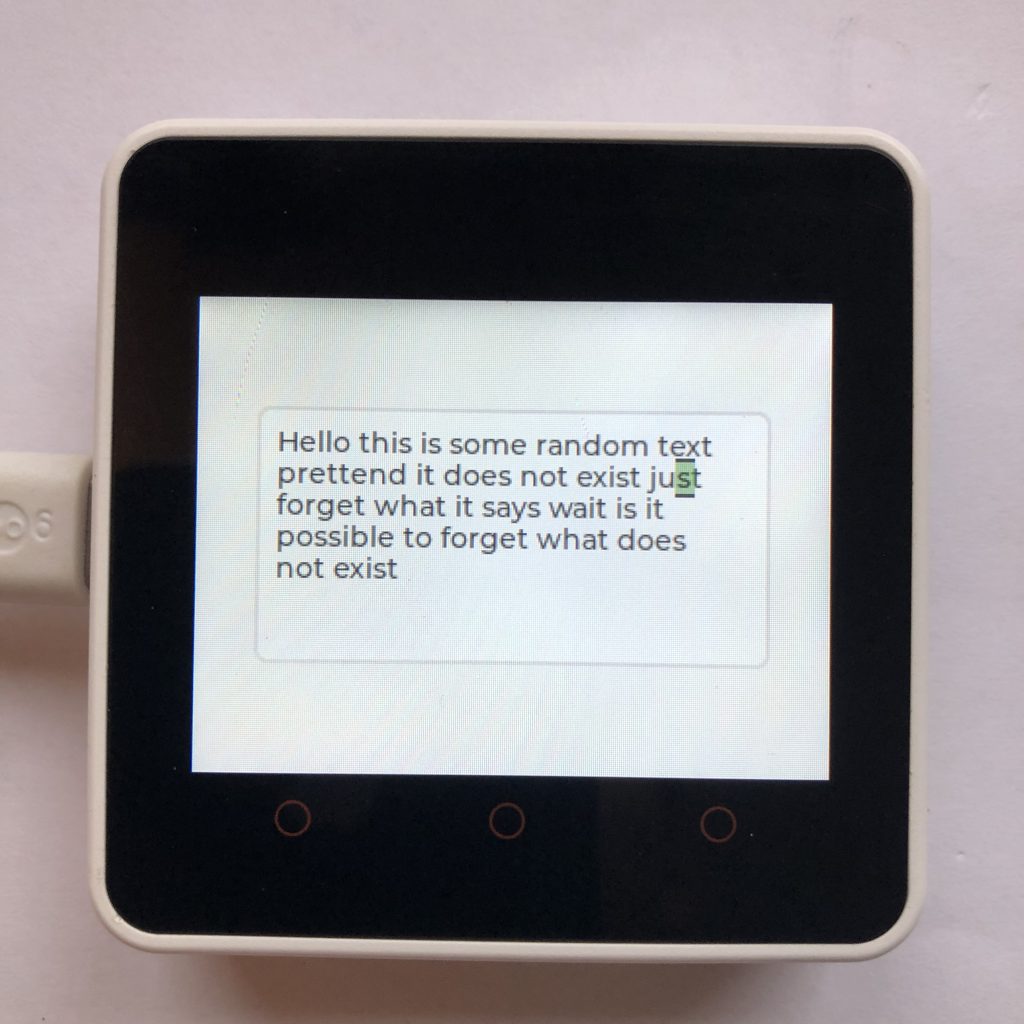
What Are Textareas?
Textareas are intended for places where you need to display text that changes often. They are usually used with keyboards or some input device. This post has examples on creating textareas, styling cursors, and creating password textareas.
How to use these examples
To get these examples to work you will need to add some screen code to the beginning of each example. If you want more information try How to get a LVGL Micropython screen to work.
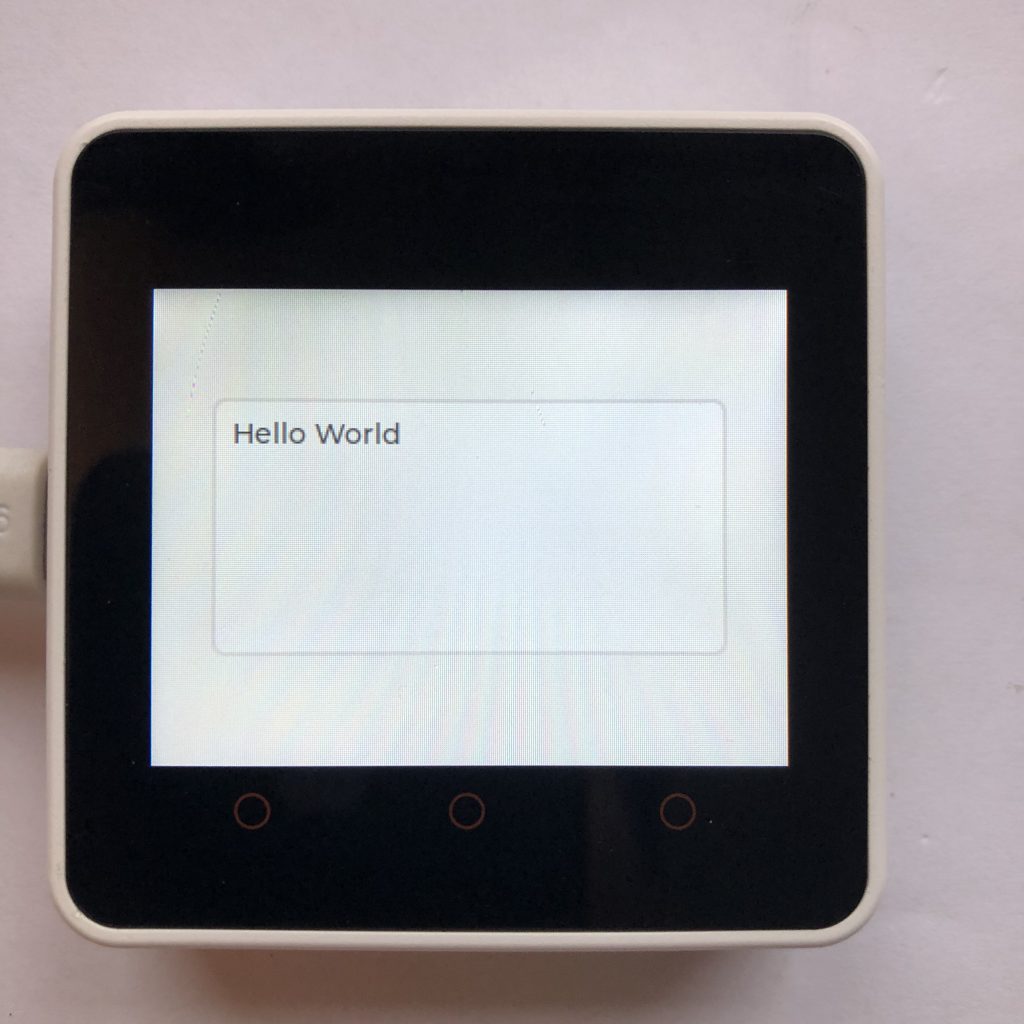
The Basic Example
This code creates a textarea and shows how adding text works.
import lvgl
from time import sleep
textarea = lvgl.textarea( lvgl.scr_act() )
textarea.set_text( 'Hello ' )
textarea.center()
sleep( 1 )
textarea.add_text( 'W' )
sleep( 0.5 )
textarea.add_text( 'o' )
sleep( 0.5 )
textarea.add_text( 'rld' )
sleep( 0.5 )
textarea.set_cursor_pos( 0 )
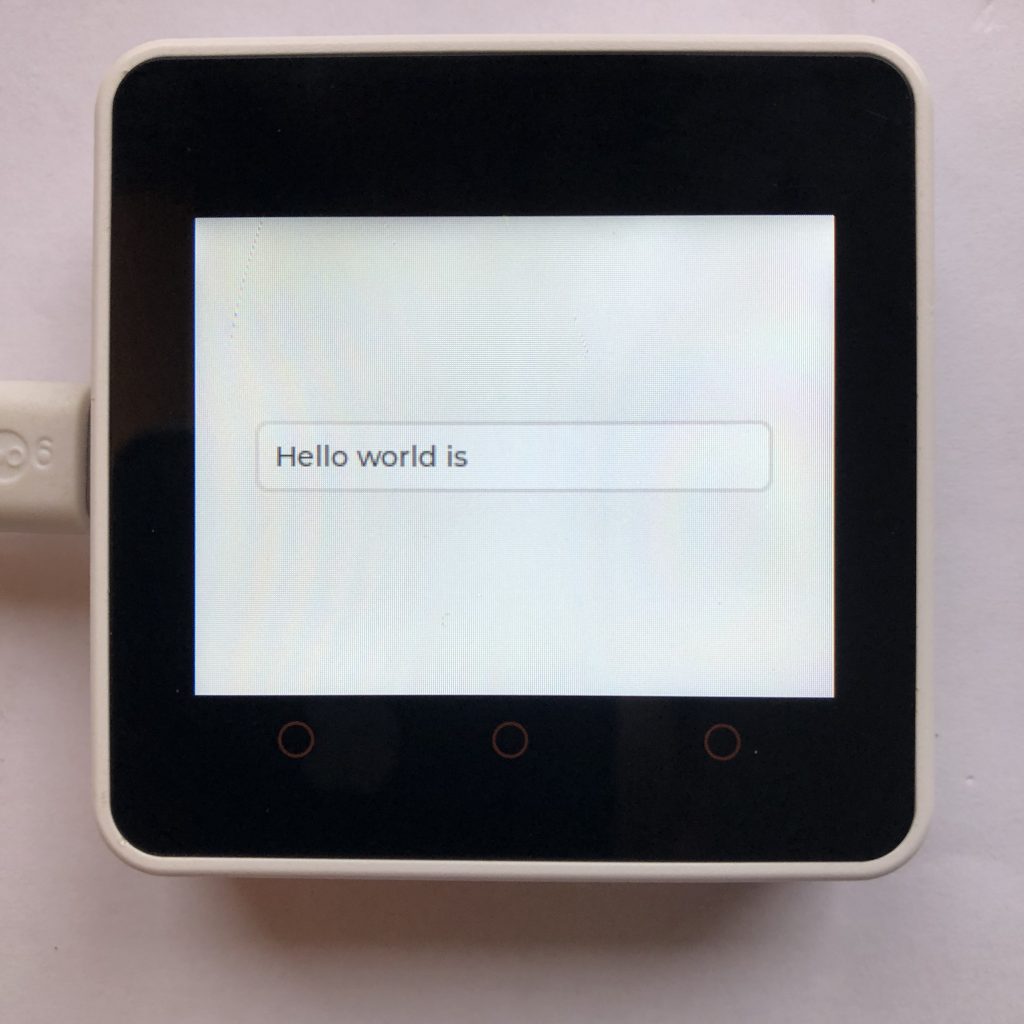
Maximum Characters and One Line Mode
LVGL allows you to set the textarea to only use one line and to set the maximum number of characters that are allowed in the textarea.
import lvgl
textarea = lvgl.textarea( lvgl.scr_act() )
textarea.set_max_length( 15 )
textarea.center()
textarea.set_text( 'Hello world is to long' )
textarea.set_one_line( True )
textarea.set_cursor_click_pos( True )
#does not work with touch input might with mouse
textarea.set_text_selection( True )
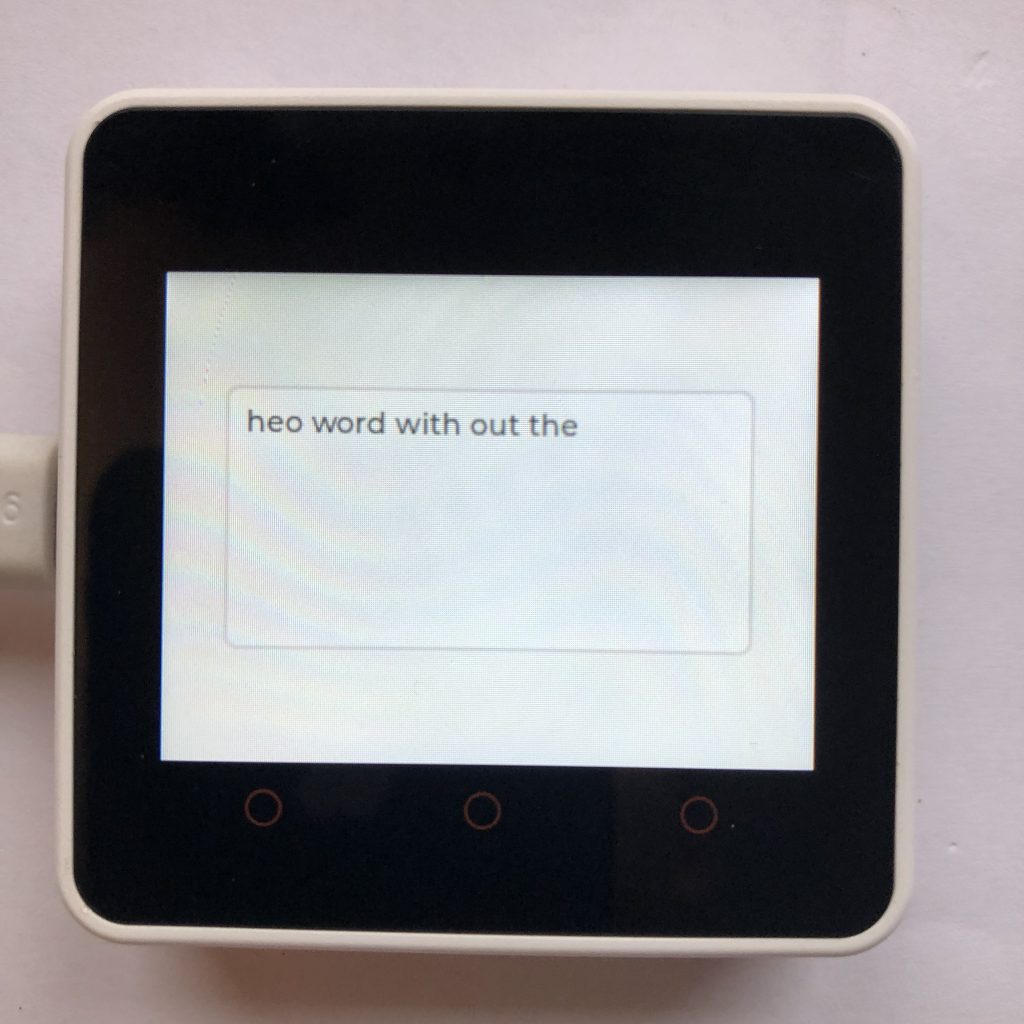
Accepted Characters
Textareas can filter out any character they don’t want and not put them on the screen. This code creates a textarea that does not display the letter L.
import lvgl
textarea = lvgl.textarea( lvgl.scr_act() )
#l is the only letter not accepted
textarea.set_accepted_chars( 'abcdefghijk mnopqrstuvwxyz' )
textarea.set_text( 'hello world with out the l' )
textarea.center()
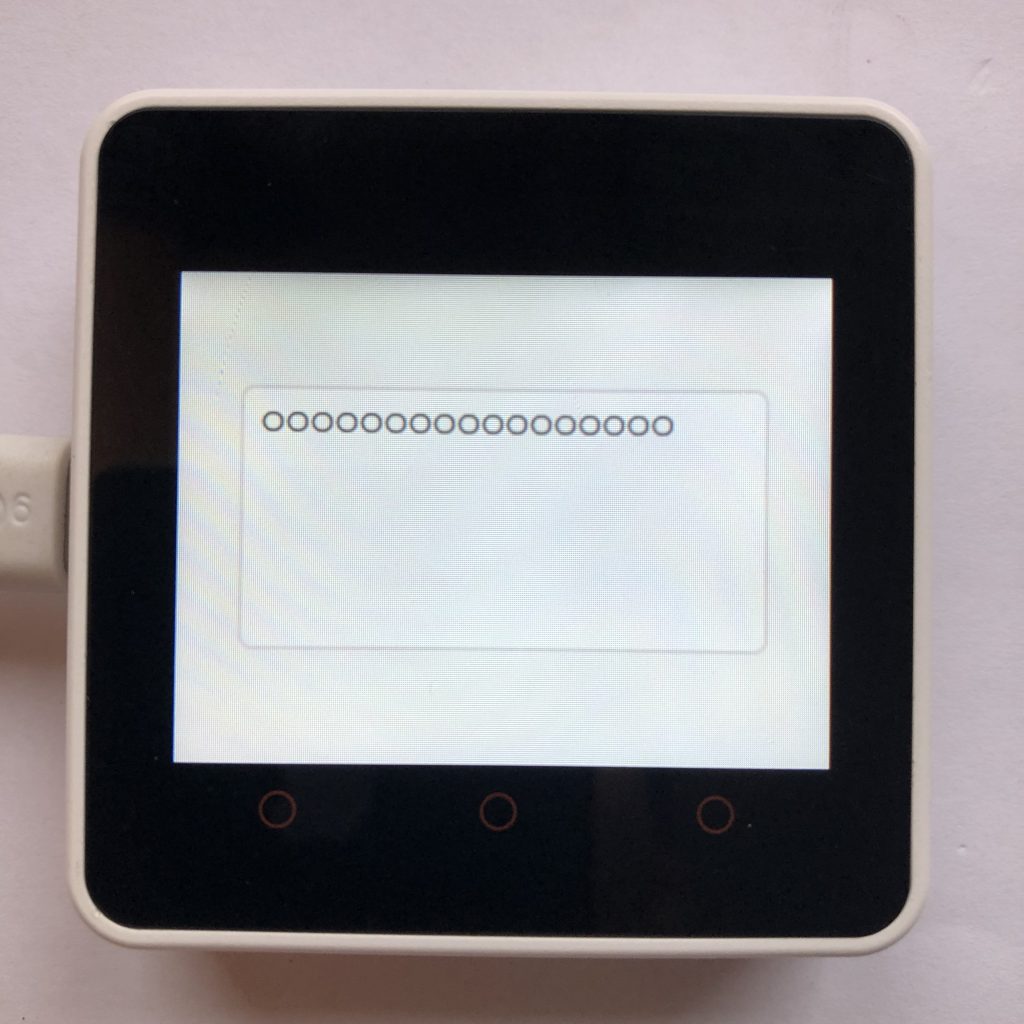
Passwords and Textareas
One of the features of micropython textareas is that they can be used for passwords. This code show the most import features of password textareas.
import lvgl
from time import sleep
textarea = lvgl.textarea( lvgl.scr_act() )
textarea.set_text( 'super secret text' )
textarea.center()
textarea.set_password_mode( True )
sleep( 2.5 )
#the bullet should be only one character long
textarea.set_password_bullet( 'O' )
#update text area to see new bullet
textarea.add_text( '' )
print( textarea.get_text() )
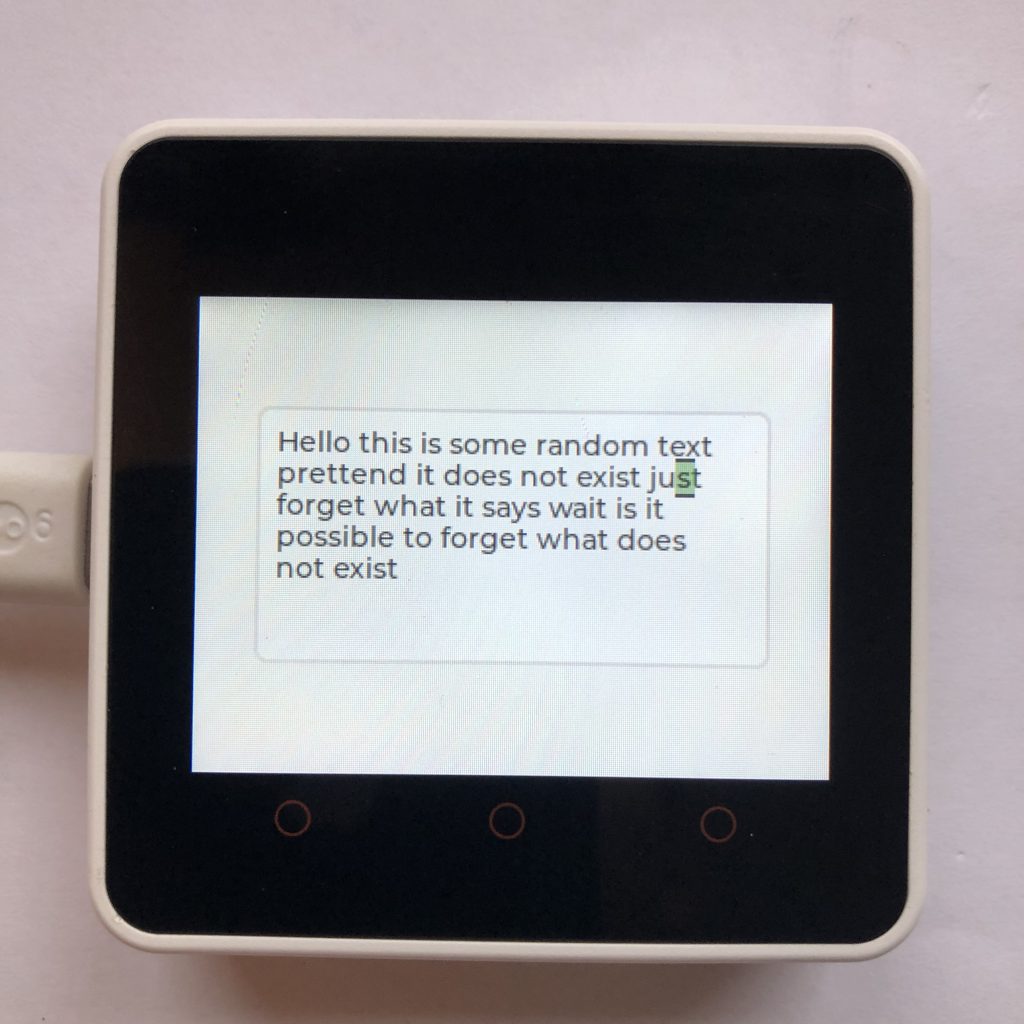
The Cursor
Micropython lvgl allows you to create and style cursors. This code will randomly move the cursor around the screen and add some styles to it.
import lvgl
from time import sleep
textarea = lvgl.textarea( lvgl.scr_act() )
textarea.set_text( 'Hello this is some random text prettend it does not exist just forget what it says wait is it possible to forget what does not exist' )
textarea.center()
cursor_style = lvgl.style_t()
cursor_style.set_border_side( lvgl.BORDER_SIDE.TOP | lvgl.BORDER_SIDE.BOTTOM )
cursor_style.set_bg_color( lvgl.color_hex( 0x009A00 ))
cursor_style.set_bg_opa( 100 )
textarea.add_style( cursor_style,lvgl.PART.CURSOR | lvgl.STATE.FOCUSED )
textarea.add_state( lvgl.STATE.FOCUSED )
while True:
textarea.set_cursor_pos( lvgl.rand( 0, 70 ))
sleep( 0.5 )
textarea.cursor_right()
sleep( 0.5 )
textarea.cursor_down()
sleep( 0.5 )
textarea.cursor_left()
sleep( 0.5 )
textarea.cursor_up()
sleep( 0.5 )
Where to go next
Textareas are nice, but they are not super useful with out an input. A great input to use is keyboards.
You can wire up a custom keyboard with physical buttons, but if you want a simpler (but more annoying) keyboard, you could try micropython lvgl keyboard examples. The last example has code for connecting keyboards to textareas.