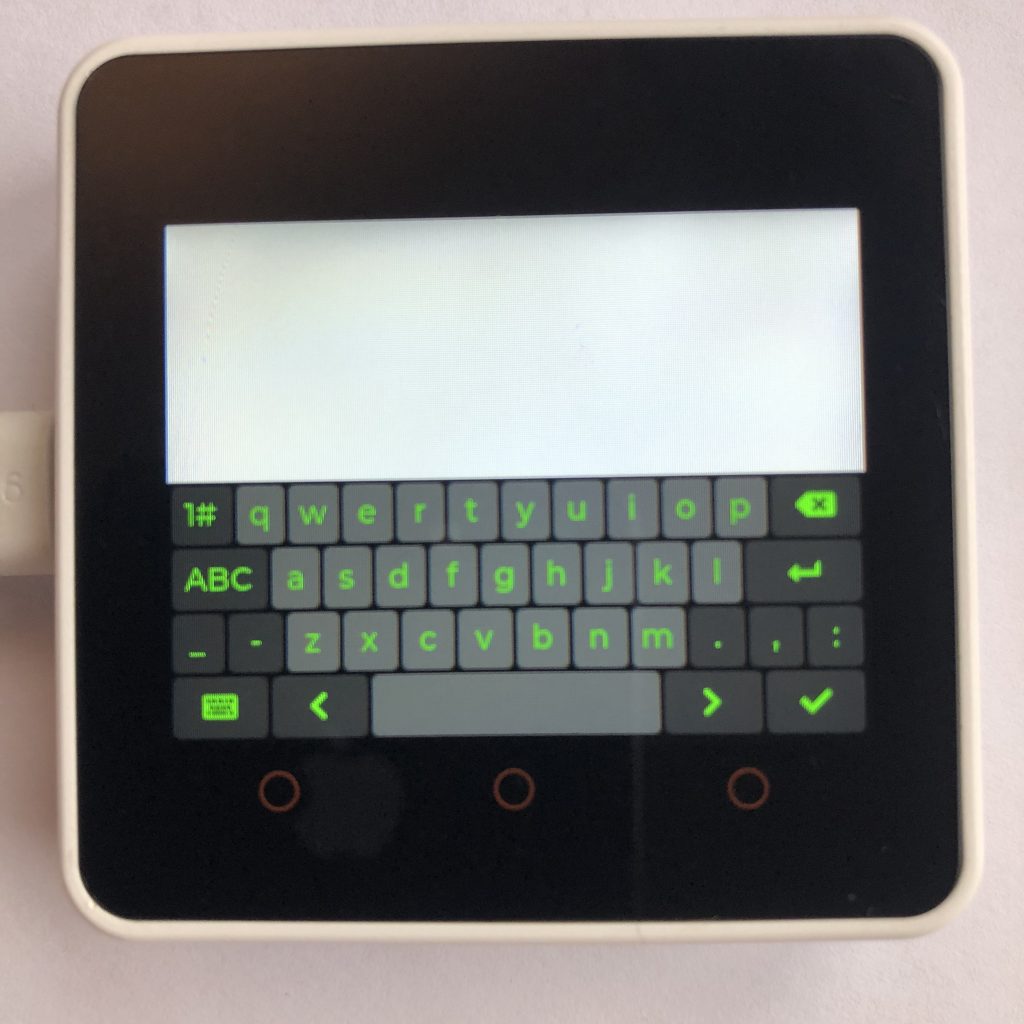
What are micropython lvgl keyboards?
Lvgl keyboards allow you to create a complete touchscreen keyboard with a few lines of code. This post will show you five things you can do with them.
Getting a Screen to Work In Micropython
This is some simple code to get a ili9341 display to work with micropython lvgl. You will want to add it or your own screen code to the beginning of all the examples on this page.
Instead of putting it at the beginning of your code you could just put this code in the micropython boot file. It seems to work best there.
import lvgl
#this is the code that works for my screen:
#from ili9XXX import ili9341
#screen = ili9341(mosi=23, miso=38, clk=18, dc=15, cs=5, invert=True rot=0x10, width=320, height=240 )
# this is some generic code that might work better for you
from ili9XXX import ili9341
screen = ili9341(mosi=23, miso=38, clk=18, dc=15, cs=5,width=320, height=240)
#You will want some touch screen code
#here is what I use
from ft6x36 import ft6x36
touch = ft6x36()
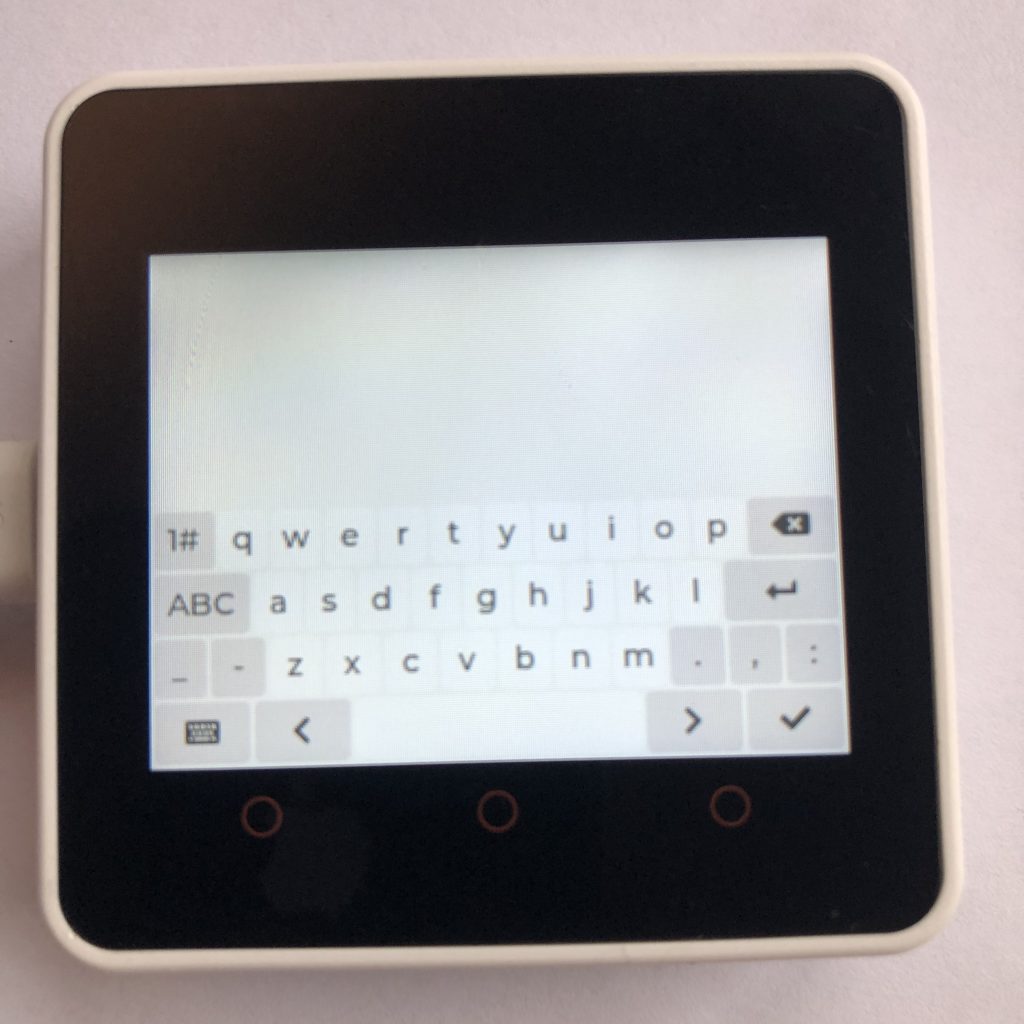
The basics of LVGL keyboards
This code will create a keyboard and print the buttons pressed on your shell screen.
import lvgl
#function to print key presses
def handler( data ):
tar = data.get_target()
btn = tar.get_selected_btn()
value = tar.get_btn_text( btn )
print( value )
#create keyboard and add the handler function
kyb = lvgl.keyboard( lvgl.scr_act() )
kyb.add_event_cb( handler, lvgl.EVENT.VALUE_CHANGED, None )
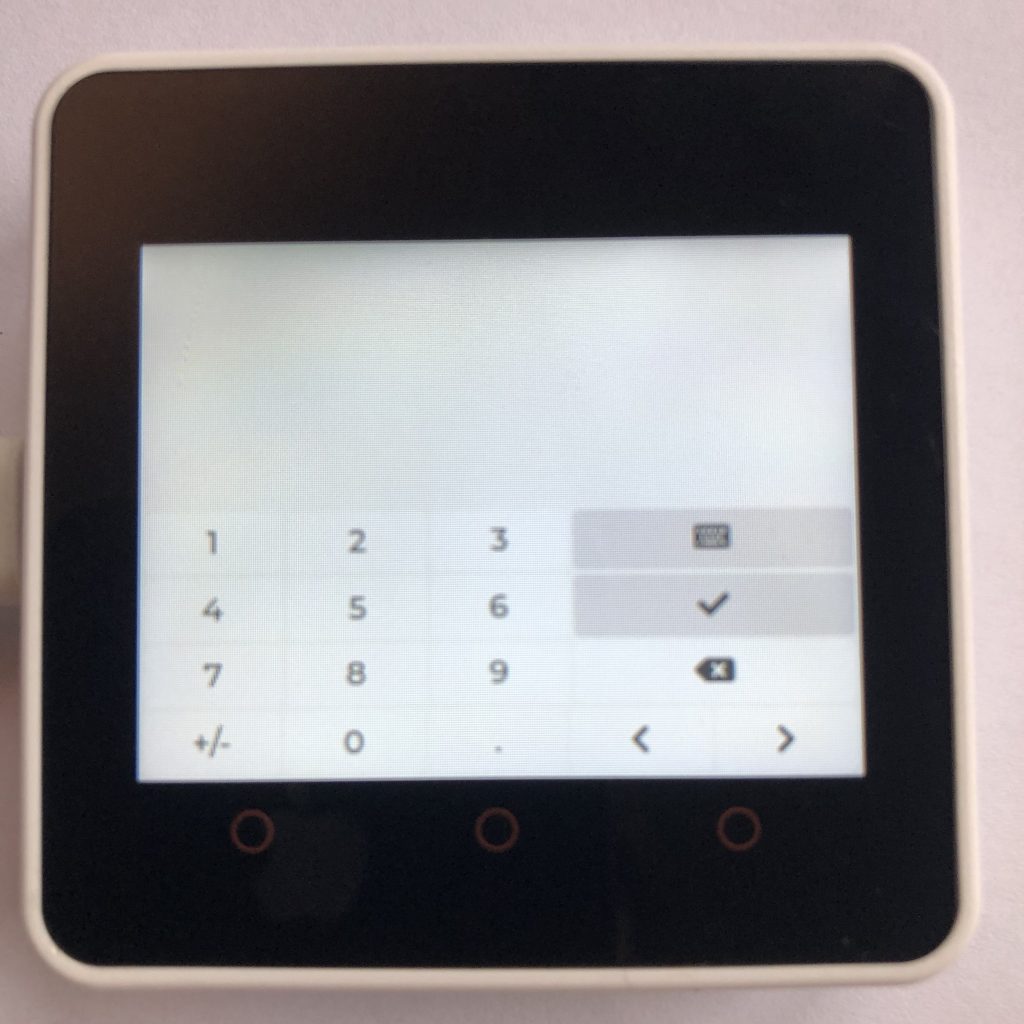
Having code control LVGL keyboards
This code will create a keyboard and then every second will switch between the different keyboard modes.
import lvgl
kyb = lvgl.keyboard( lvgl.scr_act() )
from time import sleep
while True:
kyb.set_mode( lvgl.keyboard.MODE.TEXT_LOWER )
sleep(1)
kyb.set_mode( lvgl.keyboard.MODE.TEXT_UPPER )
sleep(1)
kyb.set_mode( lvgl.keyboard.MODE.SPECIAL )
sleep(1)
kyb.set_mode( lvgl.keyboard.MODE.NUMBER )
sleep(1)
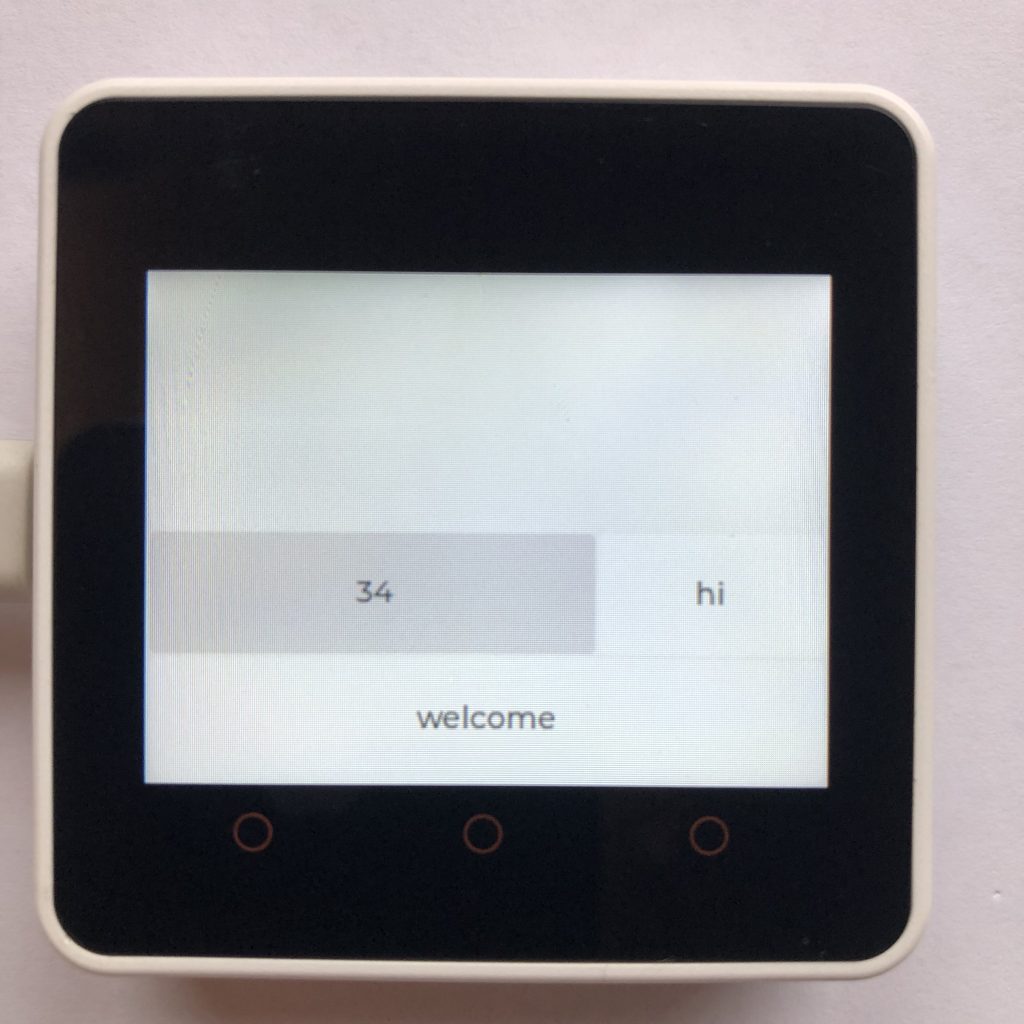
Creating custom keyboards
This code will create a custom lvgl keyboard and print the name of the buttons pressed in your shell.
import lvgl
#list of button texts
customMap = [ '34', 'hi', '\n', 'welcome', '']
#list of button sizes and settings
customCtrl = [ 2 | lvgl.btnmatrix.CTRL.CHECKABLE, lvgl.btnmatrix.CTRL.POPOVER, 1]
#function to print keypresses
def handler( data ):
tar = data.get_target()
btn = tar.get_selected_btn()
value = tar.get_btn_text( btn )
print( value )
kyb = lvgl.keyboard( lvgl.scr_act() )
kyb.add_event_cb( handler, lvgl.EVENT.VALUE_CHANGED, None )
#add key map were the lower case one used to be
kyb.set_map( lvgl.keyboard.MODE.TEXT_LOWER, customMap, customCtrl )
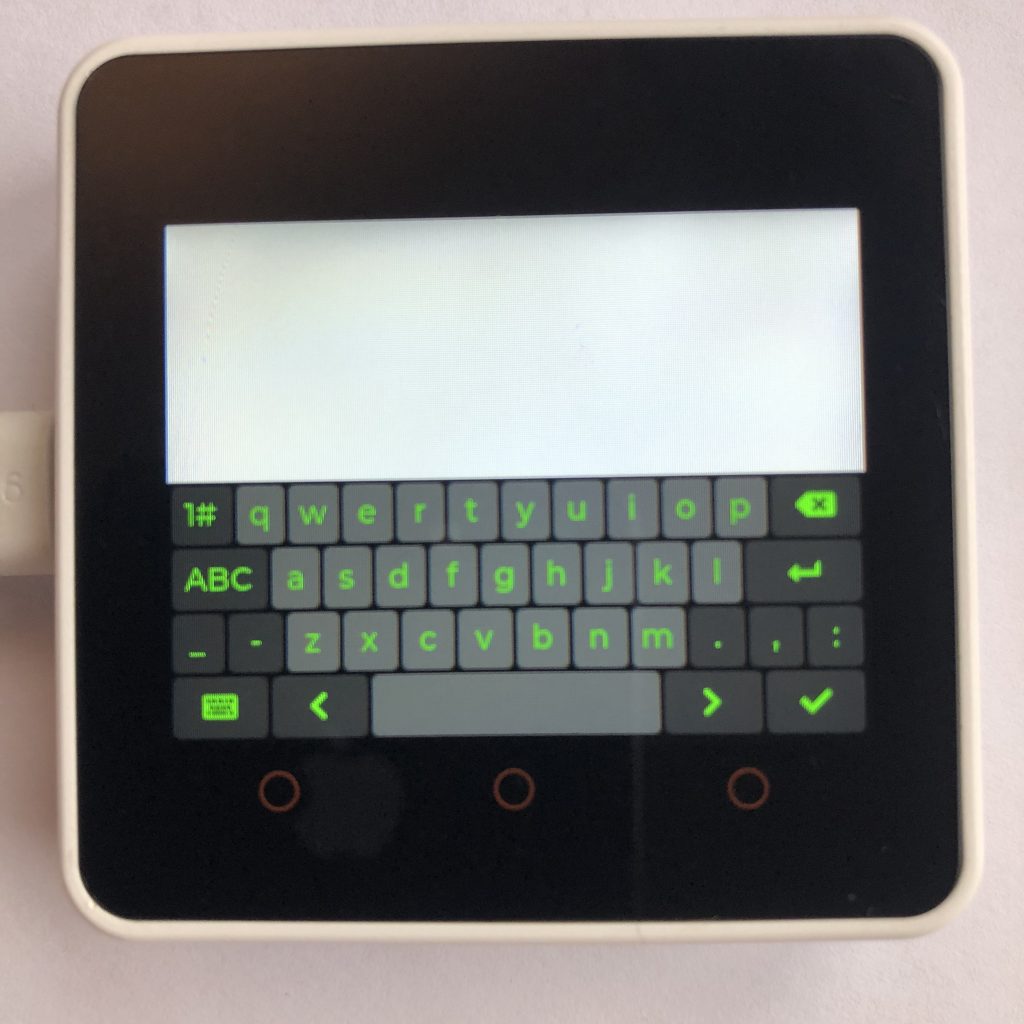
Styling LVGL keyboards
This code creates a keyboard and styles it. As usual, it will print the pressed button to your shell.
import lvgl
#function to print keypresses
def handler( data ):
tar = data.get_target()
btn = tar.get_selected_btn()
value = tar.get_btn_text( btn )
print( value )
#create black background
kyb_style = lvgl.style_t()
kyb_style.init()
kyb_style.set_bg_color( lvgl.color_hex( 0x000000 ))
#create normal button color
btn_style = lvgl.style_t()
btn_style.init()
btn_style.set_bg_color( lvgl.color_hex( 0x303030 ))
btn_style.set_text_color( lvgl.color_hex( 0x00DF00 ))
#create control button color
ctrl_btn_style = lvgl.style_t()
ctrl_btn_style.init()
ctrl_btn_style.set_bg_color( lvgl.color_hex( 0x101010 ))
ctrl_btn_style.set_text_color( lvgl.color_hex( 0x00FF00 ))
#create and style keyboard
kyb = lvgl.keyboard( lvgl.scr_act() )
kyb.add_event_cb( handler, lvgl.EVENT.VALUE_CHANGED, None )
kyb.add_style( kyb_style, 0 )
kyb.add_style( btn_style, lvgl.PART.ITEMS | lvgl.STATE.DEFAULT )
kyb.add_style( ctrl_btn_style, lvgl.PART.ITEMS | lvgl.STATE.CHECKED )
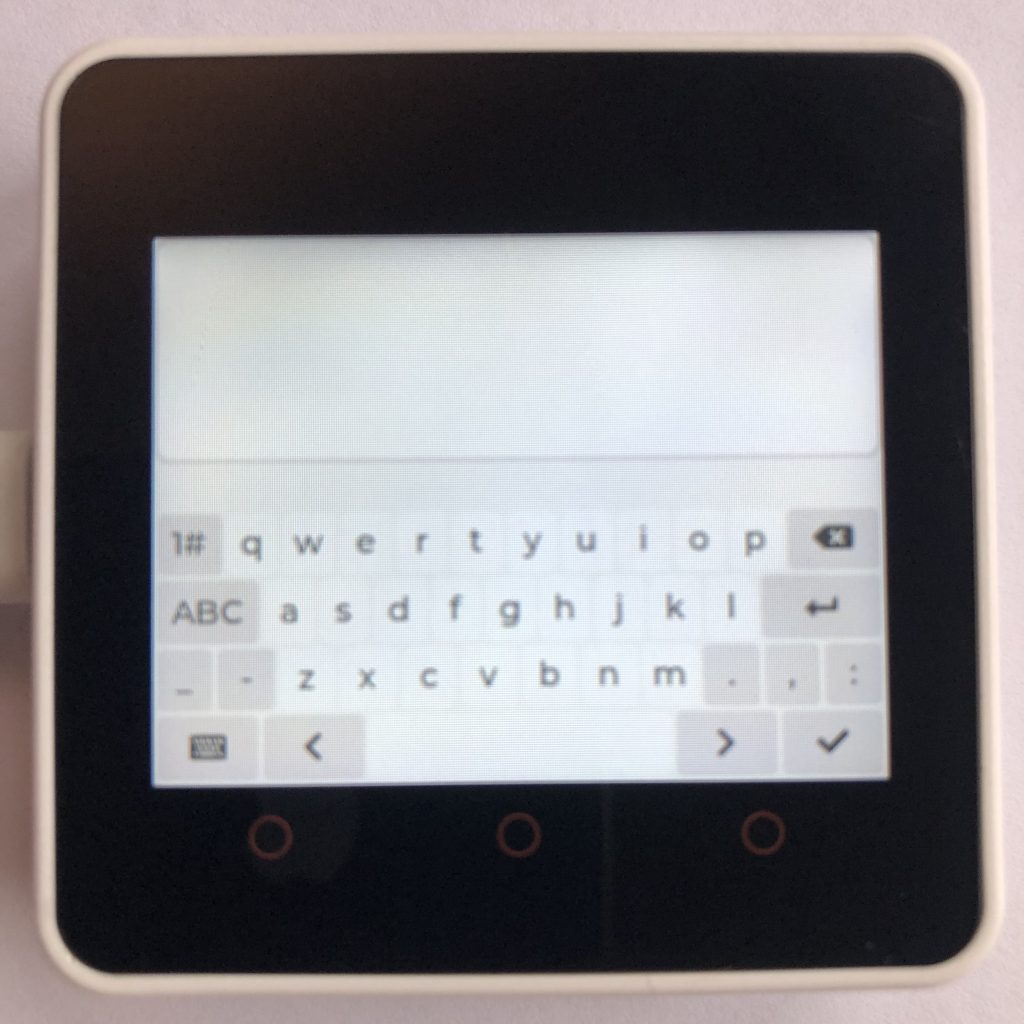
Complete lvgl keyboard example
This code will create a keyboard and textarea and connect them together. If you press the little keyboard button in the corner, the keyboard will disappear.
import lvgl
#the text area, its connect to the keyboard at the bottom
ta = lvgl.textarea( lvgl.scr_act() )
ta.set_size( 320, 100 )
ta.set_pos( 0, 0 )
#the button that makes the keyboard visable
btn = lvgl.btn( lvgl.scr_act() )
def visible( data ):
kyb.clear_flag( lvgl.obj.FLAG.HIDDEN )
btn.add_flag( lvgl.obj.FLAG.HIDDEN )
#the buttons text
label = lvgl.label( btn )
label.center()
label.set_style_text_color( lvgl.color_hex( 0x000000 ), 0 )
label.set_text( lvgl.SYMBOL.KEYBOARD )
btn.add_flag( lvgl.obj.FLAG.HIDDEN )
btn.set_style_bg_color( lvgl.color_hex( 0xCFCFCF ), 0 )
btn.add_event_cb( visible, lvgl.EVENT.CLICKED, None )
btn.set_size( 48, 31 )
btn.align( lvgl.ALIGN.BOTTOM_LEFT, 0, 0 )
#the keyboard
def handler( data ):
event = data.get_code()
key = data.get_target()
if event == lvgl.EVENT.READY:
print( ta.get_text() )
if event == lvgl.EVENT.CANCEL:
key.add_flag( lvgl.obj.FLAG.HIDDEN )
btn.clear_flag( lvgl.obj.FLAG.HIDDEN )
kyb = lvgl.keyboard( lvgl.scr_act() )
kyb.add_event_cb( handler, lvgl.EVENT.ALL, None )
kyb.set_popovers( True )
#connect the textarea to keyboard
kyb.set_textarea( ta )
Summery of LVGL keyboards
Keyboards have a lot of uses and are highly customizable. Best of all, they are not to complicated to work with. They can be a great addition to your projects.
If you liked keyboards you might like micropython lvgl chart examples.