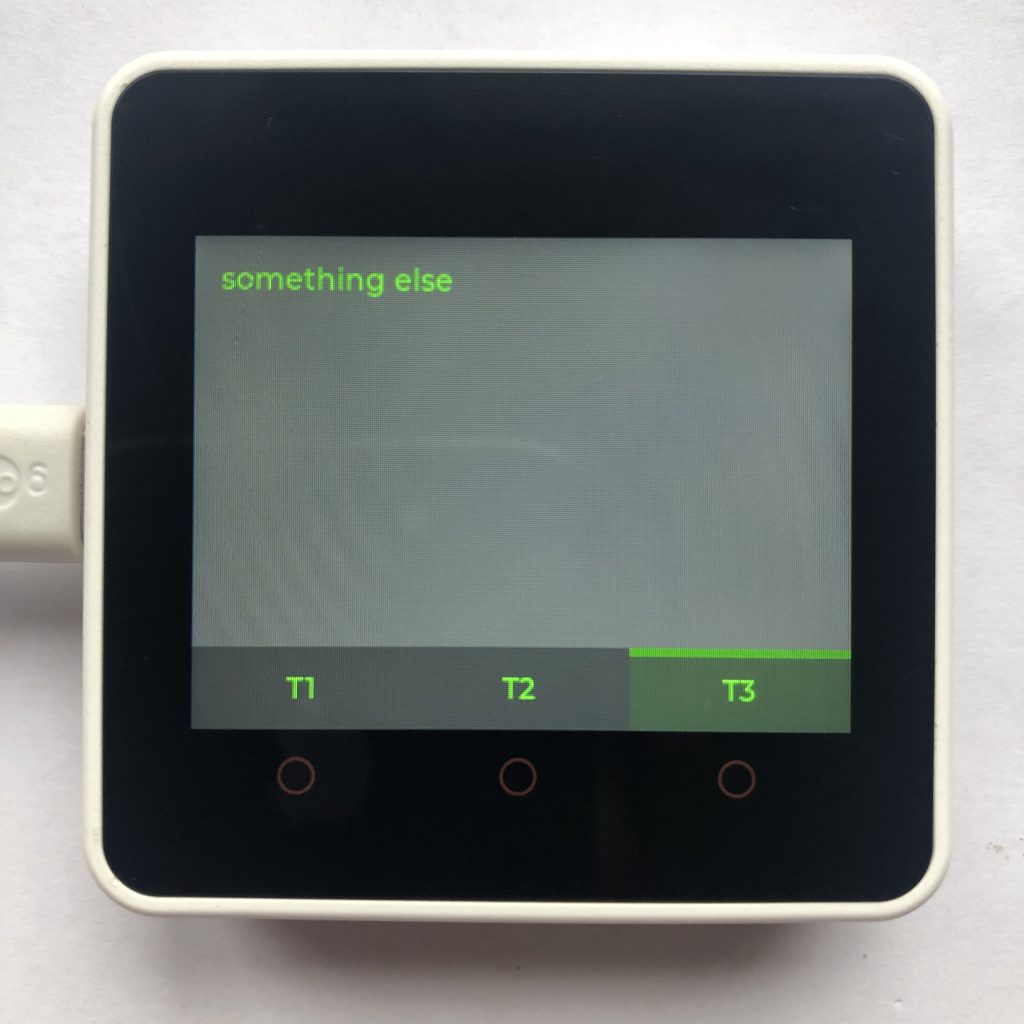
What are LVGL tabviews?
Tabviews allow you to have multiple pages and create buttons on the screen that move between the pages. Tabviews often allow you to swipe between pages too, instead of just pressing a button.
How to use these examples
To get these examples to work you will need to add some screen code to the beginning of each example. If you want more information try How to get a LVGL Micropython screen to work.
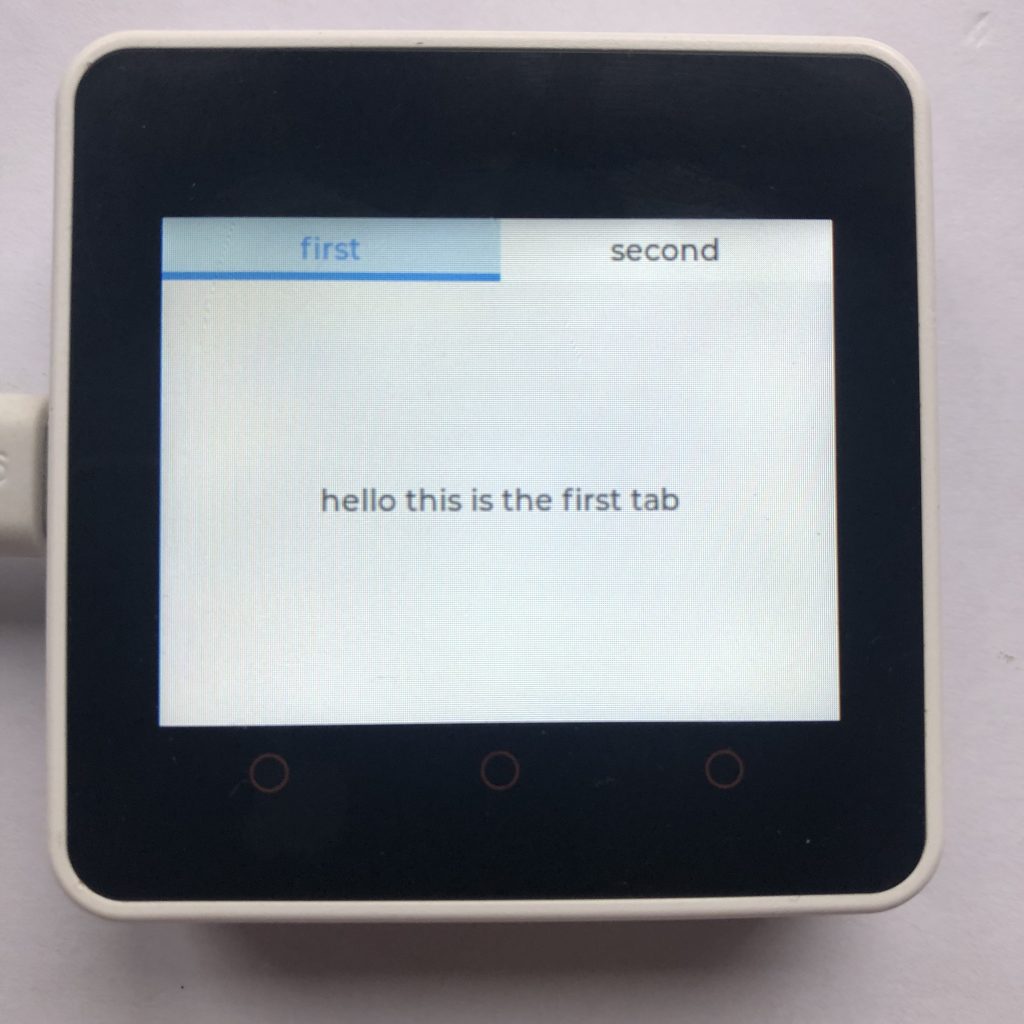
The Basic Example
This code will create a tabview with two pages, and place the tabs on the top of the screen.
import lvgl
tabview = lvgl.tabview( lvgl.scr_act(), lvgl.DIR.TOP, 30 )
tabview.set_size( 320, 240 )
tabview.center()
firstTab = tabview.add_tab( 'first' )
firstLabel = lvgl.label( firstTab )
firstLabel.set_text( 'hello this is the first tab' )
firstLabel.center()
secondTab = tabview.add_tab( 'second' )
secondLabel = lvgl.label( secondTab )
secondLabel.set_text( 'somthing really interesting' )
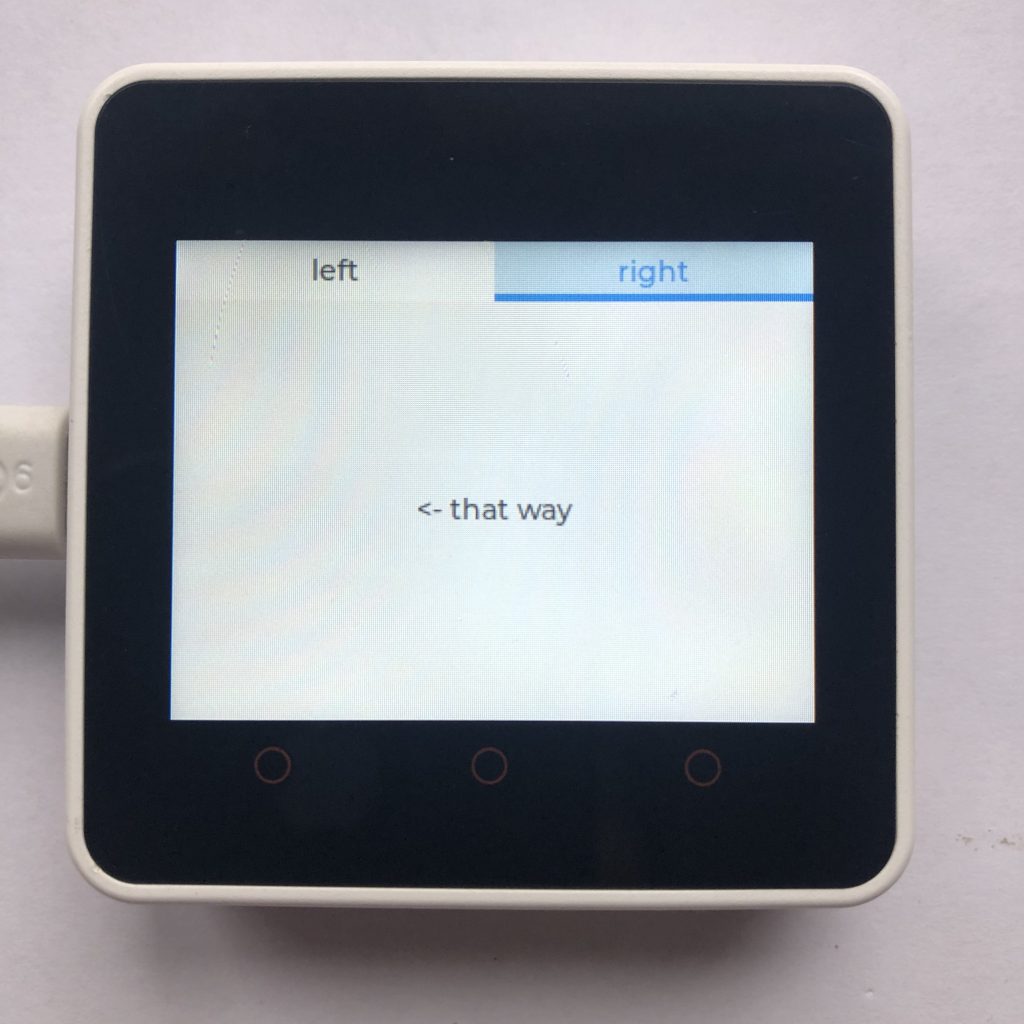
Having Code Set The Current Tab
Besides pressing the tab buttons or swiping, you can change the current open tab in code with the .set_act function.
import lvgl
tabview = lvgl.tabview( lvgl.scr_act(), lvgl.DIR.TOP, 30 )
tabview.set_size( 320, 240 )
tabview.center()
firstTab = tabview.add_tab( 'left' )
firstLabel = lvgl.label( firstTab )
firstLabel.set_text( 'that way ->' )
firstLabel.center()
secondTab = tabview.add_tab( 'right' )
secondLabel = lvgl.label( secondTab )
secondLabel.set_text( '<- that way' )
secondLabel.center()
from time import sleep
while True:
tabview.set_act( 1, lvgl.ANIM.ON )
sleep( 1 )
tabview.set_act( 0, lvgl.ANIM.OFF )
sleep( 1 )
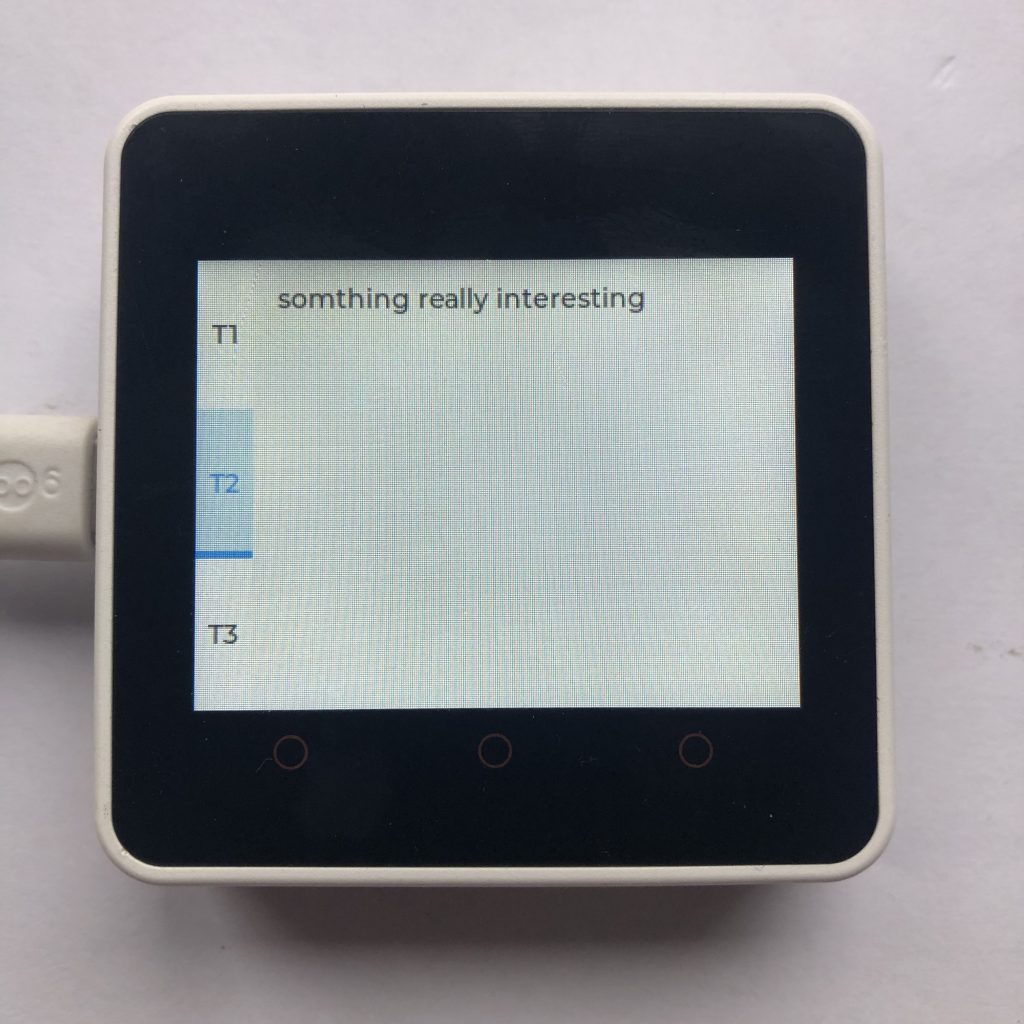
Changing The Tab Button’s Position
The tab buttons don’t always have to be on the top. You can put them all over the place.
import lvgl
tabview = lvgl.tabview( lvgl.scr_act(), lvgl.DIR.LEFT, 30 )
tabview.set_size( 320, 240 )
tabview.center()
firstTab = tabview.add_tab( 'T1' )
firstLabel = lvgl.label( firstTab )
firstLabel.set_text( 'hello this is the first tab' )
firstLabel.center()
secondTab = tabview.add_tab( 'T2' )
secondLabel = lvgl.label( secondTab )
secondLabel.set_text( 'somthing really interesting' )
thirdTab = tabview.add_tab( 'T3' )
thirdLabel = lvgl.label( thirdTab )
thirdLabel.set_text( 'something else' )
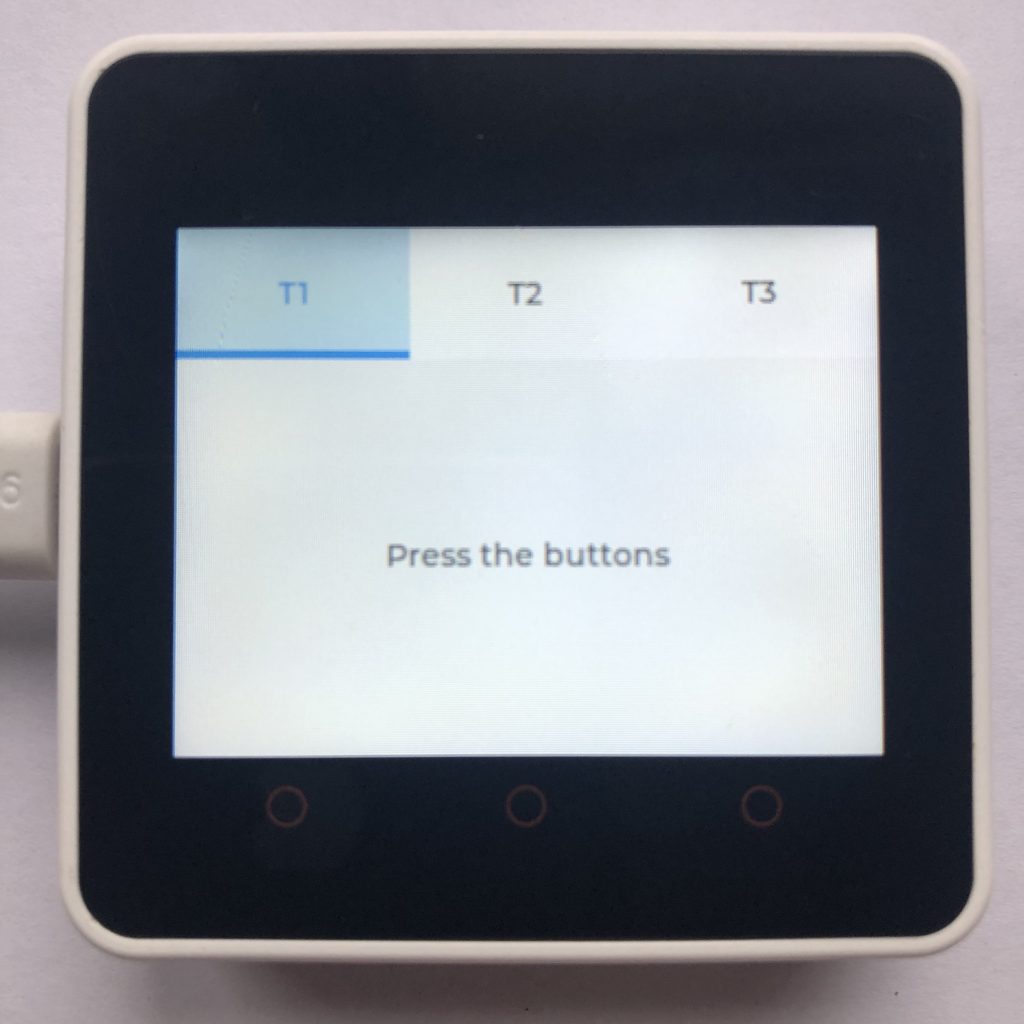
LVGL Tabview That Doesn’t Scroll
In this tabview, scrolling has been removed and it no longer plays an animation when changing screens.
import lvgl
def handler(data):
anim = lvgl.anim_t.__cast__( data.get_param() )
if anim:
anim.time = 0
tabview = lvgl.tabview( lvgl.scr_act(), lvgl.DIR.TOP, 60 )
tabview.set_size( 320, 240 )
tabview.center()
cont = tabview.get_content()
cont.add_event_cb( handler, lvgl.EVENT.SCROLL_BEGIN, None )
cont.clear_flag( lvgl.obj.FLAG.SCROLLABLE )
firstTab = tabview.add_tab( 'T1' )
firstLabel = lvgl.label( firstTab )
firstLabel.set_text( 'Press the buttons' )
firstLabel.center()
secondTab = tabview.add_tab( 'T2' )
secondLabel = lvgl.label( secondTab )
secondLabel.set_text( 'swipping does not work' )
thirdTab = tabview.add_tab( 'T3' )
thirdLabel = lvgl.label( thirdTab )
thirdLabel.set_text( 'tab3 says nothing' )
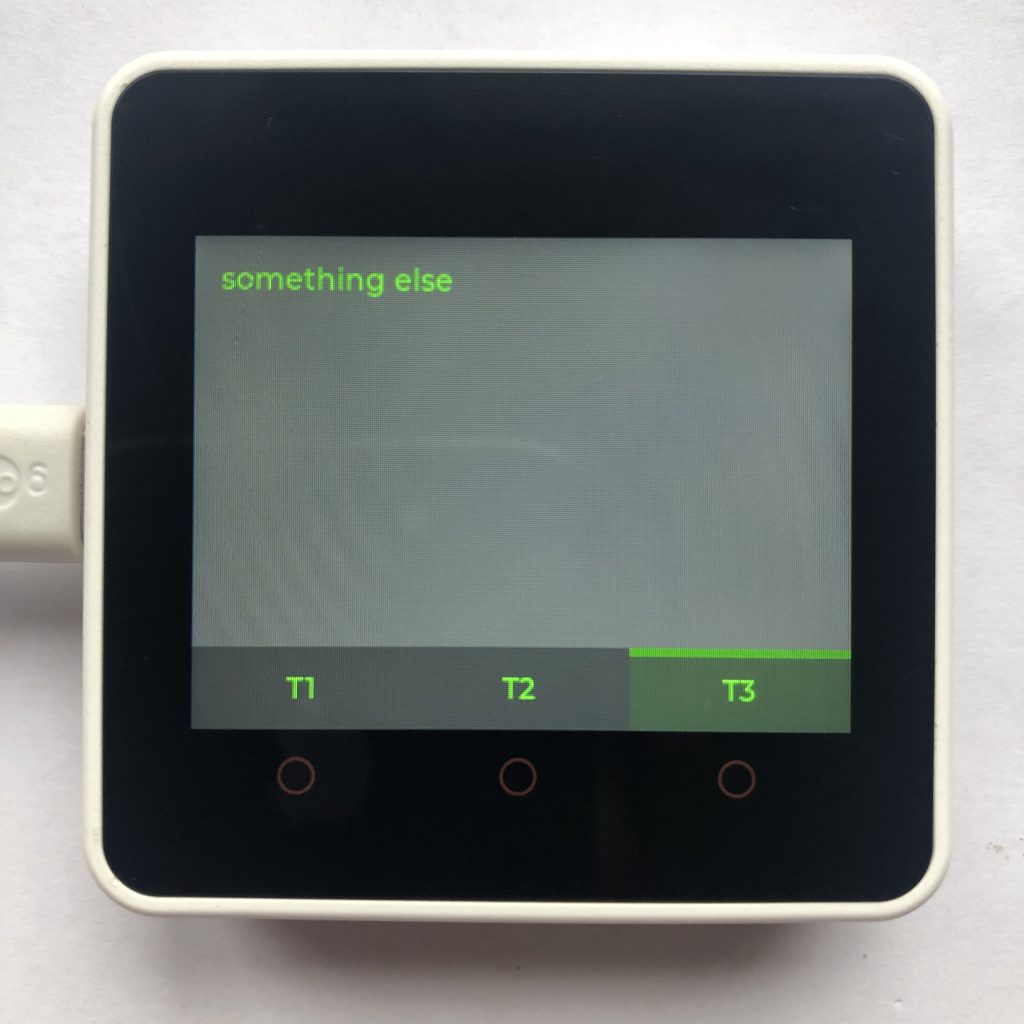
Styling Tabviews
This example just shows how you can style tabviews and their different parts.
import lvgl
tabview = lvgl.tabview( lvgl.scr_act() ,lvgl.DIR.BOTTOM, 40 )
tabview.set_size( 320, 240 )
tabview.center()
firstTab = tabview.add_tab( 'T1' )
firstLabel = lvgl.label( firstTab )
firstLabel.set_text( 'hello this is the first tab' )
firstLabel.center()
secondTab = tabview.add_tab( 'T2' )
secondLabel = lvgl.label( secondTab )
secondLabel.set_text( 'somthing really interesting' )
thirdTab = tabview.add_tab( 'T3' )
thirdLabel = lvgl.label( thirdTab )
thirdLabel.set_text( 'something else' )
cont = tabview.get_content()
tabview.set_style_bg_color( lvgl.color_hex(0x404040), 0 )
cont.set_style_text_color( lvgl.color_hex(0x00FF00), 0 )
btn = tabview.get_tab_btns()
btn.set_style_bg_color( lvgl.color_hex(0x202020), 0)
btn.set_style_text_color( lvgl.color_hex(0x00ff00), 0)
btn.set_style_border_side( lvgl.BORDER_SIDE.TOP, lvgl.PART.ITEMS | lvgl.STATE.CHECKED )
btn.set_style_border_color( lvgl.color_hex(0x008000), lvgl.PART.ITEMS | lvgl.STATE.CHECKED )
btn.set_style_bg_color( lvgl.color_hex(0x006800), lvgl.PART.ITEMS | lvgl.STATE.CHECKED )
btn.set_style_text_color( lvgl.color_hex(0x00FF00), lvgl.PART.ITEMS | lvgl.STATE.CHECKED )
Conclusion of Tabview
Tabviews are great at displaying a lot of information in a small area of your screen. The examples on this page should have giving you a good start on how to create them.
If you liked tabviews you might like micropython lvgl textarea examples.