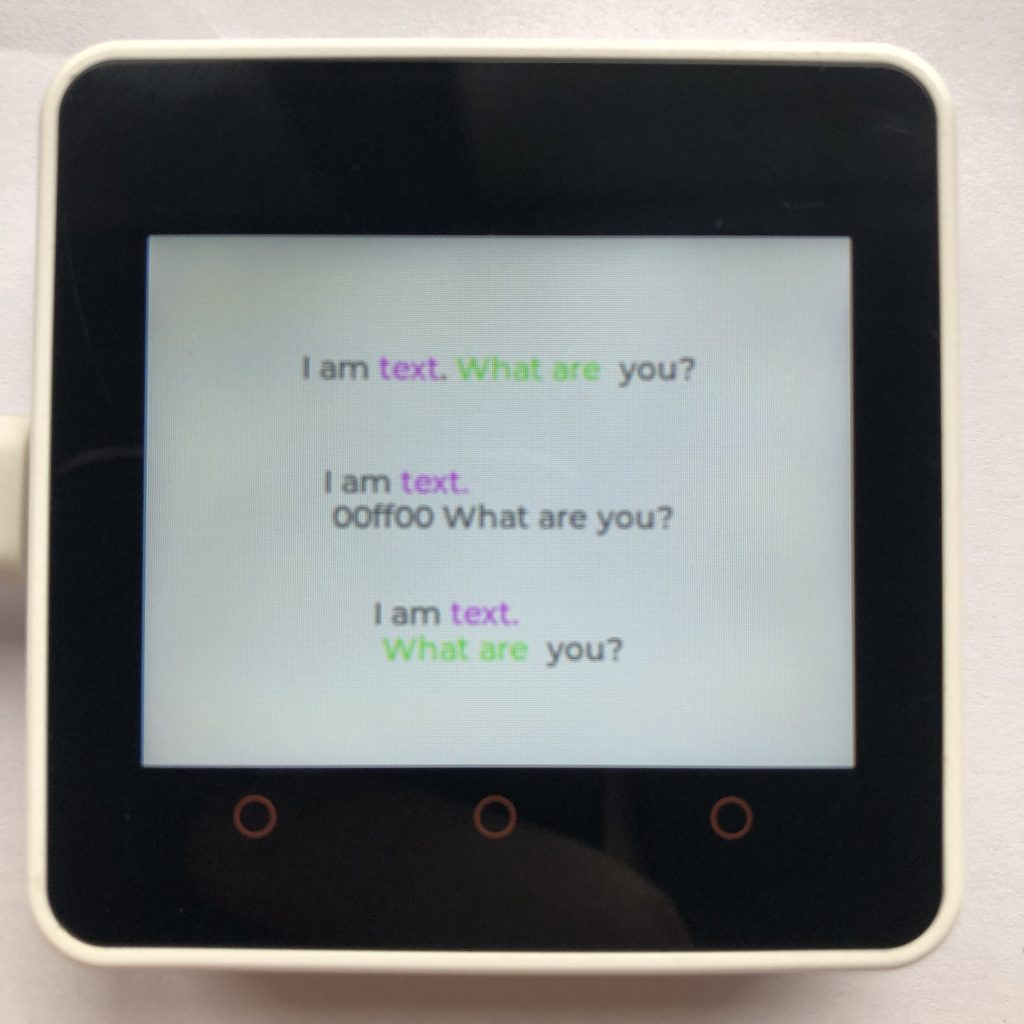
Coloring Sections of Text
Recoloring allows you to change the color of a small part of text.
import lvgl
# recoloring
text = lvgl.label(lvgl.scr_act())
text.set_recolor(True)
text.set_text('I am #ff00ff text#. #00ff00 What are # you?')
text.center()
text.set_y(-60)
# recoloring works only on one line
otherText = lvgl.label(lvgl.scr_act())
otherText.set_recolor(True)
otherText.set_text('I am #ff00ff text. \n # #00ff00 What are # you?')
otherText.center()
# but you can recolor both lines individually
lastText = lvgl.label(lvgl.scr_act())
lastText.set_recolor(True)
lastText.set_text('I am #ff00ff text. # \n #00ff00 What are # you?')
lastText.center()
lastText.set_y(60)
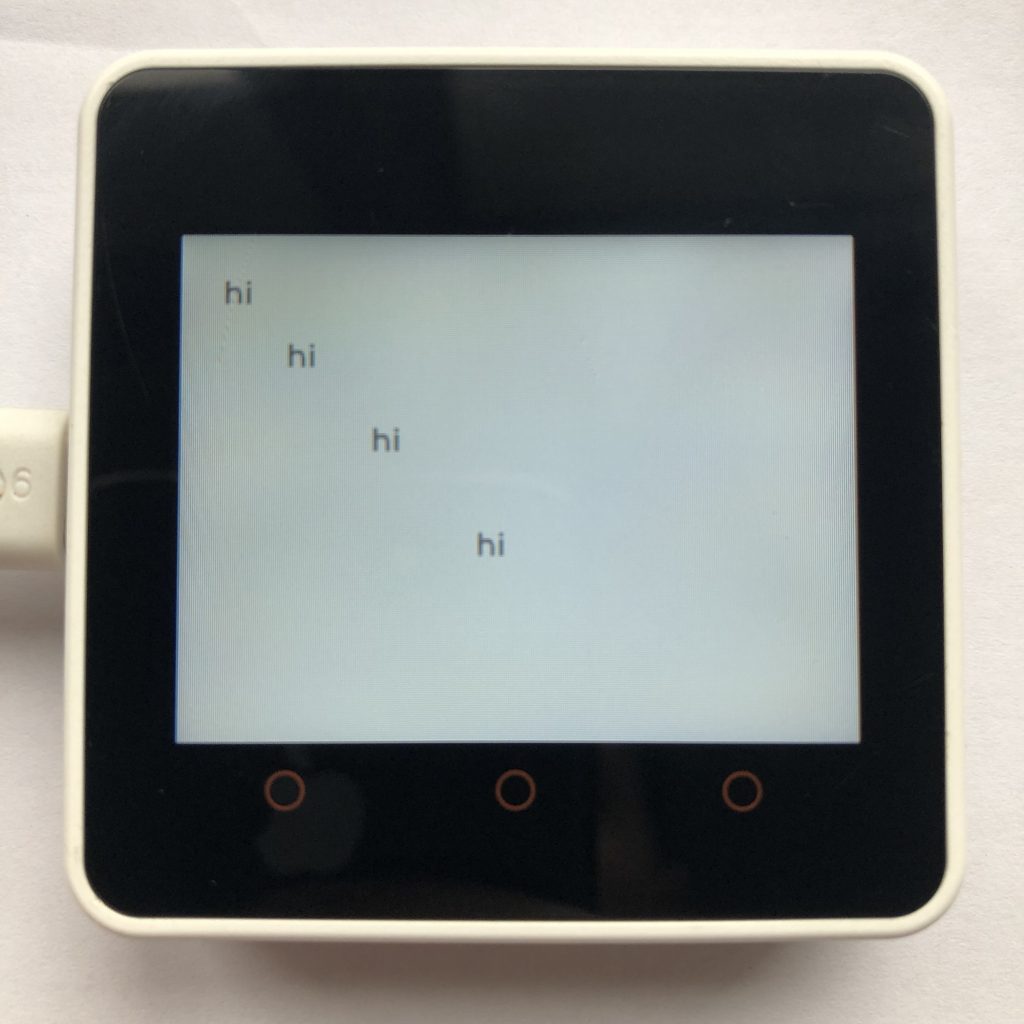
LVGL Posistion Inheritance
Lvgl uses inheritance, and it can make your program do weird things. So, it’s good to get familiar with it.
import lvgl
labelOne = lvgl.label(lvgl.scr_act())
labelOne.set_text('hi')
labelOne.set_x(20)
labelOne.set_y(20)
#first label with normal position x = 20 and y = 20
#second label inherits from first label which has x=20,y=20
labelTwo = lvgl.label(labelOne)
labelTwo.set_text('hi')
labelTwo.set_x(30)
labelTwo.set_y(30)
#labelTwo's actual positon is x=20+30,y=20+30 or x=50,y=50
#previous label's position was 50,50
#labelThree's position is 50+40,50+40
labelThree = lvgl.label(labelTwo)
labelThree.set_text('hi')
labelThree.set_x(40)
labelThree.set_y(40)
#previous label's position was 90,90
#labelThree's position is 90+50,90+50
labelFour = lvgl.label(labelThree)
labelFour.set_text('hi')
labelFour.set_x(50)
labelFour.set_y(50)
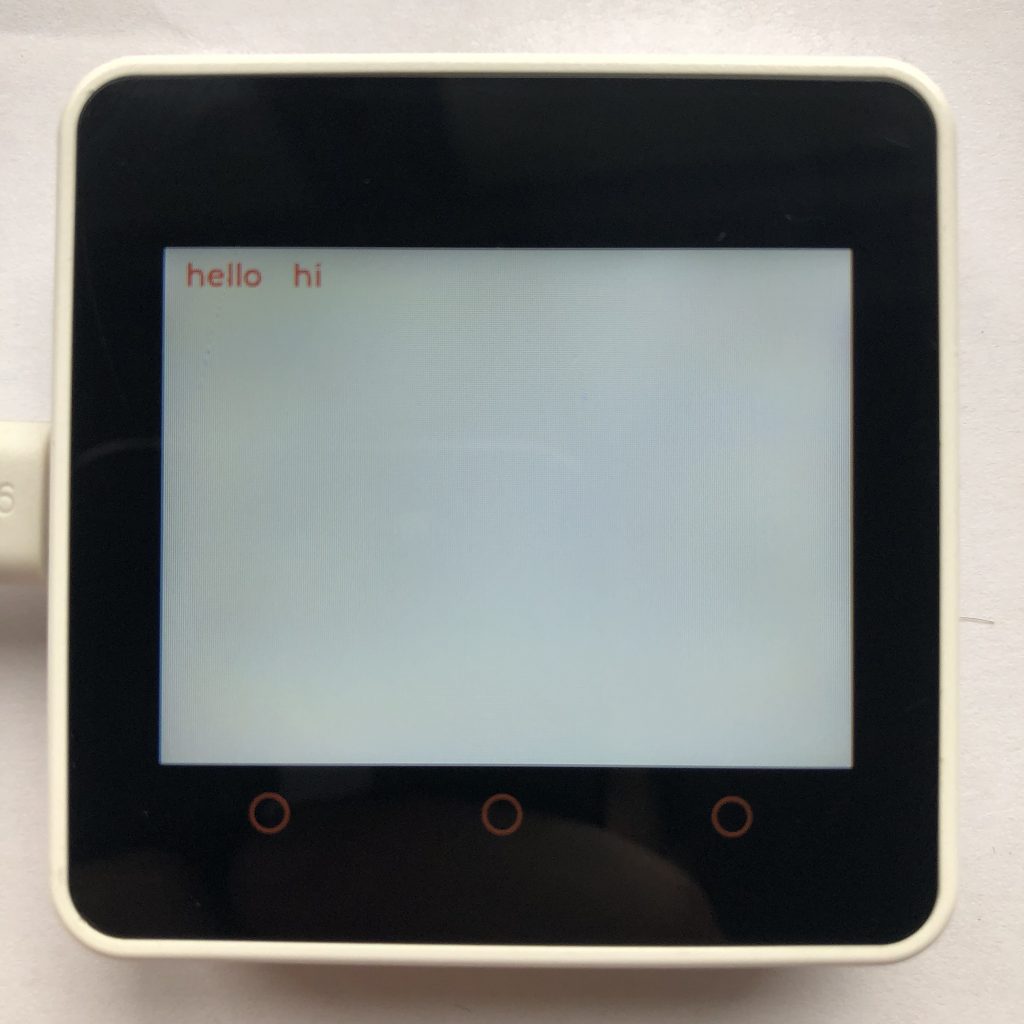
LVGL Style Inheritance
In lvgl objects can also inherit styles too. Here is what that looks like.
import lvgl
redStyle = lvgl.style_t()
redStyle.init()
redStyle.set_text_color(lvgl.color_hex(0xf50000))
labelOne = lvgl.label(lvgl.scr_act())
labelOne.set_text('hello')
labelOne.set_x(10)
labelOne.set_y(5)
labelOne.add_style(redStyle,0)
#because labelTwo inherits from labelOne it has the same style as labelOne
labelTwo = lvgl.label(labelOne)
labelTwo.set_text('hi')
labelTwo.set_x(50)
Wrapping Up
These examples should give you some practice with micropython lvgl and hopefully demystify coloring and inheritance for you.