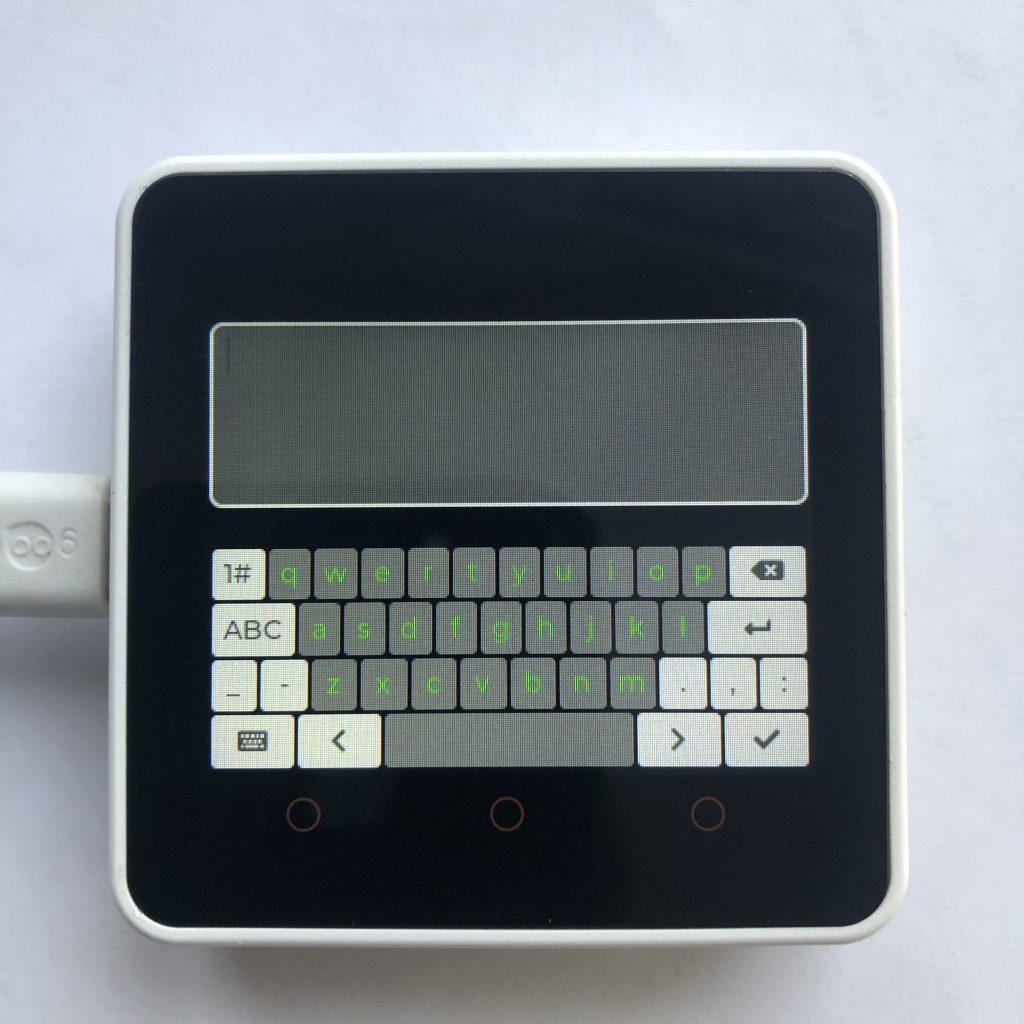
If you have ever worked with LVGL, you’ve probably wondered how to change the color of text or the background color of the screen. The way you do that in micropython lvgl is with styles.
When you are finished with this code tutorial today, you will be able to apply styles on the background to a keyboard and to a textarea, and you will now how to set styles to specific parts of an object.
To get this example to work, you will need to add your screen code to the beginning of the example. If you want more information try How to get a LVGL Micropython screen to work.
Here is the code:
import lvgl
ta_style = lvgl.style_t()
ta_style.init()
ta_style.set_bg_color(lvgl.color_hex(0x303030))
ta_style.set_text_color(lvgl.color_hex(0x00df00))
kb_style = lvgl.style_t()
kb_style.init()
kb_style.set_bg_color(lvgl.color_hex(0x000000))
btn_style = lvgl.style_t()
btn_style.init()
btn_style.set_bg_color( lvgl.color_hex( 0x505050 ))
btn_style.set_text_color( lvgl.color_hex( 0x00DF00 ))
obj_style = lvgl.style_t()
obj_style.init()
obj_style.set_bg_color(lvgl.color_hex(0x000000))
lvgl.scr_act().add_style(obj_style,0)
ta = lvgl.textarea( lvgl.scr_act() )
ta.set_size( 320, 100 )
ta.set_pos( 0, 0 )
ta.add_style(ta_style,0)
kyb = lvgl.keyboard( lvgl.scr_act() )
kyb.set_textarea(ta)
kyb.add_style(kb_style,lvgl.PART.MAIN | lvgl.STATE.DEFAULT)
kyb.add_style(btn_style,lvgl.PART.ITEMS | lvgl.STATE.DEFAULT )
How Does This Code Work?
Since you’ve seen the program above, why don’t we walk through the code and see how it works. This should make it clearer how you can modify this code for your own uses…
The Setup
First we have to import the LVGL library so we can use it.
import lvgl
The Textarea Style
To start, we will need a style for the textarea we are going to create later.
ta_style = lvgl.style_t()
Then we need to initialize it so it’s ready to use.
ta_style.init()
Next we want to set the background color to gray (aka. 0x303030).
ta_style.set_bg_color(lvgl.color_hex(0x303030))
Finally we will change the text color to green (aka. 0x00df00)
ta_style.set_text_color(lvgl.color_hex(0x00df00))
The Keyboard Style
We need to divide the keyboard style into two parts: the main keyboard style and the buttons style.
The Main Keyboard Style
To start we will create and initialize the style.
kb_style = lvgl.style_t()
kb_style.init()
Then we will set the keyboard background color to black.
kb_style.set_bg_color(lvgl.color_hex(0x000000))
The Button Style
As usual we create and initialize the new style.
btn_style = lvgl.style_t()
btn_style.init()
Finally we will set the styles background color to light gray (aka. 0x505050) and the text color to green (aka. 0x00DF00).
btn_style.set_bg_color( lvgl.color_hex( 0x505050 ))
btn_style.set_text_color( lvgl.color_hex( 0x00DF00 ))
The Background Style
To begin we will create the new style and initialize it.
obj_style = lvgl.style_t()
obj_style.init()
Then we will set the background color to black.
obj_style.set_bg_color(lvgl.color_hex(0x000000))
Connecting the Background Style Up
To actually use a style we need to add it to an object. The lvgl.scr_act() command gets the screen object and then we can add the obj_style to it.
lvgl.scr_act().add_style(obj_style,0)
Creating the Textarea
To start we will make a new textarea.
ta = lvgl.textarea( lvgl.scr_act() )
After that we will set its size to 320*240 pixels and set its position to 0,0.
ta.set_size( 320, 100 )
ta.set_pos( 0, 0 )
Finally we will need to connect the ta_style we created earlier to our textarea.
ta.add_style(ta_style,0)
Creating the Keyboard
First, we will generate a new keyboard.
kyb = lvgl.keyboard( lvgl.scr_act() )
Next, we will connect the textarea to the keyboard so that when you press a button, the textarea will display the pressed button.
kyb.set_textarea(ta)
Then the main keyboard style gets added. This is the one that sets the keyboards background color to black.
The lvgl.PART.MAIN tells the computer to put the style on the main part of the keyboard and the lvgl.STATE.DEFAULT makes it the default style. The bar( | ) tells the computer to run both of those commands together.
kyb.add_style(kb_style,lvgl.PART.MAIN | lvgl.STATE.DEFAULT)
After that, we will add another style to the keyboard buttons to change their color.
The lvgl.PART.ITEMS adds the style to the keyboards buttons.
kyb.add_style(btn_style,lvgl.PART.ITEMS | lvgl.STATE.DEFAULT )
What Happens Next
Today you have created a keyboard and textarea and styled them with different colors. Styles can also be used to add borders, shadows, opacity, etc. You can find a complete list at the LVGL docs.
The best way to learn code is to experiment. You could try adding other styles properties to the code above or just changing the color of the ones that already exist.
If you would like some more examples on textareas, you could try Micropython LVGL Textarea Examples, and for keyboards, Micropython LVGL Keyboard Examples might be helpful.